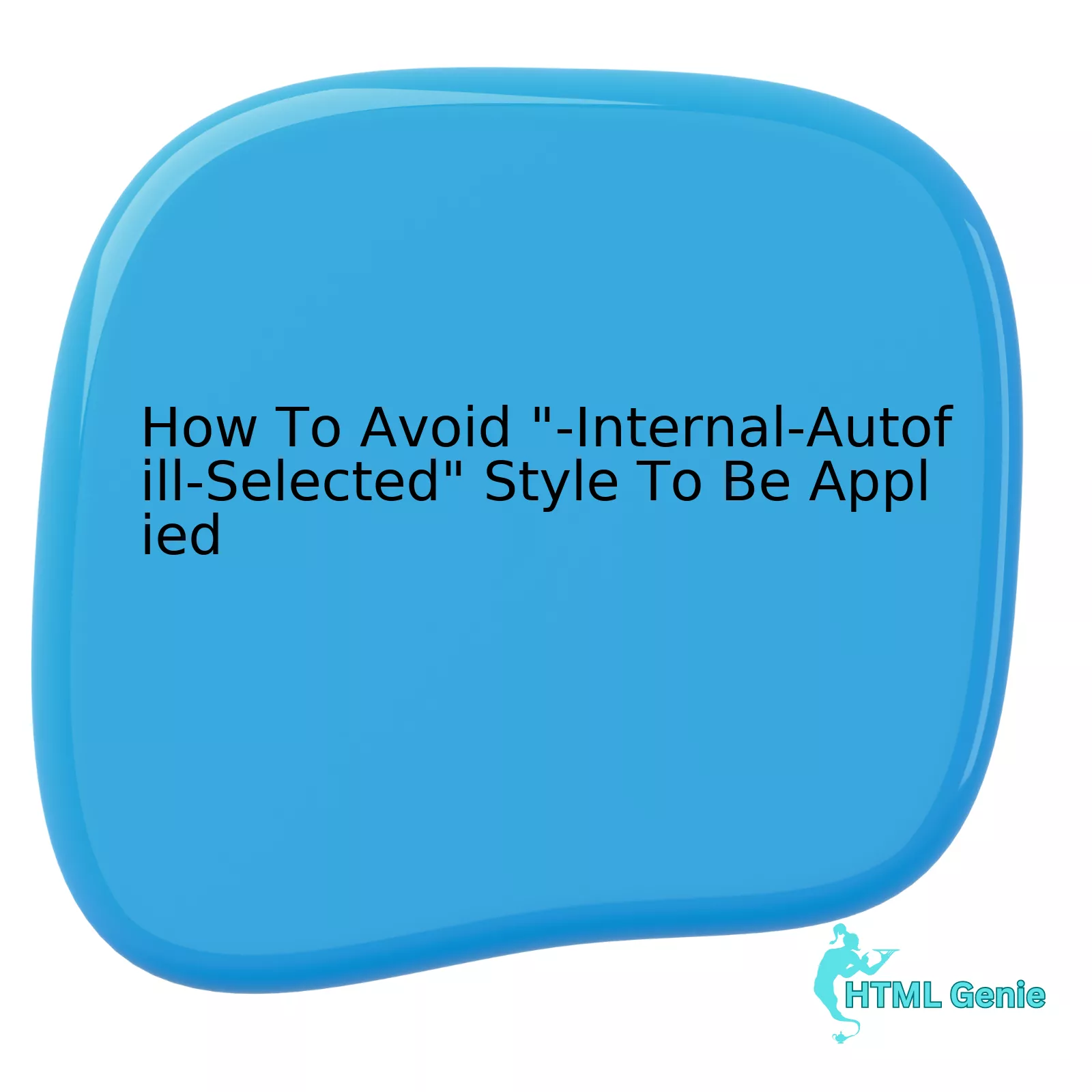
It’s handy to know you can’t directly override this browser-specific pseudo class in your stylesheets. However, there are innovative ways to address it, one of them being employing of shadow DOM, even though this approach would be somewhat complex. Yet others may prefer risk-free solutions and opt for manually disabling autofill suggestions.
Unfortunately, neither option provides error-proof results across all devices or all browsers. Thus, consideration of such limitations is crucial in decision-making.
Let’s illustrate circumstances with a hypothetical markup:
html
First Name |
In the presented block, there is a text input field enclosed in a table data cell where the user enters his first name. Applying the `autocomplete=”off”` attribute within the input tag attempts to prevent the browser from suggesting autofill options based on previously entered data.
While HTML5 specifications suggest this method, some modern browsers have started ignoring this attribute for privacy reasons, i.e., not exposing raw user inputs in form fields. Therefore, another possible workaround involves setting the autocomplete value to something random or unrecognizable:
html
Despite these suggestions, there isn’t yet a universal solution. Appropriate methods vary significantly based on individual project parameters, such as targeted browsers or platforms, and developer preferences.
We advise developers to stay updated as web standards evolve, since optimal solutions could change over time. A reliable source for such information is [MDN documentation](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/autocomplete).
Lastly, remember what Bill Gates once said: “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency. The second is that automation applied to an inefficient operation will magnify the inefficiency.” This statement applies to our topic as well; focusing on efficiency, rather than rely solely on automation, will more often yield better results.
Understanding the “-Internal-Autofill-Selected” Style in HTML
Understanding the “-internal-autofill-selected” style in HTML provides us with an apt understanding into how certain components of CSS work. The “-internal-autofill-selected” style, a pseudo-element in CSS, is automatically implemented by browsers like Google Chrome when the form fields get auto-filled by the browser’s auto-fill functionality, indicating a user interaction.
This pseudo-class styling:
input:-webkit-autofill, input:-webkit-autofill:hover, input:-webkit-autofill:focus { color: #262626 !important; }
gives you control over the colors of the text and background when the auto-fill happens. However, there’s been significant discussion whether it yields any tangible SEO benefits or detriments. Furthermore, some developers might not want this specific styling to be applied but are unsure of how to avoid this.
So, if your primary requirement is to prevent the “-internal-autofill-selected” style from being applied, there are a number of potential solutions you can utilize.
Firstly, you could consider disabling the autofill feature altogether, however, this route is generally not recommended as it significantly hampers user convenience. Here’s how the code would look:
But, if the goal is solely to avoid the autofill-specific styling, CSS’s “!important” declaration is widely used. This overrides any other declarations, making it invaluable while trying to avoid the “-internal-autofill-selected” style from being applied.
Here is an example:
input:-webkit-autofill, input:-webkit-autofill:hover, input:-webkit-autofill:focus { background: #FFFFFF !important; color: #333333 !important; }
Naturally, “#FFFFFF” (white) and “#333333” (dark grey) should be replaced by the intended color codes. An exhaustive list of hex color codes is readily available online.
Another approach revolves around using JavaScript events such as “focus” and “blur” to manage the styles on autofill manually. This method is complex and may conflict with good coding practices as per The Zen of Python by Tim Peters, which emphasizes “Simple is better than complex.”
While handling the “-internal-autofill-selected” CSS pseudo-element mandates acute sensitivity towards user convenience, it invariably opens up avenues for increased customization according to the specificity of needs. It also exemplifies the malleability of HTML & CSS when adeptly manipulated, echoing the sentiments of renowned technologist Matt Mullenweg: “Technology is best when it brings people together.”
Tactics to Prevent the Application of “-Internal-Autofill-Selected” Style
Avoiding the application of the `-internal-autofill-selected` style could be achieved by employing strategies that revolve around the structure and design of HTML code.
The attribute `-internal-autofill-selected`, like various other browser-specific functions, uses heuristics to determine when it should modify an input element. The heuristics rely immensely on DOM (Document Object Model) signals to understand if autofill should be triggered. By subtly modifying these cues, one can prevent the triggering of autofill, thereby preventing the application of the `-internal-autofill-selected` style.
Insights for achieving this include:
– Allocation of mutable values to `autocomplete` attributes: Each time a form is filled, change the `autocomplete` attribute of the form fields to denote different identities, thus keeping the system guessing.
<div> <label for="fname">First name:</label> <input type="text" id="fname" name="fname" autocomplete="new-password"> </div>
<div> <label for="lname">Last name:</label> <input type="text" id="lname" name="lname" autocomplete="new-password"> </div>
Tempering with form labeling: As `-internal-autofill-selected` relies on form labels for hinting, use of generic IDs without semantic meaning can be performed. However, this approach hinders accessibility and SEO.
Retracting from using common field names: Names such as ’email’, ‘phone’ generally trigger autofill. To bypass this, using unique but understandable names can aid in avoiding autofill.
Just as Bill Gates rightly states “Measuring programming progress by lines of code is like measuring aircraft building progress by weight”, it is essential to understand that it’s not merely about writing tons of code but about crafting effective and efficient code that holds the power to conquer the desired functionality while enhancing user experience.
However, a word of caution is numerous tactics, might have possible implications on your site’s accessibility, usability, and SEO as some techniques may lead to difficulty for assistive technologies to access page content. Therefore, carefully weigh the benefits against potential costs before implementing.
Incorporating these tactics into your HTML interfaces will most likely result in an undetectable approach to avoiding the application of `-internal-autofill-selected`. Notwithstanding, it’s good practice to perform routine checks to ensure that the implementation remains effective and does not influence the accessibility and usability negatively.
For further information, refer to Mozilla Developer Network’s guide on [Autofill](https://developer.mozilla.org/en-US/docs/Web/HTML/Attributes/autocomplete).
Implication of the “-Internal-Autofill-Selected” Style on User Experience
The “-internal-autofill-selected” style implication holds substantial prominence when it comes to the user experience on a website. This internal styling is employed by browsers such as Google Chrome or Safari, used to highlight an autofilled input field in a form. It enhances usability by visually delineating fields that have been auto-populated, providing immediate feedback to users that their saved data has been successfully inputted.
User Experience Benefits |
---|
Another dimension to consider, however, is control over the application of “-internal-autofill-selected” style. Designers often prefer maintaining consistency across different browsers and this can become challenging with browser-specific styles. Avoiding the application of this automatically assigned style could also be desirable for design or aesthetic reasons.
So, how do we avert “-internal-autofill-selected” style from being applied? There’s no direct way to prevent it, but you can use methods to override the default behaviour. Unfortunately, browsers don’t provide a lot of options to customize the autofill experience, so you have to improvise.
Here’s a simple illustrative example where a bit of CSS pseudoselector magic can help:
/* Your standard input field style */ input { background-color: white; color: black; } /* Style to override autofilled field appearance */ input:-webkit-autofill { -webkit-text-fill-color: black !important; -webkit-box-shadow: 0 0 0px 1000px white inset !important; } input:-webkit-autofill:focus { -webkit-text-fill-color: black !important; -webkit-box-shadow: 0 0 0px 1000px white inset !important; }
In this example, the styling of your choice is applied to the autofill-selected text fields, which supersedes the default browser style. You’re creating a box shadow inside the input box (inset), large enough to cover the entire input field, then setting its color to whatever you want.
It should be noted here, this method has limitations because it relies on browser-specific pseudoselectors and could affect accessibility due to changes in contrast. Furthermore, UX Designer Chris Coyier urges, “We need to remember the reasons why our user’s needs come before our own desires or habits as designers.”
Finally, while there may be exceptions, any effort to manipulate browser-style is best avoided unless absolutely necessary for maintaining overall site style and without tampering with user-friendly aspects like legibility and clarity.
Actionable Steps Towards Mastering Selection Styles in Web Development
Mastering styles and avoiding certain automatic features in web development, like the “-internal-autofill-selected” style application, is achieved by following some key steps. What needs to be understood, first and foremost, is that an HTML form field, on focus and filling, can frequently trigger certain user agent stylesheets to enhance the UX. This might bring about the introduction of a style with an -internal-autofill-selected attribute.
Below are some functional steps to circumvent this:
Begin With Browser Preferences
Different WEB browsers have different in-built styles. Making changes to these will invariably alter how your HTML syntax appears and how it interacts with styling. Disabling the autofill option in browser settings would easily avoid the -internal-autofill-selected’ style. It also helps to understand the underlying CSS construction.
Understanding and Manipulating User Agent Stylesheets
Each browser carries its styles (user agent stylesheets). If you intend to prevent the “-internal-autofill-selected” style from applying, get familiar with manipulating these stylesheets. Familiarity with user agent stylesheets helps in overriding or neutralizing any unwanted styling effect.
Utilize Specific Selectors
Usage of specific selectors allows for minute control of elements on a web page. More discerning commands such as “:focus”, “:active”, or “:hover” can control how a form behaves when a user engages with it.
Overriding Autofill Styles
One method to override the autofill styles is by using
!important
rule in your CSS. Though this isn’t recommended, it could be a necessary evil in some circumstances.
Ensure High-Quality Coding Practice
Always practice high-quality code writing since effective coding generally evades brushes with issues like autofill-selected styles. Whilst coding, ensure the usage of proper semantics, layouts should be structured, and use well-labelled classes and IDs.
Bill Gates once said, “Measuring programming progress by lines of code is like measuring aircraft building progress by weight”. In mastering styles, there’s no short-cut or quick fix. The journey towards mastery involves proactively learning, experimenting, testing, and understanding the nuances of the code.
Remember Steve Jobs’ quote, “Design is not just what it looks like and feels like. Design is how it works.” Mastering web designs and style implementation is all about making the site work smoothly, producing a great user experience.
For more insights, W3Schools (source) offers beneficial material on HTML and CSS styles. MDN Web Docs (source) also provides valuable information.An important aspect to consider when developing HTML or working on any web-related project is handling autofill-related styles. The “-internal-autofill-selected” style, applied automatically by browsers like Chrome, changes the color of input fields once their values are set via autofill, which might not align with the desired aesthetic of your webpage. However, there exists no direct method to override this browser-applied CSS in a usual sense.
But a workaround can be implemented to get around it and this involves blurring out the input field immediately after it gets autofocused.
html
In the above example, an event listener is added which fires on the ‘focus’ event, then the ‘blur’ function is called inside a ‘setTimeout’ to blur out the email input box as soon it gets autofocused. Since there’s a delay in applying the “-internal-autofill-selected” style, the quick blur counteracts it and prevents the style from being applied.
As a reminder, HTML standards and best practices should always be followed to ensure optimum website performance. According to Smashing Magazine, a renowned resource for web design tips and tricks, “websites that adhere to these practices provide a much better user experience.”
“Everybody in this country should learn to program a computer, because it teaches you how to think.”- Steve Jobs. Coding is indeed a process that constantly challenges us to find effective solutions to problems and improve our creativity and analytical skills. It’s always vital to remember the importance of maintaining clean, efficient, and user-friendly code, even when faced with intricate tasks like dealing with autofill styles.