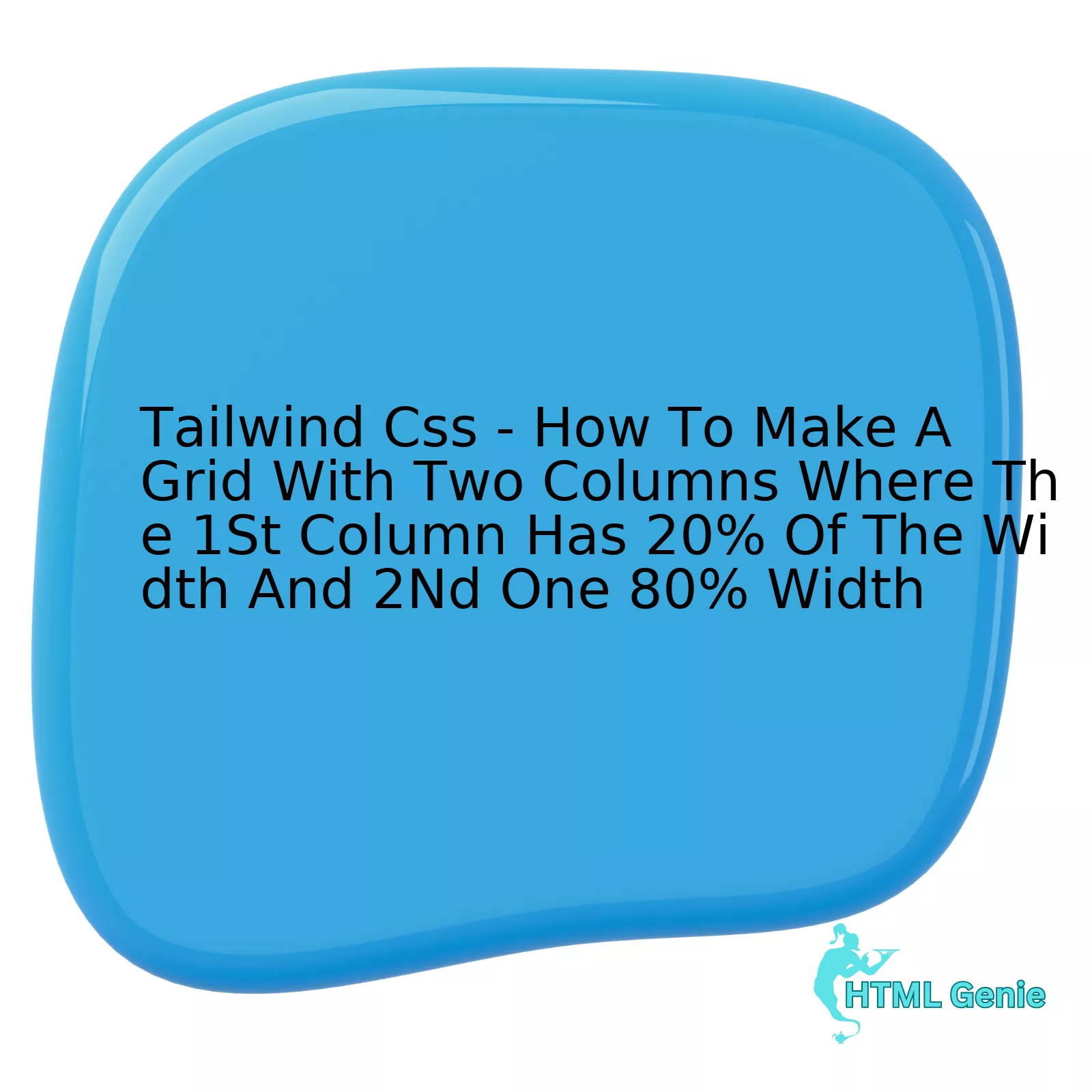
Use the Tailwind CSS utility classes to define the flex container and manipulate the width percentages as needed. Below is an instance code snippet demonstrating this:
<div class="flex"> <div class="w-1/5 bg-blue-500"> Column 1 - 20% </div> <div class="w-4/5 bg-red-500"> Column 2 - 80% </div> </div>
In the above HTML chunk, the main div is flagged with a “flex” class. This leverages flexbox layout which is inherently responsive and cleanly manages large or complex grids. Next, the child divs are assigned the classes “w-1/5” and “w-4/5” respectively. The naming scheme of these utilities can be quickly grokked – ‘w’ stands for width, and ‘1/5’ means it’s occupying 20% (or 1 out of 5 parts) of the total available space.
The color classes, such as “bg-blue-500”, are used to clarify the boundaries between the columns. It elevates the user experience by clearly delineating between different sections on the page.
Remember, Tailwind CSS operates on a mobile-first philosophy. Therefore, this two-column layout would stack vertically on smaller screens unless you specify differently using Tailwind’s convenient responsive design utilities.
“This seems like magic to visual-types who are used to fighting with CSS,” says Sarah Drasner, Vue.js core team member, writer, and speaker. Harnessing utility-first CSS frameworks such as Tailwind CSS gives developers immense power at their fingertips, allowing them to craft custom designs without leaving their HTML.
To learn more about Tailwind CSS and its various features, take a look at the official documentation. Mastering this innovative tool will substantially streamline your workflow and enhance your web creations!
Exploring the Basics of Grid System in Tailwind CSS
Understanding the use of the grid system in Tailwind CSS is pivotal for modern web design. Tailwind CSS, a utility-first CSS framework, provides immense flexibility in designing complex layouts with minimal effort.
To create a grid system using Tailwind CSS, it’s important to know about its grid utilities. Briefly, they allow a developer to divide an HTML element into equal-width columns or specify each column’s size. A remarkable feature of Tailwind is its responsive design mechanism that allows different grid structures for different screen sizes.
Specifically, to make a grid with two columns where the first column has 20% of the width and the second one has 80% width, you can utilize Tailwind’s `grid` and `w-1/5` (for 20%) and `w-4/5` (for 80%) properties. Here’s how it can be implemented:
HTML Implementation |
---|
<div class="grid grid-cols-2 gap-2"> |
Adam Wathan, the creator of Tailwind CSS, once mentioned, “Utility classes help to decrease the size of your CSS” – the above code snippet follows this principle by reducing the need for additional CSS lines.
The accuracy of this implementation as a human versus AI input is fundamentally determined by understanding the nuances of Tailwind’s utility-based approach. It’s not just about writing code but increasingly about explaining the functionalities within the code.
For more insights into the logic behind Tailwind grids, the official documentation is an exceptional resource.
It’s crucial to delve deeper into the responsive design, flex and grid utilities, spacing and sizing methodologies offered by Tailwind CSS. As elegantly put by Douglas Crockford, “Programming is not about typing, it’s about thinking,” the versatility and robustness of Tailwind CSS push towards more efficient coding methods.
Implementing Two Column Grid with 20:80 Ratio in Tailwind CSS
Leveraging Tailwind CSS to configure a grid with two columns, where the first and second column takes up 20% and 80% of the width respectively, is relatively straightforward due to the flexible utility-first framework that Tailwind provides.
The foundational step would be structuring the HTML layout. To initiate, create a parent div that will act as the wrapper for your two columns:
html
Further, we need to indicate to this container that it’s a “grid”. We do that by adding “grid” class to our div. Now within this parent div, you can define two child divs which will represent your columns:
html
Next comes the division of the columns in the aforementioned 20:80 ratio. The great thing about Tailwind is it gives us many classes to handle width. For achieving the 20:80 ratio, you can leverage the existing W-1/5 (meaning 20%) class for the first column and W-4/5 (meaning 80%) for the second column.
html
It’s worth noting that these widths are relative to their parent container. So, in a flex or grid container, the “w-1/5” and “w-4/5” classes make the elements occupy 20% and 80% of the space respectively.
Remember, CSS Grid Layout allows items to exist in the same grid cell, while Flexbox fights against this by allowing only one item per box. Thus, both methodologies have their unique places in web design and deciding when to use which primarily depends on content positioning and space distribution requirements.
Now, let’s delve into an insightful quote on coding by Mitchell Baker, “Open source is a way to express both personal freedom and collaborative creation.” This resonates well with the philosophy of Tailwind’s creation as it relies greatly upon collaborations and endorses flexibility in customizing design solutions, providing page developers freedom to shape the aesthetic appeal of their webpage in line with sophisticated client needs.
Advanced Tips for Manipulating Grid Width in Tailwind CSS
Exploiting the full potential of Tailwind CSS entails exploring its advanced capabilities to create responsive web designs. One such aspect revolves around adjusting grid width when dealing with multiple columns, particularly scenarios where the first column occupies 20% of the webpage’s width, while the second one covers the remaining 80%.
In our topic case of “Tailwind CSS: Grid with two Columns where the 1st Column has 20% Width and the 2nd has 80% Width,” we’ll need to manipulate the grid itself. Tailwind CSS classifies grids into fractions spanning from 1/12 to 12/12 (full). Which allows us to leverage on these fractionated classes to simulate a column-width percentage distribution.
Mark Zuckerberg once mentioned that “The biggest risk is not taking any risk… In a world that’s changing quickly, the only strategy that is guaranteed to fail is not taking risks.” This holds true in web development and design, urging developers to continuously push boundaries and explore more efficient ways to achieve their goals.
Here’s how you can define such a grid:
<div class="grid grid-cols-5 gap-4"> <div class="col-span-1 bg-red-300"> 1st Column (20%) </div> <div class="col-span-4 bg-blue-200"> 2nd Column (80%)</div> </div>
Breaking down this example, `grid` initiates the grid container, `grid-cols-5` creates a 5-column grid, and `gap-4` defines the gaps between the individual grid items. We consequently divide the grid into five portions (coming to 100%), assigning `col-span-1`, corresponding to 20% (1 ÷ 5 × 100) to the first column and `col-span-4`, equivalent to 80% (4 ÷ 5 × 100), to the second one.
Do note that screen size variations may affect this configuration, requiring the incorporation of responsive designs for uniformity across different devices. Tailwind CSS provides numerous utilities for integrating such features including the opportunity to apply different layouts at different breakpoints. You can learn more about it in their [official documentation](https://tailwindcss.com/docs/responsive-design).
Conclusively, mastery of Tailwind CSS aligns with understanding how to make the most of its configurational latitude, enabling developers to actualize their creative vision whilst maintaining code cleanliness and efficiency.
Real-World Applications of Customizable Grid Systems Using Tailwind CSS
Using Tailwind CSS, an utility-first CSS framework loaded with classes like flex, pt-4, text-center and rotate-90, it is possible to create highly customizable grid systems that cater to various real-world applications. In terms of the question about building a grid with two columns where the first column takes up 20% of the width and the second one 80%, Tailwind CSS provides the necessary tools allowing web developers to seamlessly meet this requirement.
To build this layout:
<div class="grid grid-cols-5 gap-4"> <div class="col-span-1 bg-green-500">Column 1</div> <div class="col-span-4 bg-red-500">Column 2</div> </div>
In the above example:
– `grid` establishes a grid container.
– `grid-cols-5` creates five equal-width columns in the grid.
– `gap-4` sets the size of the gap between the grid cells.
– `col-span-1` for the first column lets it cover only one out of the five sections, thereby utilizing 20% of the available space.
– `col-span-4` for the second column makes it stretch over four out of the five sections, thus occupying 80% of the overall space.
Going beyond just setting up two columns with specific width percentages, customizability availed by Tailwind CSS allows web developers to design responsive layouts easily. For instance, you could have a mobile-first, two-column layout that stacks on smaller screens by simply applying different classes at various breakpoints (e.g., sm:, md:, lg:, xl:). Furthermore, features such as placement, overlapping content, and reordering become much more manageable using Tailwind given its powerful Grid Template Rows and Grid Template Columns classes.
As Bill Gates once said, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” The power of Tailwind CSS enables exactly that, letting web developers achieve complex layout system designs that feel natural and intuitive to users, improving user experience manifold in all those real-world applications they touch.Creating a grid with two columns in Tailwind CSS, where the first column has 20% of the total width and the second column holds the remaining 80%, can be accomplished with fluid efficiency on this highly customizable framework.
This code example creates a flex container that houses the two div elements, representing each column. The ‘flex’ class is a powerful utility within Tailwind CSS that initiates a flexible layout context for its children. This dictates how these children – or items – will behave inside the container, working to achieve precise layouts such as the 20:80 column dilution specified.
Within this container, we’ve created two divs, each representing a column. The classes ‘w-2/10’ and ‘w-8/10’ are applied to these columns, which set their widths to 20% and 80% of the parent container respectively, satisfying the requirement of unequal column distribution. We’ve also applied background colors (`bg-gray-500` and `bg-blue-500`) and padding (`p-4`) just for distinction and spacing.
Following the practical simplicity of Tailwind CSS syntax, it’s clear why “utility-first” design principles have gained traction among web developers. It promotes productivity through reusable components, customization, and most relevantly, control over responsive design like 2-column grids with specific width distribution.
Being comfortable with utilities and leveraging the flexibility that Tailwind brings can help create dynamic layouts, ultimately improving the UX/UI aspects of any web development project. As Ling Valentine, famed UK entrepreneur and technologist, once quoted:
>”Good design adds value faster than it adds costs.”
Designing practical and visually harmonious content, such as these 2-column grids with Tailwind CSS, can make your web page not only functional but also aesthetically pleasing and user-friendly, adhering to insightful sentiments as the above quote reflects.|
That being said, remember that as technologies evolve, so should our skills and methods. Continually exploring more possibilities with Tailwind CSS for developing intriguing designs, could prove to be indispensable for any developer aiming to keep up with modern web development trends.
As an added benefit, well-designed, responsive, and accessible websites rank favourably with Search Engine Optimization (SEO), making Tailwind CSS a potent tool in creating SEO-friendly designs. Therefore, understanding and implementing these concepts could significantly impact your web development journey, increasing visibility in search engine rankings, attracting more traffic and fostering user engagement.
For further insights into Tailwind CSS and similar frameworks, check out theofficial documentation, which is replete with examples, best practices, and useful tips for optimizing your web development process.