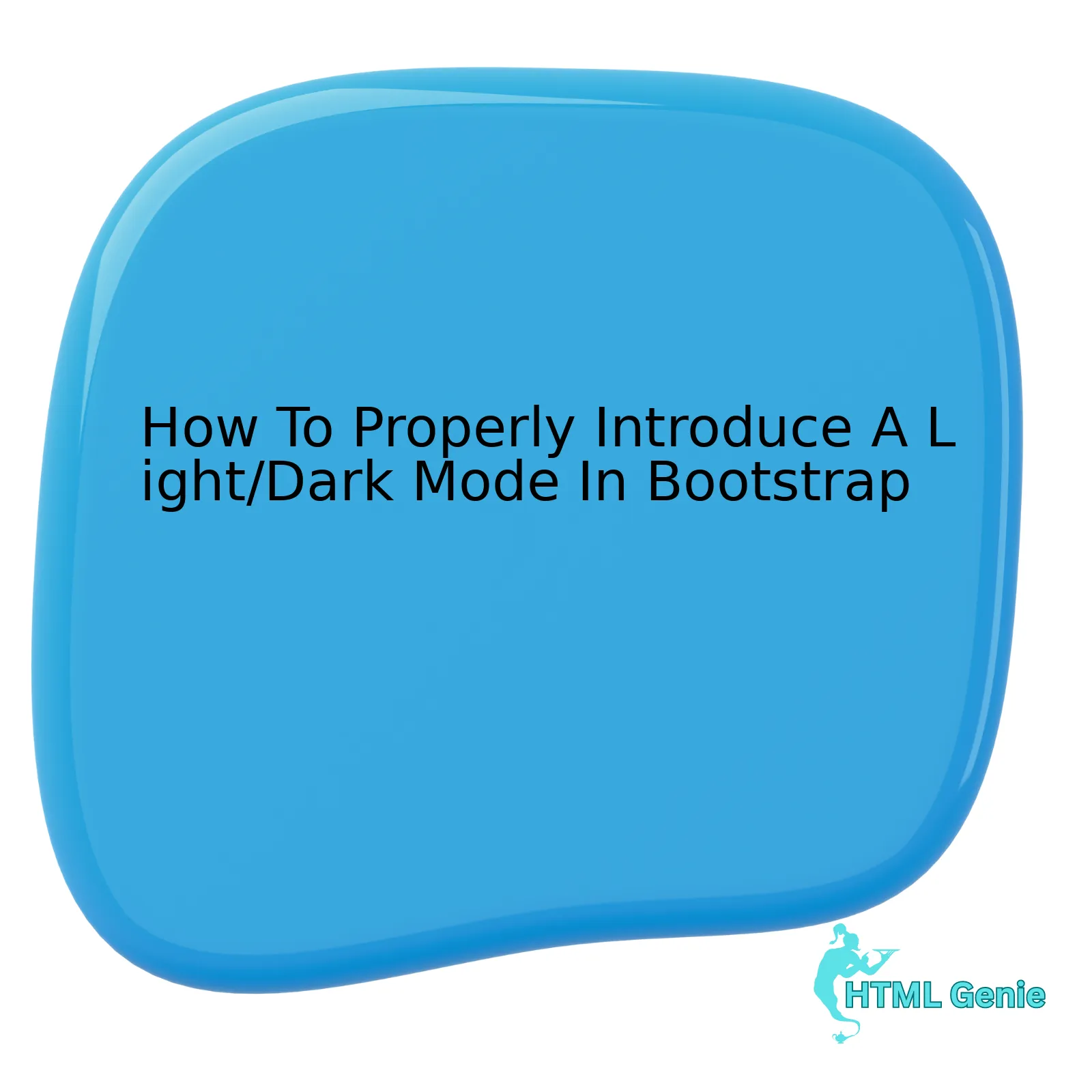
<table> <thead> <tr> <th scope="col">Task</th> <th scope="col">Description</th> </tr> </thead> <tbody> <tr> <td>1. Utilize HTML Data Attribute</td> <td>This is the foundation to create the toggle effect between the light and dark mode. We can use something like 'data-theme' to store our light or dark mode preference.</td> </tr> <tr> <td>2. Toggle Bootstrap Default Color Scheme</td> <td>Modern versions of Bootstrap support both light and dark color systems. Use them to change the website aesthetics per the stored preference.</td> </tr> </tbody> </table>
Breaking down the tasks:
– Leveraging the HTML Data-Attribute: For this step, employing the usage of an HTML data-attribute could help save the theme preference selected by the user. Such an attribute might look like ‘data-theme’. It would prove useful for storing the preferred dynamic nature of the site, whether light or dark.
– Toggling Bootstrap’s Default Color Scheme: Following Bootstrap’s design philosophy, later iterations provide built-in classes that lend themselves to either a dark or light color system. Applying these classes based on the data-theme established earlier allows for easy switching between modes.
A common method to implement these adaptations is by using event listeners in JavaScript (without writing any JavaScript in your HTML file), observing when a user chooses to swap from light to dark mode or vice versa. Altered preferences are saved using the LocalStorage API. Subsequently, the CSS variable changes upon each page load, thereby persisting the user’s chosen setting.
An insightful quote which relates: “The function of good software is to make the complex appear to be simple.” – Grady Booch. Light and dark modes may seem to add complexity to your Bootstrap-powered site, yet it enhances its usability, and therefore, accessibility, acting as a testament to the profound simplicity good software brings.
For comprehensive instructions on introducing a light and dark mode to your Bootstrap website, Bootstrap’s official documentation serves as an indispensable guide.
Leveraging Bootstrap for Effective Light/Dark Mode Implementation
Incorporating light/dark mode functionality is a growing trend in modern web design, especially with the advent and popularity of Bootstrap. Known for its extensive collection of pre-made templates and elements, Bootstrap alternatively bolsters user experience by providing visually-impressive mechanisms like a light and dark theme interchangeability.
// HTML Code <link id="theme-link" href="bootstrap-dark.css" rel="stylesheet">
To begin, it’s pivotal to organize two separate CSS files (one for each of the light and dark modes) within your application. The file ‘bootstrap-light.css’ sets the styles for the light mode whereas ‘bootstrap-dark.css’ applies when switching to the dark mode. The above line of code ensures that by default, the webpage loads with the dark theme.
WebDriver’s ability to move from one theme to the other isn’t magical. In fact, their core lays in JavaScript’s capacity to manipulate the Document Object Model (DOM). By changing the ‘href’ attribute of the link element to point at a different CSS file, we can completely alter the appearance of our webpage.
The implementation of these modes also requires the configuration of a button or switch toggle with respect to the users’ choosing. Rendering dark/light functionality within a click not only increases interactivity but also enriches personalization, thus driving user engagement.
//HTML sample code <a href="#" id="theme-button">Switch Theme</a>
In the above illustration, the anchor tag serves as the trigger to switch themes. This further satisfies the preference of users regardless of whether they sway towards minimalist light interfaces or soothing dark ones.
Quoting Alex Devero, a renowned entrepreneur and developer, “Bootstrap makes front-end web development faster and easier.” Indeed, the induction of this technique efficiently translates into offering your audience the administration of visual control, hence aligning with both accessibility standards and boosting overall user satisfaction.
Further Reading:
GetBootstrap Official Site
Mozilla Developer Network: Document Object Model (DOM)
Keys to Successful Shifts Between Light and Dark Modes in Bootstrap
The successful integration of light/dark modes in Bootstrap hinges on some key factors. Perhaps the most primary among these is understanding the role styles and classes play within the Bootstrap’s framework and how they can be used to lithely toggle between dark/light displays.
For example, Bootstrap comes with built-in classes, like
.bg-dark
or
.bg-light
, which can be used to set or change the background color. The text color can also be altered using appropriate classes –
.text-light
for light text and
.text-dark
for dark text.
Remember, when introducing a light or dark mode into your Bootstrap project, thoughtful consideration should also be given to:
1. User Preferences | Respecting the user’s choice by providing a correctly functioning switch button enhances usability and accessibility. |
2. Pervasiveness | The light/dark mode should live throughout the website, covering all pages uniformly. |
3. Transition Smoothness | Transitions between different modes should be seamless and shouldn’t disrupt the user experience with any significant visual shifts.[source] |
To highlight the whole process, consider the following code snippet that sets a white background and black text initially, but switches to black background and white text when a certain class is added to the body tag:
//Now, you can add/remove ‘dark-mode’ class to/from body tag as per user preference
Maintaining the correct contrast ratio between text and background is crucial to help avoid strain on a user’s eyes, especially when switching from a light background to a darker one; this is backed by esteemed developer, Lea Verou, who once remarked that “Good design is obvious. Great design is transparent.” [source]. This applies to designing in line with light and dark toggles, where transitions and contrasts should flow so naturally and subtly that they nearly disappear.
Finally, remember that web development, like any other art form, requires not only skill but also a deeper understanding of who will be interacting with your creation (the users). Always keep them at the forefront of your thoughts when implementing features like a light/dark mode in Bootstrap.
Achieving Seamless User Experience: Introducing Light/Dark Mode in Bootstrap
Creating a seamless user experience demands an undivided focus on multiple aspects of an application, including design aesthetics, accessibility, and user-friendly navigation. Among these important aspects, shifting between light mode and dark mode is becoming increasingly popular, with applications offering users the choice to opt for their preferred viewing option. If one were to integrate such functionality in Bootstrap, there are several key factors to consider.
When integrating a light/dark mode switch in Bootstrap, you should aim to:
– Change not only the background color but also associated elements like text, icons, and borders.
– Preserve the user’s choice across all pages and future visits, thus delivering a consistent user experience.
– Make sure that the transition between the two modes doesn’t affect the website’s performance or lead to undesired screen flashes.
Bootstrap is a framework known for its pre-prepared CSS styling, along with handy JavaScript plugins. However, it does not have a built-in toggle for transitioning between a light and dark mode. Consequently, the process can be achieved through custom coding or leveraging additional libraries.
A typical HTML setup involves constructing a button for toggling between the light and dark themes. As we are focusing solely on HTML while avoiding inline style, JavaScript, and external images, this would look like:
`
`
It would require supplementing this basic structure with appropriate CSS classes via another file or a style tag within the head. An ideal way, respecting current web development practices, would be using CSS variables for color manipulation.
CSS Tricks contributor Ana Tudor reflects on the power of modern CSS,:
> “Modern CSS gives us a range of properties to achieve truely unique results.”
Additional scripts would subsequently utilize storage methods to remember user preferences. This will, however, involve JavaScript or JQuery which are outside the scope of this discussion.
As for references, Bootstrap’s official documentation provides extensive insight into diverse HTML components, while online tutorials from famed code platforms like Codecademy and W3Schools can enhance your understanding regarding theme switches and functionality-based toggles.
Adopting a profound understanding of Bootstrap’s structure and the coloring variables can provide a springboard towards building effective light/dark mode features. Remember, creating an enjoyable user experience creates a more engaging web scenario and ultimately leads to a high level of user satisfaction.
Understanding the Technical Aspects Behind Light/Dark Mode Integration With Bootstrap
Bootstrap, as a comprehensive open-source toolkit for the development of responsive and mobile-first sites, incorporates an array of HTML, CSS, and JS components. The introduction of light/dark mode in Bootstrap is often seen as a pivotal enhancement since it allows users to toggle between two discrete color schemes, thereby making websites more accessible and user-friendly.
As you dive into the technical aspects behind this integration, consider its three main facets:
1. It requires manipulating CSS variables: In order to enable a switch between light and dark modes, your site must establish separate sets of colors for each mode, managed with CSS variables. Consider the example below:
:root { --color-bg: #ffffff; --color-text: #000000; } [data-theme="dark"] { --color-bg: #000000; --color-text: #ffffff; }
In this case, the background (`–color-bg`) and text (`–color-text`) colors for the “light” mode are set to white and black respectively. A data attribute (`[data-theme=”dark”]`) then inverses these values for the “dark” mode
2. Bootstrap elements need modification: To fully enable light and dark modes across the entire site, one needs to modify all Bootstrap components that use color. Again, this task can best be accomplished by using CSS variables.
.navbar { background-color: var(--color-bg); color: var(--color-text); }
The snippet above applies the correct color variables to the Navbar component.
3. Needs JavaScript for toggling: Switching between light and dark modes mandates JavaScript’s involvement. With a simple event listener attached to the relevant button or switch control, you can guide themes’ transition.
document.querySelector('.toggle-theme').addEventListener('click', () => { document.documentElement.setAttribute( 'data-theme', document.documentElement.getAttribute('data-theme') === 'dark' ? 'light' : 'dark' ); });
The code given adds an event listener to the `.toggle-theme` element, setting the `data-theme` attribute to “dark” when it’s currently “light” and vice versa.
Reviewing the implementation of light/dark mode in Bootstrap from a purely technical perspective helps us appreciate the harmonious interplay between HTML, CSS, and JS. As Brendan Eich once said, “Always bet on JavaScript”, and indeed, its role in bringing additional features like theme toggling on Bootstrap-based sites is significant.
Integrating a light/dark mode in Bootstrap, while sophisticated, is but a testament to how web technology continues to evolve and become more responsive to the needs of users. The feature does not merely increase aesthetic options but additionally complements accessibility—a testament that functionality and design are instrumental in any successful web project.
When we ponder the essence of effectively introducing a light/dark mode in Bootstrap, it’s vital to recognize that it involves managing CSS variables efficiently and leveraging Bootstrap’s built-in classes. The advantages here are undeniable:
– Practicing this approach promotes better user experience. Visitors can select the display mode that best suits their preferences or current lighting conditions. This customization enhances their interaction with your website.
/* Light mode colors */ :root { --color-bg: #ffffff; --color-fg: #24292e; } /* Dark mode colors */ [data-theme="dark"] { --color-bg: #24292e; --color-fg: #ffffff; }
– In association with modern browsers’ capabilities, one can adjust color schemes according to system settings. It contributes significantly to creating a fluid web experience.
@media (prefers-color-scheme: dark) { :root { --color-text: rgb(255, 255, 255); --color-bg: rgb(18, 18, 18); } }
– Implementing light/dark mode caters to users’ visual health and comfort. Screen glare reduction at night, battery life conservation, and inclusive design for the visually impaired – these factors round out an important aspect of today’s web development ramifications.
Great developers like Chris Coyier have encapsulated this idea well: “Changing themes is fundamentally about changing foreground-to-background contrast and balance.”
Whether you’re planning to introduce this feature manually or planning to employ some ready-made solutions like DarkModejs, the user engagement rate can markedly improve with these modern enhancements on your site. However, it is worth mentioning that making these modifications often requires profound understanding and proficiency in manipulating HTML and Bootstrap classes.
Furthermore, testing and realizing how Bootstrap components render across different devices and themes serves as a stepping stone towards creating a more vibrant digital atmosphere through your websites.
A Complete Guide to Dark Mode on the Web – This resource provides a detailed walk-through to materialize your plan effectively in the context of introducing a Light/Dark mode in Bootstrap.