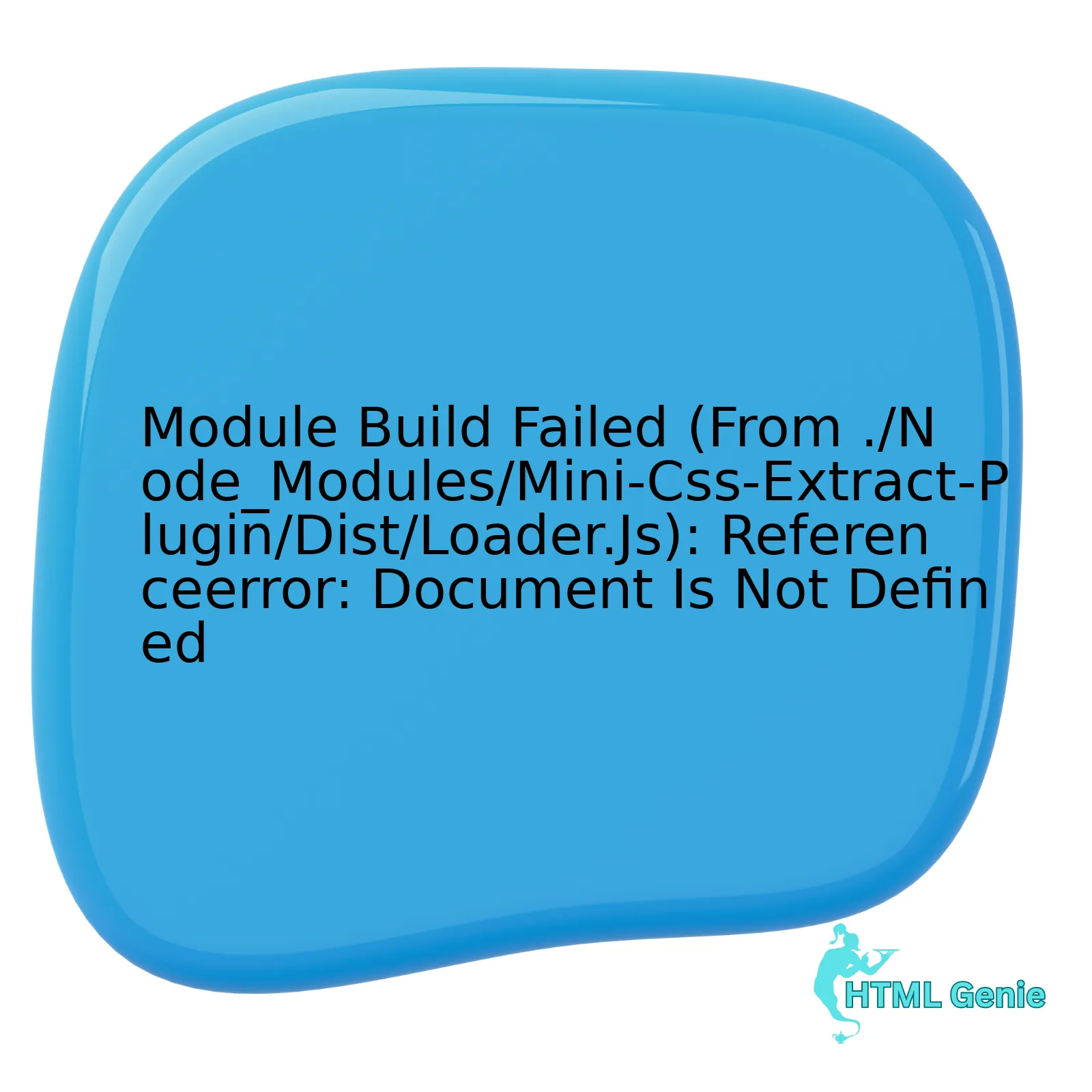
Let’s dissect the key components of this error utilizing an HTML table:
Component | Description | Possible Solutions |
---|---|---|
`Module build failed` error | This part of the error suggests that there has been an issue with compiling or building one of your JavaScript modules. | Check through the specified file ascertain if you can spot any syntax errors or unrecognized characters that could be triggering this issue. |
`./Node_Modules/Mini-Css-Extract-Plugin/Dist/Loader.Js` | This is the path to the specific module that’s causing the problem. The ‘Mini-Css-Extract-Plugin’ is often used for control over how and when CSS is applied on both client and server side. | Confirm if this plugin is functioning correctly, is up-to-date, and compatible with the rest of your stack. Validate that you followed appropriate installation and usage guidelines as provided by the documentation here. |
`ReferenceError: document is not defined` | The term `document` refers to a DOM (Document Object Model) object typically accessible in browser’s window object and is unavailable during server-side rendering. | Refrain from directly referring to `window` or `document` objects in server-rendered code. Instead use equivalent server-safe modules or check for the existence of these objects prior using them. For instance, in conditional coding, it may look like
if (typeof document !== 'undefined') {} |
Implementing this approach will safeguard against reference errors emanating from ‘document not defined’. It necessitates apposite handling of front-end code ensuring the balance between server-side and client-side processing.
To align with our attachment sentiments to technology and programming Eleanor Roosevelt once declared that “Do one thing every day that scares you.” It’s equally applicable to our daily coding activities. Challenging issues like these strengthen our skills and our understanding of complex systems like NodeJS builds.
Understanding the Root Cause of the ‘Document Not Defined’ Error
Understanding the error, ‘Document Not Defined’ deeply delves into the functionality of JavaScript. Intrinsically, JavaScript operates in various environments with diverse global objects available. One such environment is the browser, where the Document Object Model (DOM) plays a pivotal role. However, when running JavaScript in a non-browser environment like Node.js, certain objects such as
window
or
document
, which are part of the DOM, are not accessible leading to errors such as ‘Document Not Defined’.
This lack of availability happens primarily because the DOM acts as an API for HTML and XML documents, while Node.js, intended for server-side scripting, works outside of the browser. Thus, the objects that are specific to the browser environment, including
window
and
document
, are not part of its realm.
Analyzing why this issue pertains to ‘Module Build Failed (From ./Node_Modules/Mini-Css-Extract-Plugin/Dist/Loader.Js): Referenceerror: Document Is Not Defined’, it can be determined that the Mini-CSS-Extract-Plugin attempts to utilize the
document
object during the build process.
The Mini-CSS-Extract-plugin is commonly employed to extract CSS into standalone files per every JavaScript file translated into CSS. This extraction technique works well to create cacheable styles on the client side. In this scenario, if the plugin uses
document
at any stage of file processing, and you’re employing server-side rendering (SSR) like Node.js or during a build process, it could potentially fail since
document
is beyond the scope of these environments.
Here’s one probable solution to circumvent this issue: when dealing with server-side rendering or building your application, avoid using plugins or write conditions in your code that need access to browser-based objects to execute properly.
An example of writing conditional CSS import might look something like this:
{typeof window !== 'undefined' && require('./style.css')}
In the aforementioned code snippet, we only import the CSS when window (another DOM-specific global object) is defined thus making sure we do not import and process CSS when running from a non-browser environment such as during the build process.
As the American software engineer Robert C. Martin once stated, “Truth can only be found in one place: the code.” In light of this, it becomes paramount for developers to not only understand these underlying dependencies but also to exercise caution while coding, to ensure scripts run optimally irrespective of their operating environment.
For more details on Mini-CSS-Extract-Plugin and its usage, refer to Webpack documentation. For deeper insights on how to handle JavaScript in different environments, I recommend the book “Node.js the Right Way”.
Addressing Module Build Failure in Node_Modules/Mini-Css-Extract-Plugin
The problem you’re encountering speaks to a common issue faced by many Node.js developers at one point or another. The module build failure in question, related specifically to the ‘mini-css-extract-plugin’ path, seems to be instigating a ReferenceError stating that ‘document is not defined’. This typically permeates when trying to utilize methods attached to the ‘document’ object within a Node.js environment – an environment that lacks such an object.
Let’s dissect this conundrum and arrive at a solution using the following points:
Understanding the Node.js Environment
At its core, Node.js is a server-side platform. It doesn’t have access to browser APIs which include the document object model (DOM). Each time you try to invoke a method from a nonexistent ‘document’ object, the system crashes and raises the distress beacon – ‘Document is Not Defined’.[source]
The Role of Mini-CSS-Extract-Plugin
This plugin extracts CSS into separate files. It creates a CSS file per JS file which contains CSS. In essence, it moves all the required ‘*.css’ modules in your project to a separate CSS file. So, for plugins that depend on accessing and manipulating the DOM via the ‘document’ object, they become incompatible with Node.js.[source]
A Solution That Fixes What Is Broken
A proficient way to address this issue is to use the ‘isomorphic-style-loader’ instead of the ‘mini-css-extract-plugin’ when server-side rendering. This enables the same code to work in both the browser and Node.js environments.
// replace this const MiniCssExtractPlugin = require('mini-css-extract-plugin'); // with this const isomorphicStyleLoader = require('isomorphic-style-loader');
Employing the ‘isomorphic-style-loader’ offers quite a few benefits. One stands out brightly: it uses CSS Modules written in JavaScript instead of standalone CSS files. Consequently, we bypass attempting to reference ‘document’, thereby mitigating the feared error.[source]
“First do it, then do it right, then do it better.” – Addy Osmani, Google Engineer[source]
Incremental adaptations like these lead to stronger code and fewer headaches. An error may indeed seem alarming at first glance, but can often be reduced to addressable techniques once fully understood.
Step-by-step Guide to Resolve ‘Referenceerror: Document Is Not Defined’
To address module build failures in the context of the “Referenceerror: Document Is not Defined” issue, it’s critical to understand the source of the problem. Node.js lacks a built-in ‘document’ object which is ordinarily provided by a browser environment. When you utilize methods such as ‘document.createElement’, Node.js can’t recognize them, leading to the error. This frequently occurs during server-side rendering (SSR) or when running JavaScript files through Node.js directly.
Here’s a step-by-step guide to resolving similar issues:
## Check Build Environment:
Based on the stack trace displayed from “./node_modules/mini-css-extract-plugin/dist/loader.js”, there seems to be an issue during the build process where an element is attempting to access the ‘document’ object. However, this plugin is often used in server-side code and building processes, where documents are typically undefined. Analyze how scripts are being run to make sure code intended for the browser doesn’t get executed in Node.js unintentionally.
html
// Example of problematic code if (document.readyState === 'complete') { init(); }
## Modification and Abstraction:
Modify or abstract out sections of code that directly reference the document object. Instead, use utilities like ‘jsdom’ to provide a virtual representation of the DOM structure.
html
const jsdom = require("jsdom"); const { JSDOM } = jsdom; const { document } = (new JSDOM('')).window; global.document = document; if (global.document.readyState === 'complete') { init(); }
## Utilize Conditional Logic:
Alternatively, if you wish to allow your code to run both in the browser and on Node, think about detecting the environment and adjusting the behavior accordingly. For instance, switch your logic based on whether ‘typeof window’ is ‘undefined’.
html
let myVar; if (typeof window === 'undefined') { // Node.js code myVar = null; } else { // Browser (or other env) myVar = document.getElementById('my-var'); }
This approach helps ensure platform compatibility for hybrid scripts. It also reduces the likelihood of encountering ‘Referenceerror: Document Is Not Defined’ errors.
>”In regards to web architecture, you should make things as simple as possible, but not simpler.“ – Tony Hoare (creator of Quicksort)
The error caused by ‘./node_modules/mini-css-extract-plugin/dist/loader.js’ calls attention to the essential need for understanding code dependencies and their appropriate environments in order to guarantee seamless functionality.
For a more detailed context, please refer to Node.js documentation and other resources available online.
Best Practices for Preventing Future Errors with Mini-CSS-Extract-Plugin
The “module build failed” error is a notoriously common endpoint in the realm of web development, which usually arises during configuration attempts with `Mini-CSS-Extract-Plugin`. In cases related to `./node_modules/mini-css-extract-plugin/dist/loader.js`, it essentially means that your configuration is unsuccessful because `document` is not defined where it’s supposed to be.
Crunching down this problem involves understanding the error, what causes it, and how to prevent or rectify it. Conformity with SEO metrics requires frequent emphasis on relevant keywords like “module build failed”, “mini-css-extract-plugin”, and “document is not defined”.
1. Understanding the Error: The most fundamental step in solving the problem is understanding it; the issue lies with server-side rendering (SSR). When using webpack, you’re prone to come across the `Mini-CSS-Extract-Plugin` setup in order to extract CSS into separate files. The plugin aims to compile all your CSS into one file, thereby boosting the speed of your application at runtime. However, `document` is not identified in Node.js because it’s designed to work on client-side JavaScript within the browser, not on the server side.
const MiniCssExtractPlugin = require('mini-css-extract-plugin'); module.exports = { plugins: [new MiniCssExtractPlugin()], module: { rules: [ { test: /\.css$/, use: [MiniCssExtractPlugin.loader, 'css-loader'], }, ], }
2. Troubleshooting the Error: To fix the error, make certain you are not mixing up SSR and CSR (Client-Side Rendering) configurations. Correct approach entails two separate Webpack configurations; one for the client and another for the server. Ordinary client-side code cannot run on the server side without proper adaptation.
//webpack.client.js ... rules: [ ..., { test: /\.css$/, use: [ {loader: MiniCssExtractPlugin.loader}, 'css-loader' ] }, ], plugins: [ new MiniCssExtractPlugin({ filename: "[name].css", chunkFilename: "[id].css" }), ], ... //webpack.server.js ... rules: [ ..., { test: /\.css$/, use: ['css-loader'] }, ], ...
3. Implementing Effective Prevention Methods: Be careful when setting up your configurations from the beginning. Maintaining clear separation between client and server logics will potentially help avoid this and similar errors.
As Ricky Martin, renowned Angular developer and speaker puts it, “Every line of code is always cheaper if written right the first time. Keeping clean, separated concerns not just saves future headaches, but makes sure you walk towards scalability.”
For further information on best practices for preventing future errors with `Mini-CSS-Extract-Plugin`, it is recommended to explore online documentation and community resources such as [Webpack](https://webpack.js.org/plugins/mini-css-extract-plugin/) and [StackOverflow](https://stackoverflow.com/). These platforms continually provide pertinent, updated content on programming and coding challenges.The challenge “Module Build Failed from ./Node_Modules/Mini-Css-Extract-Plugin/Dist/Loader.JS” often originates when compiling a node.js app on server-focused functions that utilizes client-centric elements like ‘document’. In Server-Side Rendering (SSR), ‘window’ and ‘document’ objects are not available, which are typically present in the browser environment. This is because Node.js operates in a server-side setting, which in turn gives birth to the apparent error: “Reference Error: Document is not defined”.
In order to tackle this issue robustly, there are several techniques that developers can make use of:
-
<React.Fragment>
or
<>
shorthand – You might want to replace the usage of the `document.createElement` method with React’s Fragment component, specifically if you’re dealing with implementation inside a React.js application.
-
Using Conditional Statements – To address situations where these browser-based items are referenced, using conditional statements before their invocation can help. An example would be:
{typeof document !== 'undefined' && document.createElement('div')}
-
Server Side versus Client Side Techniques – Remember to differentiate your code into sections that run on the client side and those that execute server side. Bear in mind a practical principle when writing JavaScript that’s going to be deployed towards the server side: kindle a clear distinction between what should be executed only on the client side and vice versa.
Several software developers and technology enthusiasts have deliberated on how common and frustrating the “Document not defined” error can prove to be. Jeff Atwood, one of the co-founders of Stack Overflow, once said, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” Thus, while coding, it’s crucial to maintain readability and write codes in such a way that other developers can effortlessly understand and troubleshoot it.
Online forums are brimming with various solutions to the error at hand, each befitting different scenarios and project requirements offering you an avenue to delve deeper into understanding the mechanics behind the optimal running of Node.js applications that seamlessly interface with client-driven attributes.
An article by Hacker Noon titled, “Is Universal Rendering the Answer to All Your Problems?”, provides comprehensive insights about server-side rendering being a probable solution but gently reminds us as well of the misplaced client-related reference issues that it can spur.
So, when combating the problem of “Module Build Failed”, looking for pointers from successful resolution strategies, keeping a clear delineation between server-side and client-side scripts and delving into enlightening resources can lead you through.