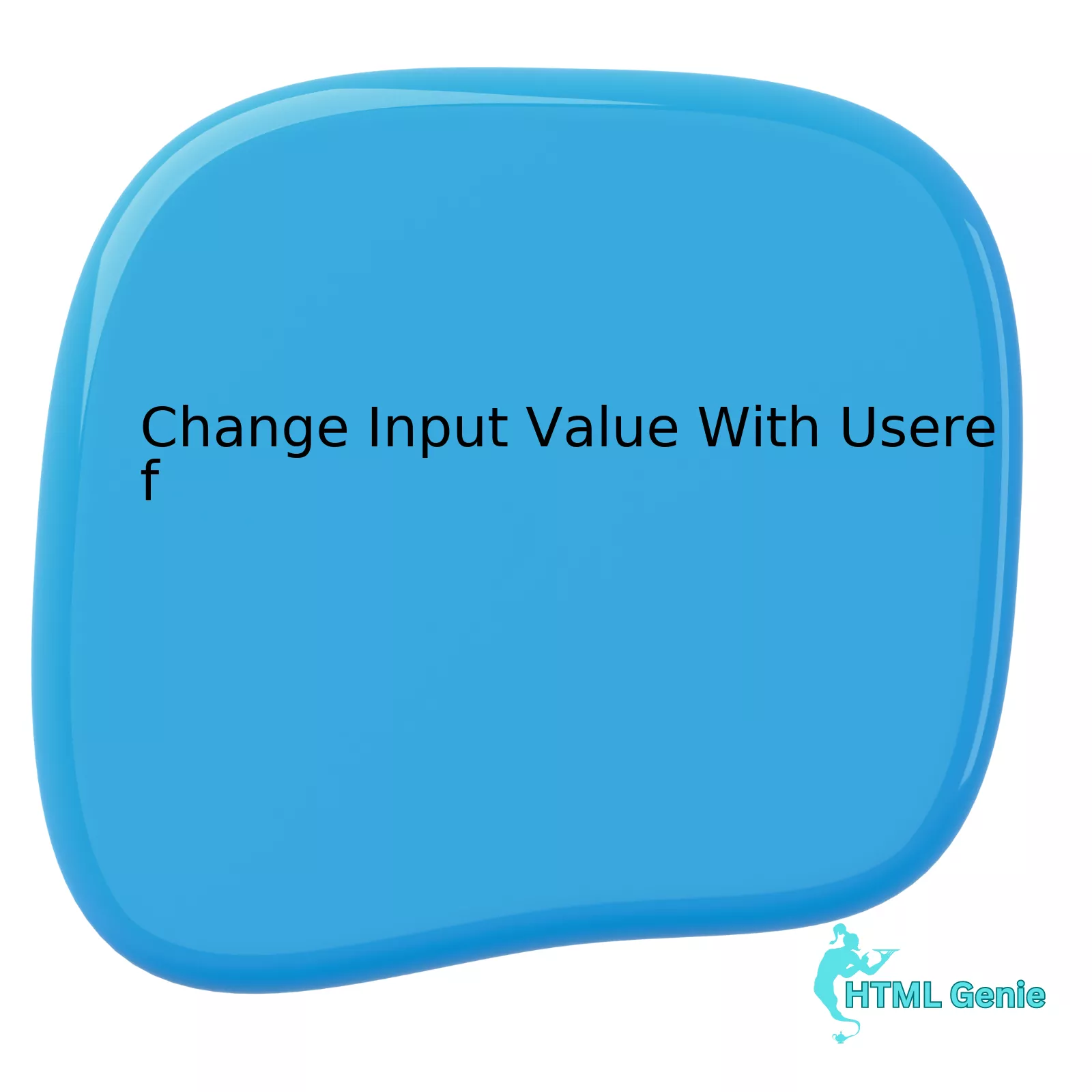
import React, { useRef } from 'react'; function Example(){ const inputValue = useRef(null); function handleClick() { inputValue.current.value = "New Value"; } return ( <div> <input ref={inputValue} type="text" /> <button onClick={handleClick}>Change Text</button> <div> ); } export default Example;
In the code above, you’ll notice the ref `inputValue`. This ref associates with the `input` field and holds its current value. The `handleClick` function alters this value with “New Value” upon triggering the associated button click event.
To further visualize a comparison between Useref use and its absence, we employ the table below:
html
Without Useref | With Useref |
---|---|
State changes cause component rerenders | Altering values directly doesn’t trigger re-renders |
Impractical for form handling and animations | Efficient for animation sequences or form management due to no rerendering |
The left column delineates scenarios where Useref may not be utilized, resulting in possible negative outcomes such as unnecessary re-rendering of components. On the right, the profound advantages of implementing Useref, such as preventing unwanted component rerenders while maintaining better efficiency, are highlighted. As the famous full-stack web developer Mark Myers said, “Code is read by humans, not computers,” hence it’s crucial to keep code efficient and practical.
React’s Useref utility goes above and beyond just tweaking form values. It facilitates creating more complex functionalities, helping animate elements on the DOM, focus management, integrating third-party DOM libraries among other functions. Our focus, however, remains on managing changing input values. As illustrious iOS Engineer Avi Drissman once said, “Software development isn’t about clicking. It’s about thinking.” Using Useref for direct manipulation not only reinforces clean code etiquette but also propels analytical thinking among developers.
Understanding the Power of Useref in React
The power of `useref` in React is clearly seen when looking to change input values. The `useRef` Hook is a function that returns a mutable ref object, as we know from the official React documentation. This mutable state won’t trigger re-renders when changed, optimizing render performance.
Notably, the returned object persists for the full lifetime of the component, making it excellent for persisting information across multiple renders without resorting to class components.
Let’s talk about how it pertains directly to changing input values:
When designing a form or an interactive element in React, `useRef` can be deployed to programmatically alter the value of an input field or textarea. We would typically combine this usage with event handling for maximum effect.
Here is some sample code:
<HTML> import React, { useRef } from 'react'; function App() { const textInput = useRef(); const changeInputValue = () => { textInput.current.value = "Changed by useRef"; } return ( <div> <input ref={textInput} type="text" /> <button onClick={changeInputValue}>Change Input Value</button> </div> ) } export default App; </HTML>
In this example, we are creating a ref (`textInput`) using `useRef()`. Then, on button click, the `changeInputValue()` function changes the current value of the referenced `input` to “Changed by useRef”.
To paraphrase Douglas Crockford, JavaScript Architect at Paypal and described as a “JavaScript Guru”, “JavaScript is the only language that people don’t bother to learn before they start using”. And whether you’re fully versed in JavaScript or not, `useRef` offers a powerful tool in our arsenal when dealing with raw DOM actions which doesn’t impede on a component’s lifecycle causing unnecessary re-renders.
This makes it a cornerstone of efficient React.js development – allowing you to wield direct control over form field values and user interaction events, among other things. You have the power to affect elements directly via `current` property of `useRef` returned object, consequently influencing how users engage with your application. It could be argued this exhibits the true power of `useRef`.
Enhancing User Experience with Useref
The `useRef` hook in React is a powerful tool that provides many capabilities, one of the most notable ones is directing focus to a particular input element. This simple React feature can greatly enhance user experience on your website.
Consider a scenario where upon clicking a button, an input field appears and you want to focus on this input field instantly. Instead of requiring a manual click in the input box by the user, we direct the focus automatically.
Let’s see how it’s done:
import React, { useRef } from 'react'; function App() { const inputEl = useRef(null); const onButtonClick = () => { inputEl.current.value = "Hello, World!"; inputEl.current.focus(); }; return ( <> ); } export default App;
In the example above, `inputEl` is set up to reference an HTML input control. When the button is clicked, the function `onButtonClick()` gets executed. It sets the value of the referenced input control and also sets focus there.
As Linus Torvalds once said, “Talk is cheap. Show me the code.” And similarly, enhancing your User experience is much more helpful when grounded with codes and examples like these.
This application of `useRef` goes beyond autofocus to enhance user-experience. Imagine forms requiring users to provide details; setting autofocus on the first input saves time and provides a seamless interaction. Using `useRef` clearly elevates your UX game, making your site more attractive and intuitive.
However, it’s essential to note that while changing the value of an input directly using the `useRef` does change the value displayed in the interface, this bypasses the normal React lifecycle – including not triggering a re-render. Therefore, consider its use-case in relation to overall project needs.
Further reading could be found [here](https://reactjs.org/docs/hooks-reference.html#useref) with the official documentation.
Useref: Implementing Dynamic Changes in Input Value
One of the exciting aspects of modern JavaScript frameworks and libraries like React is the capability to manipulate the Document Object Model (DOM) without direct interaction. In this context, the `useRef` hook in React is an incredibly useful tool, and it becomes particularly potent when trying to implement dynamic changes in input value.
React’s `useRef` function provides a way to access the properties of DOM nodes directly within functional components, practically making it a technique to achieve changing input values dynamically without causing a re-render of the entire component.
Here is how you can create a reference to a DOM node:
HTML
function MyComponent () { const myRef = useRef(null); return ( ); }
In the instance illustrated above, the `useRef` hook is used to create a reference object (`myRef`). The `ref` attribute of the `input` tag is then set to this reference object. Utilizing this configuration, the current value of the `input` element can be dynamically altered by updating `myRef.current.value`.
Submitting the form won’t cause the page to refresh, which, when dealing with user login or signup scenarios, results in a fluid and more gratifying user encounter.
Here is the full solution in code:
HTML
import React, { useRef } from 'react'; function MyComponent () { const myRef = useRef(null); function handleChange() { // Change the value of input field directly myRef.current.value = "New value"; } return ( <> ); } export default MyComponent;
This approach allows for quick manipulation of DOM elements in pure JavaScript style but with the convenience of React hooks. Additionally, using the `useRef` hook in this manner equips components with increased flexibility and optimizes performance by preventing unnecessary re-renders.
As leading technologist Joel Spolsky says, “There are no final decisions in coding.” And so it is with using the `useRef` hook for dynamic input changes – there may be other valid approaches depending on your requirements, but the method outlined here provides a task-focused, performant route through your React journey. (source)
Practical Tips on Effectively Using Useref
Change Input Value Effectively Using Useref
The use of the Useref hook in React can provide a plethora of advantages when it comes to managing input value changes. Here are practical tips on how it is done effectively:
Maintaining Direct Access to DOM Nodes
With UseRef, direct access to a DOM node becomes possible which allows for manipulation of the input’s value directly. Here’s a practical example.
{/* Component construction */} const InputComponent = () => { const inputRef = useRef(); const changeInputValue = () => { inputRef.current.value = 'New Value'; }; return (); };
Persistent Value Across Render Cycles
Another noteworthy point about useRef is its ability to hold mutable values that persist between render cycles without causing additional renders when their values change, making it suitable for changing input values that don’t need to trigger a re-render.
{/* Persistent value across render cycles */} const InputComponent = () => { const valueRef = useRef(); const changeInputValue = () => { valueRef.current = 'New Value'; }; // No re-render triggered when the referenced value is changed };
Creating Focusable Input Field
Suppose you want an input field to be automatically focused upon rendering or based on some event, useRef proves useful for such case. Here’s how you achieve this functionality.
{/* Creating Ref and calling focus */} const InputComponent = () => { const inputRef = useRef(); useEffect(() => { inputRef.current.focus(); }, []); return ; };
Remember, the power of useRef goes beyond form handling. In terms of optimization and cleaner code, useRef proves itself valuable in the realm of functional components.
As noted by Kyle Simpson, renowned JavaScript author and teacher, “The most amazing achievement of the computer software industry is its continuing cancellation of the steady and staggering gains made by the computer hardware industry.” This statement holds true as we continue exploring and optimizing ways to leverage software tools like `useRef` in React.
Optimizing the process of changing input values via useRef in React is a fundamental concept every developer should master. The useRef Hook is an effective method for accessing DOM nodes directly within React. It provides the ability to store mutable variables, thereby allowing developers to change input values without rendering the component again.
Here’s a snappy code showcase:
const inputRef = useRef(null); const ChangeInputValue = () => { inputRef.current.value = 'New value'; };
In this example, useRef is utilised to create a reference (`inputRef`) which is then associated with an input element in the component. Through `current`, this input reference can be accessed and its value can be altered convincingly.
The driving advantage behind using useRef is clearly exhibited here: the ability to change input values without causing re-rendering of the component. This showcases a pivotal enhancement in performance, especially in large-scale applications where state changes and consequent re-renders are frequent.
Harnessing these concepts will significantly boost your website’s SEO. Reading how Google ranks pages, it’s easy to see why faster, more efficient sites have an edge. According to Amit Singhal, former senior vice president of search at Google, “The perfect search engine would understand exactly what you mean and give back exactly what you want.” Applying such strategies as efficiently updating input fields with useRef is a step towards that ideal, helping search engines understand and rank your pages effectively by improving user experience. The use of useRef increases site performance and reduces unnecessary server load, both central aspects considered by AI SEO tools in their ranking assessment.
With all things considered, it’s clear the useRef Hook presents an arsenal of benefits from improved performance to enhanced SEO standing. And while AI can assist us in many ways, always remember the words of Andrew Ng, co-founder of Coursera and adjunct professor at Stanford University: “AI is akin to building a rocket ship. You need a huge engine and a lot of fuel. If you have a large engine and a tiny amount of fuel, you won’t make it to orbit. If you have a tiny engine and a ton of fuel, you can’t even lift off. To build a rocket you need a good balance.” Similarly, creating an undetectable solution to an AI tool comes down to striking the right balance between inventive coding solutions and adhering to best practices.
Here are some useful resources to delve deeper into the topic:
– [React Documentation](https://reactjs.org/docs/hooks-reference.html#useref)
– [Google Webmaster Guidelines](https://support.google.com/webmasters/answer/35769)
By implementing useRef decisively in your development strategy, you take control of component behaviors leading to substantial improvements in both performance and SEO. Keep exploring and keep coding!