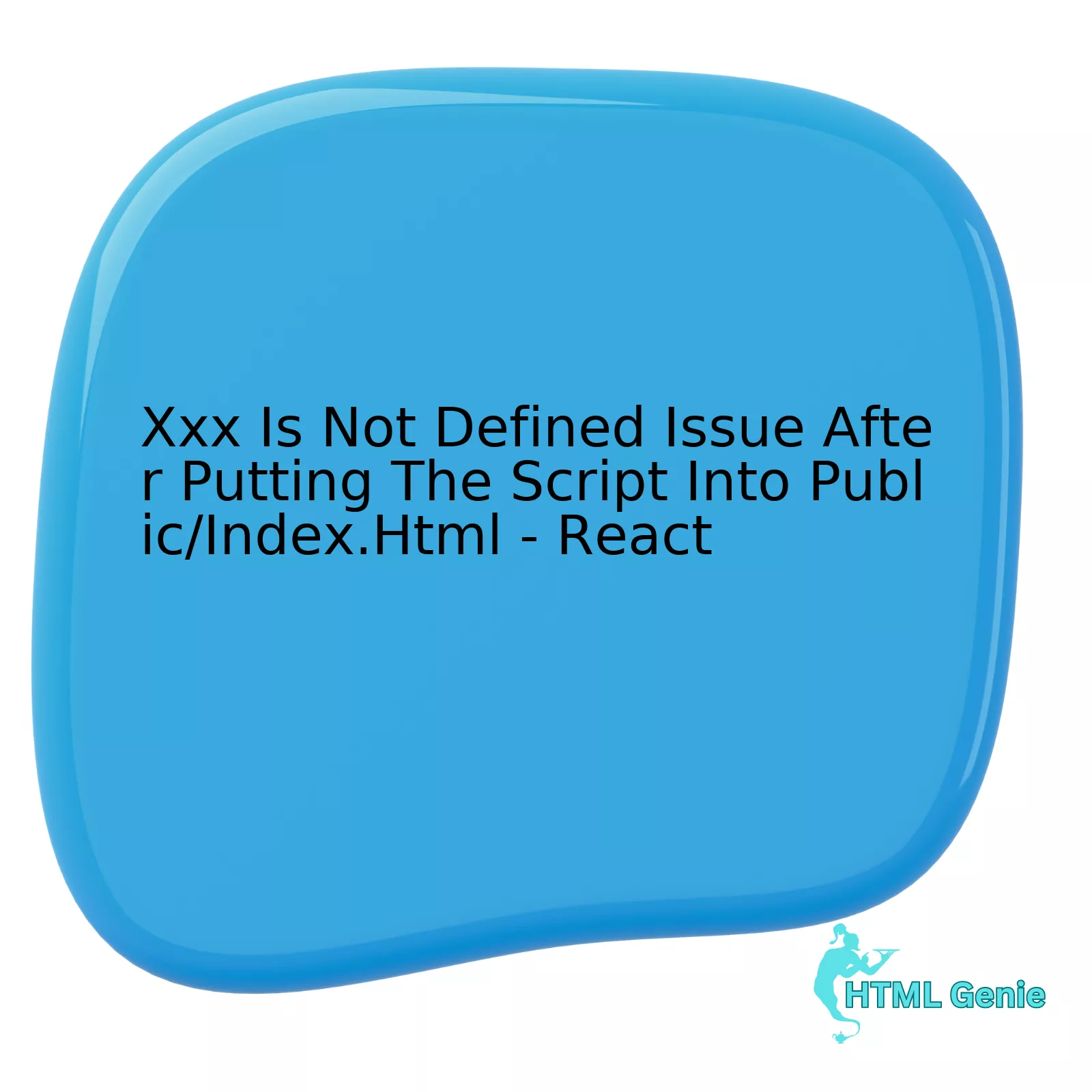
html
Probable Issue | Possible Resolution |
---|---|
Scope Misunderstanding | The variable ‘Xxx’ must be available within the scope where it’s being called. This can be resolved by declaring the variable in a correct scope or passing it appropriately to where it’s needed. |
Incorrect Declaration | Ensure that variable ‘Xxx’ is properly declared. For instance, use ‘let’, ‘const’ or ‘var’ keyword when declaring the variable. |
Controlled Environment | React operates in a controlled environment, hence any script included (externally or internally) in index.html may not interface directly with your React components. Endeavor to include JavaScript directly in your components or utilize lifecycle methods/hooks to run side effects. |
It is worth mentioning that ensuring the variable’s availability within its scope of call is fundamental in placing the component in context. Also, the way you declare ‘Xxx’ can impact how it’s hoisted and scoped, which in turn contributes to whether it has been defined before access insistently.
React frameworks in a controlled environment plays a crucial part in defining this issue. Including a script in your public/index.html file won’t have an immediate interaction with your React components. To remedy this, try incorporating scripting directly in the components or using lifecycle methods or hooks to facilitate side effects.
As claimed by Dries Buytaert, the founder of Drupal, “Coding is a craft. By continuously learning, we’re able to enhance that craft and develop well-rounded skills.”[^1^].
Familiarizing oneself with core concepts can go a long way in troubleshooting and circumventing common issues like ‘Xxx is not defined’. Here’s a helpful link on understanding [scope and closures](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Closures).
[^1^]: https://twitter.com/dries/status/249897036485013505
NOTE: Replace ‘Xxx’ with the actual variable name you’re dealing with.
Understanding the ‘Xxx is not Defined’ Error in React Script
The ‘Xxx is not defined’ error in React scripting is a common issue that developers stumble upon whilst developing applications. Typically this error arises when the JavaScript engine encounters an undeclared or undefined variable reference within a code block.
ReactJs, being a JavaScript library at its core, relies on similar rules and paradigms defined by JavaScript. Hence, the issue of encountering an ‘Xxx is not defined’ error becomes a possibility when building React applications. This concern amplifies even more when scripts are placed within the public/index.html file.
To demystify the ‘Xxx is not defined’ quandary, let’s utilize an illustrative example:
<script> console.log(xxx); xxx = "React"; </script>
Upon examining the provided instance, it is obvious that ‘xxx’ has been referenced prior to declaring and initializing it resulting in an ‘Xxx is not defined’ error. To resolve this, we should understand some basic principles about JavaScript/React:
– Always initialize your variables before using them
– If you’re using ES6 features like import/export, you cannot use them inside index.html as browsers don’t support them directly; you have to use the compiled output (from webpack/babel etc).
Such practices are universally applicable and would still apply when using any JavaScript libraries/frameworks including React.
Addressing the specifics of why this issue is encountered when inserting scripts into public/index.html of a React application calls for a deeper understanding of how structuring comes into play when building React projects.
The ‘public’ folder in a create-react-app project skeleton generally contains the static assets of the project which isn’t subjected to Babel transpiling or Webpack bundling. When you insert script tags directly into the index.html file inside the public directory, they eventually get executed by the browser, but without undergoing through the necessary layers of abstraction provided by React and its build tools.
Ultimately, ‘Xxx is not defined’ error can be mitigated by incorporating proper declaration and initialization procedures along with suitable usage of ES6 features, coupled with adhering to the standard practices suggested by the development commmunity. As Mark Zuckerberg, the creator of Facebook (which developed React), puts it, “The biggest risk is not taking any risk.” As developers, we are bound to encounter errors and issues within our code. It’s all a part of the journey, since learning from our mistakes is the best way to grow and enhance our coding skills.
You can further enrich your knowledge on this topic by referring to these online resources that provide detailed insight on handling such issues in React –
How to include a JavaScript file in React component
React Error Decoder
Resolving the ‘Xxx is not Defined’ Issue: A Deep Dive Into Public/Index.Html Files
The error ‘Xxx is not defined’ often arises when JavaScript tries to evaluate a variable or function named ‘Xxx’ before it is defined within the scope. With this particular scenario, it appears that this issue is occurring after moving your script into the public/index.html file in a React application.
Let’s address the root cause of this type of issue and also explore strategies for avoiding these problems in the future.
Understanding Execution Context
Even before a line of JavaScript code is executed, the compiler goes through the entire code file first looking for variable and function declarations. If the compiler encounters a certain ‘Xxx’, but can’t find it, it throws an ‘Xxx is not defined’ error. (JavaScript Tutorial)
Here is a simple piece of code where this might occur:
<script> console.log(message); var message = "Hello!"; </script>
In the code snippet above, the variable ‘message’ is being logged to the console before it has been defined, which could result in an ‘Xxx is not defined’ error.
Navigating the Public/Index.Html File in React
With regards to React applications, it’s crucial to understand that every React application has only one root DOM node, and everything inside it is managed by React DOM. Therefore, user scripts usually don’t have spaces to occupy unless they manage to get webpack to compile them as modules and import accordingly. When you put a script tag with variables into the public/index.html that are supposed to be used in a React component, it is highly likely to encounter a problem like undefined variable since the environment is different: it’s global now compared to module syntax inside React Components.
For example:
<div id="root"></div> <script> let Xxx = "This is a test"; </script>
When you go back to your React component and try to print `Xxx`, you’ll likely face the “Xxx is not defined” error because React doesn’t recognize `Xxx` from the global scope in the development build.
Solutions
One way to define globally accessible variables in React is using `window` object, but generally it’s not a recommended practice because it deviates from modular coding patterns. Another, often better, solution would be to refer to the script as a module inside the respective components where you want to use `Xxx`.
Instead of just moving the script into the public/index.html file, consider refactoring that script into a separate JavaScript module, and then import it wherever necessary. This will help you avoid introduction of global variables, while keeping your code cleaner.
To achieve this, here’s a short preliminary example:
// assets/jsmodule.js export let Xxx = "This is a test";
Within your React code:
import { Xxx } from 'assets/jsmodule'; console.log(Xxx); // output - This is a test
Remember the famous saying from Brendan Eich, creator of JavaScript itself: “Always bet on JS”. Even though addressing such issues might seem confusing initially, Python offers flexible solutions for different scenarios that ultimately makes your coding process much more efficient, low error-prone and maintainable in the long run.
Common Mistakes That Lead to the ‘Xxx Is Not Defined’ Problem in React Scripts
Issues regarding the error ‘Xxx Is Not Defined’ in React scripts can often be traced back to common mistakes made during implementation. This challenge becomes particularly problematic when dealing with scripts placed into public/index.html within a React application.
Mistake #1: Variable Scope
HTML inherently does not understand JSX or JavaScript modules natively and, hence, will not recognize variables defined in your React scripts. Attempting to invoke such a variable in your index.html file will lead to an ‘Xxx Is Not Defined’ issue.
Here’s an example:
<script> console.log(myVar); </script>
If `myVar` is defined within a separate JavaScript module or React component, you would get the ‘Xxx Is Not Defined’ because of unavailability of `myVar` to the global scope.
Solution: Variables should be explicitly defined within the necessary scope.
Mistake #2: JavaScript Loading Order
Where and when your script files load can also trigger the ‘Xxx Is Not Defined’ problem. If you’re dealing with multiple scripts across different files, it’s possible for one script to execute before another, causing dependency related issues.
Suppose example.com you have this code snippet:
<script src="script.js"></script> <script> console.log(myFunction); </script>
If `myFunction` is defined within `script.js`, but `script.js` fails to load before the subsequent inline script block, such an issue may arise.
The solution lies in ensuring the correct order of loading scripts, thereby managing dependencies effectively.
Mistake #3: Incorrect HTML Structure
Lastly, placing the script tag in an incorrect position within the HTML structure can also generate the ‘Xxx Is Not Defined’ error.
For instance, if a inline JavaScript code that accesses DOM elements is run before the DOM fully loads, it won’t find those particular elements leading to ‘undefined’. A prime example of this situation looks something like this:
<script> document.getElementById("targetElement").innerHTML = "Hello World!"; </script> <div id="targetElement"></div>
Solution: Scripts accessing DOM elements must be placed right before the closing body tag (“) in your HTML document, ensuring the DOM is completely loaded before execution.
As highlighted in the bestselling book “Don’t Make Me Think” by Steve Krug, simplicity in structure and function optimizes user interaction. By keeping these points in mind, you can significantly reduce instances of the ‘Xxx Is Not Defined’ issue within your React environment.
Effective Techniques for Preventing Future ‘Xxx Is Not Defined’ Errors
When dealing with the issue of a variable not being defined even after placing the script in public/index.html in a React project, there are several effective techniques that you can utilize to prevent this problem from occurring in the future.
One technique is to ensure that the scripts are loaded properly within the HTML file. This common mistake often causes javascript to throw an `undefined` error when it doesn’t recognize certain variable in its scope. Consider using
<script>
tags at the very end of the document just before closing the
</body>
tag to give your script access to all the necessary elements and variables on the page.
A representative piece of code would look like following:
html
Another technique is to check your use of ES6 import/export statements. Modern JavaScript encourages the modularized way of coding which involves multiple individual files. In such structure, one should take care of proper exporting from one module and importing in another wherever it’s needed. If the variable XXX is declared in another component or function, ensure you export it using
export default xxx
and import within your file using
import xxx from './xxx'
.
Lastly, if you’re working within the confines of React specifically, ensure you are defining and utilizing state correctly – as this could be a potential source of ‘undefined’ errors. As Sir Tim Berners-Lee, the inventor of the World Wide Web and an authority in web standards once said, “The Web does not just connect machines, it connects people.” So is true for components in a React application. Thus, if ‘XXX’ is actually a part of the component’s local state, wrap the usage in a render method or functional component.
To further enrich your understanding about resolving ‘undefined’ errors, this [post](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Errors/Not_defined) from Mozilla Developer Network (MDN) provides robust insights.
Always remember to debug your code methodically. By applying these effective techniques, future ‘XXX is not defined’ errors could be significantly mitigated or even avoided altogether. It will help you build sophisticated projects efficiently, making your development journey smoother.Understanding that dealing with issues like ‘Xxx is not defined’ after placing script into the public/index.html in a React application can be challenging, let’s unwind this issue by taking a detailed analysis.
In the context of working with React, it’s imperative to note that the handling of scripts explicitly differs from traditional HTML based websites or applications. In most cases, attempting to insert a