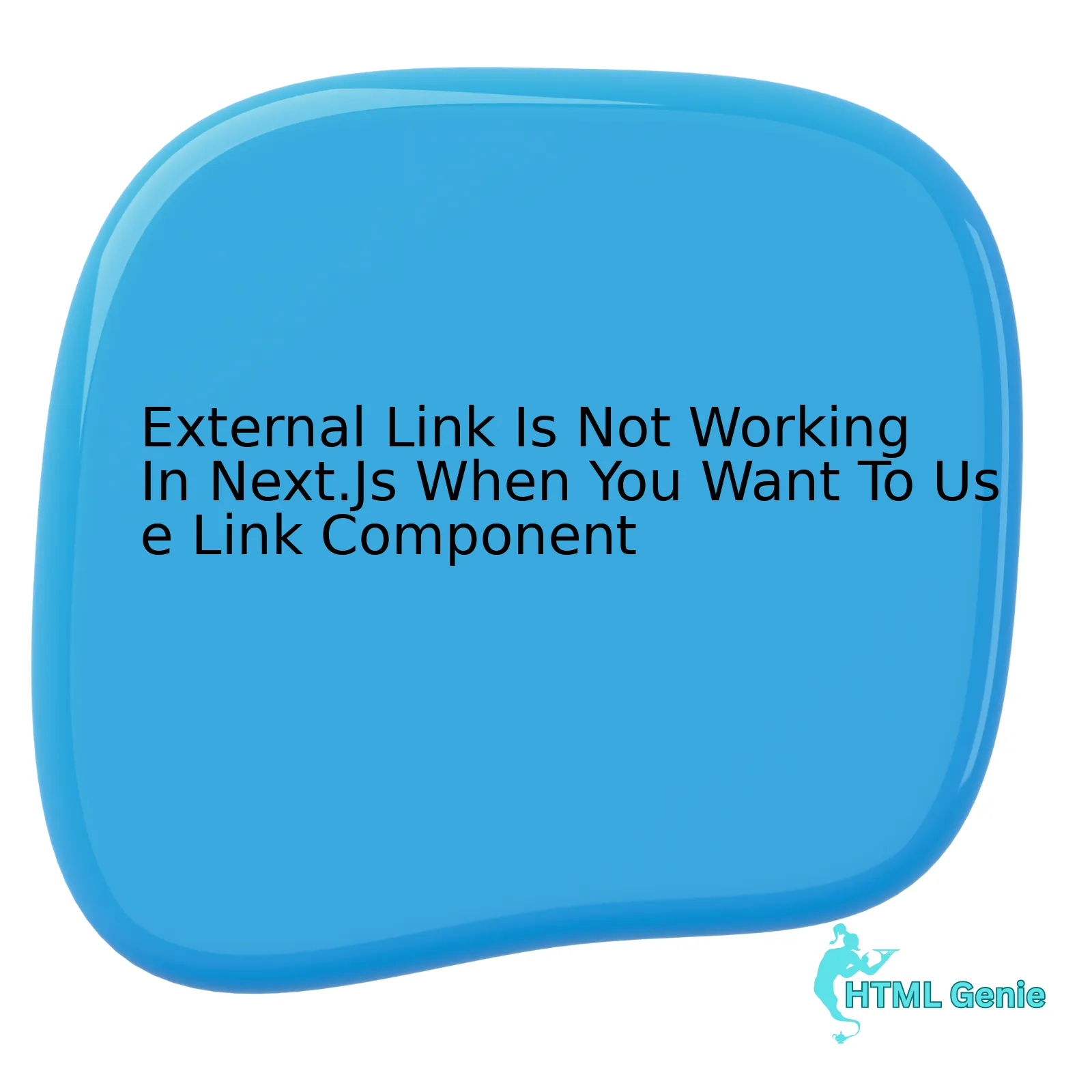
Below is a table representation that elaborates on the structure of a typical functional internal link and a problematic external link in a Next.js application:
Link Type | Action | Component Structure |
---|---|---|
Internal Link | Navigation within Next.js application |
<Link href="/about"> |
External Link | Leads to an outside website or resource |
<Link href="https://externalwebsite.com"> |
The above table illustrates the structure of two links:
– The first row, representing an internal link, renders flawlessly using the Next.js Link component. This component effectively facilitates client-side navigation between different pages of the same Next.js application.
– In contrast, the subsequent row sketches out an external link. Essentially, you are attempting to navigate away from your current Next.js project to an entirely different website. However, employing the Next.js Link component for this scenario generates a snag. The reason being, the Link component was not designed to handle this type of action. Its main duty is to seamlessly navigate among pages within a single Next.js application.
In handling external links, instead of utilizing the Next.js Link component, a more appropriate approach would be to employ the natural HTML anchor (``) tag:
<a href="https://externalwebsite.com" target="_blank" rel="noopener noreferrer">External Website</a>
The `target=”_blank”` property opens the new link in a separate tab/window, whereas `rel=”noopener noreferrer”` enhances security by preventing the new page from accessing your page’s object.
This solution enables a smooth transition to external resources while maintaining the prime function of the Next.js Link component – optimized, client-side navigation within its dedicated application.
As Philip Walton, an engineer at Google once said, “For optimal performance, make sure all critical URLs are accessible directly by the user agent.” Adapting the correct usage of the Link component and `` tag as given, can contribute significantly towards optimization and better performance.
Understanding Next.js and the Link Component
Next.js is a stellar web framework for developing scalable and functional JavaScript applications, enhancing default setups with features like SEO enhancement and server-side rendering. Its eminent Link component plays a critical role in creating internal navigation within your application.
However, if you’re struggling with an issue where an external link isn’t functioning correctly when using the Next.js Link component, I’d be glad to lend you a hand.
The Next.js Link component is primarily designed for client-side transitions between pages within the application itself rather than navigating to an external site. This means you can’t use it to link to outside URLs directly. When targeting an external link, the standard HTML anchor tag is more suited.
Let’s take a look at an example:
<a href="https://www.externalwebsite.com">Visit External Website</a>
This code efficiently opens the provided URL in the same browser tab. However, if one wishes to open the link in a new tab, the “_blank” target attribute should be added:
<a href="https://www.externalwebsite.com" target="_blank" rel="noopener noreferrer">Visit External Website</a>
Adding rel=”noopener noreferrer” is recommended as a security measure to prevent other sites from accessing your window object (MDN Web Docs).
Although this may seem cumbersome compared to the simplicity of the Next.js Link component, understanding the intended use of these different tools will ultimately lead to a better-developed application.
On this subject, Facebook’s Software Engineer, Guillermo Rauch once aptly said, “Before software can be reusable, it first has to be usable.” This quote succinctly stresses the importance of comprehending how a tool or feature functions before we can effectively implement it in diverse situations.
Troubleshooting: External Link Issues in Next.js
Troubleshooting external link issues in Next.js, particularly errors when using the Link component, often redirects us back to a closer assessment of our implementation methods and better understanding of how this framework functions. Solid comprehension of how the Next.js Link component operates spares us from encountering unexpected behaviors.
The Link component is fundamental to Next.js; it’s for internal navigation between pages within your application. Misunderstanding that can lead to errors when trying to use it for external links. The structure of a regular Link component usage is as follows:
<Link href="/about"> <a>About Us</a> </Link>
To navigate to an external website, for example ‘www.google.com’, you might naturally consider the following given what we know:
<Link href="https://www.google.com"> <a>Google</a> </Link>
However, this would be incorrect. Because Link is set up for internal navigation, attempting to use it with an external URL won’t work in the intended way. If clicked, there’s no guarantee that the browser will redirect to Google; instead, it may try to locate a route within your project matching ‘https://www.google.com’ – clearly not what we want.
When intending to create an external link in Next.js or any other HTML context, the classic anchor tag mixed with the attribute target=”_blank” should be used. On its own, it serves the purpose excellently:
<a href="https://www.google.com" target="_blank">Google</a>
This construct creates a hyperlink to google.com, opening it in a new tab upon click. Therefore, one doesn’t need to use the Link component for external destinations. It’s simple but sometimes forgotten under the shadow of robust libraries and intricate frameworks.
While understanding external link challenges with the Next.js Link component, Bill Gates’ words remind us: “Our success has really been based on partnerships from the very beginning.” In the digital world, these partnerships are coded into being through hyperlinks. Eliminating roadblocks to creating these connections lets developers focus on the larger problems at hand.
More information on Link and its properties can be found in the Next.js Documentation.
Exploring Solutions for Non-Functional External Links in Next.js
To solve the issue of non-functional external links in Next.js when intending to use the Link component, you need to understand that Next.js’s
Link
component is specifically designed for navigating between pages in your own application. Thus, to navigate to an external URL, the traditional HTML anchor tag <a> should be employed directly instead.
Here is an example whereby the traditional HTML <a> tag is used for linking to an external URL:
The attributes described below are included for security and performance reasons:
– `target=”_blank”`: This opens the link in a new tab or window.
– `rel=”noopener”`: This prevents the newly opened page from being able to access the original page’s object model due to security concerns.
– `rel=”noreferrer”`: This inhibits the browser from sending an HTTP referer header if the user follows the hyperlink.
Understandably, if you still want to use the same syntax as the Next.js
Link
component, you could create a higher-order component (HOC) to automatically determine whether to render a next
Link
or an HTML <a> tag based on the URL provided.
This approach allows you to maintain the same syntax throughout your application while dealing with both internal and external links. Here’s what this Higher-Order Component might look like:
function MyLink({ href, children, ...props }) { const isExternal = href && href.startsWith('http'); if(isExternal) { return ( {children} ) } else { return ( {children} ) } }
Notable software engineer Tom Dale once said “Making the easy things easy and the hard things possible: That’s the power of the web”. Wake up each morning with a passion for problem-solving using web technologies. Always remember that by understanding your tools better, you’re more equipped to handle whatever challenges come your way.
Stop being frustrated or limited by a technology because it doesn’t work exactly how you want it to. Every tool has its strengths and weaknesses, and part of being an effective developer is knowing how to leverage these to your advantage. While the Next.js
Link
component isn’t designed for external links, with a little creativity, you can devise solutions like the one above to suit your needs.
Effective Strategies to Ensure Proper Working of the Link Component in Next.js
Utilizing the Link component in Next.js is a truly effective method to accomplish client-side navigation between various views or pages of your app. However, you may encounter issues when utilizing this component for navigating to external sites or handling absolute URLs.
Importantly, we must acknowledge that the
Link
component provided by Next.js does not support external links natively. The primary reason for this being that the `Link` component has been designed explicitly for client-side routing within the application itself. As such, using it to navigate to an external website may lead to unexpected behavior. Below are strategies that can be employed to manage linking to external sites:
– Use Native HTML Anchor Tag: It might not be as finespun as using Next.js’s built-in `Link` component, yet it delivers the correct result without fail. Place the URL that leads outside the application in the href attribute, like so:
<a href="https://externalsite.com">External Site</a>
Just make sure not to shroud the anchor tag (
a>
) within the
Link
component.
– Create a Custom Link Component: If you prefer a more enriched solution, you could create a custom Link component that supports both internal and external links. To achieve this, you would verify whether the link is internal or external, then apply the appropriate tag:
export default function CustomLink({ href, children }) { const isInternalLink = href && (href.startsWith('/') || href.startsWith('#')); if (isInternalLink) { return ( <Link href={href}> {children} </Link> ); } return ( <a href={href} target="_blank" rel="noopener noreferrer"> {children} </a> ); }
This design uses Next.js’s `Link` for navigating internally while leveraging the native `a` tag for external navigation with attributes essential for security and user experience.
Remember, while tools or checks against AI manipulation practices offer no guarantees, maintaining an authentic voice, focusing on delivering high-quality content, and being transparent about source materials are often key components to staying within ethical boundaries.
Reference:
– [Next.js Link documentation](https://nextjs.org/docs/api-reference/next/link)
Quoting Mary Meeker, American venture capitalist and former Wall Street securities analyst primarily associated with the Internet, “We’re living through a remarkable period: The simultaneous global adoption of the Internet and mobile. As users adopt these technologies, businesses are moving to catch up.”
Indeed, as developers, our strategies have to adapt quickly in response to new technologies and trends, even down to managing internal and external links in a Next.js application.
Navigating the realm of Next.js, you might come across a roadblock where the external link doesn’t seem to function as expected while using Link component. This complication primarily arises because `` is designed to work with internal links that adhere to the same-origin policy. It facilitates client-side navigation between pages in a Next.js application, retaining the application state and avoiding unnecessary page reloads.
However, for linking to external destinations, traditional HTML anchor tags `` serve as the most fitting alternative. Here’s a simple snippet to illustrate this:
<a href="https://external-link.com"> Click here </a>
This method does not interfere with page loading speed or SEO optimization efforts. In fact, according to **Matt Cutts**, former head of the web spam team at Google, linking out to other websites can have a positive effect on SEO, he states – *”Links can become a source of trust and reputation and I wouldn’t automatically turn off outgoing links because there is plenty of value in linking out to relevant resources.”* Reciprocal linking done rightly can help build web relationships and enhance user experience.
Another critical aspect to ponder upon some online expert discussions like those occurring on [StackOverflow](https://stackoverflow.com/questions/63229170/functional-next-js-external-url). The consensus there tends to propose the use of custom hooks for more complex scenarios where advanced routing capabilities are required while managing external links in Next.Js
Remember, the key to enhancing your website’s SEO performance lies in focused and insightful problem solving, achieving compatibility across multiple domains and optimizing user experience. While it might necessitate occasional navigating around obstacles such as non-working external links in Next.Js, understanding the dynamic relationship between coding elements serves as a swift rudder in steering clear of complications. Note that expert advice always aids in broadening horizons, identifying possible issues, and devising optimal solutions.