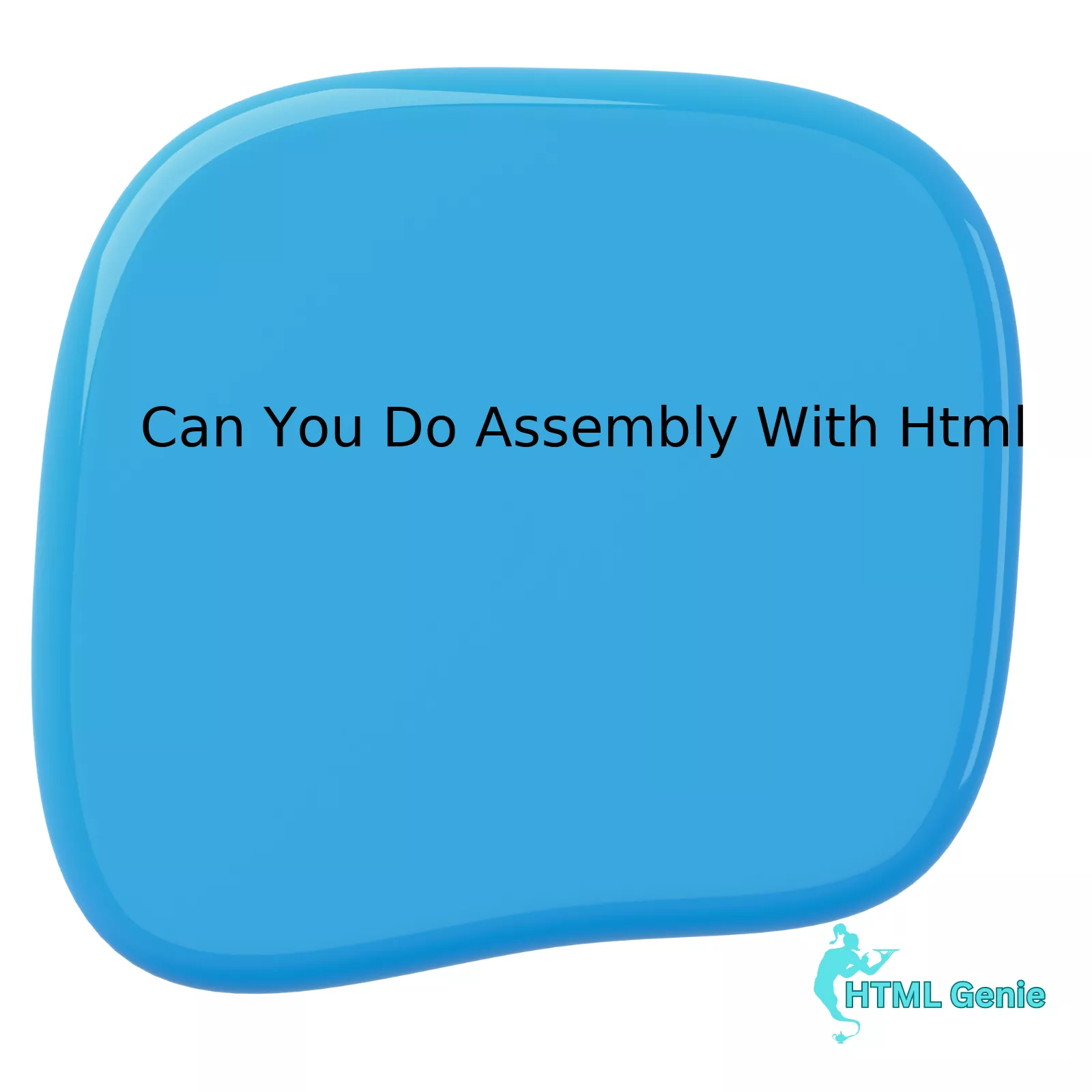
The characteristics and functionalities of both languages can be highlighted as follows:
Parameter | HTML | Assembly |
---|---|---|
Definition | A markup language used for structuring and presenting content on the World Wide Web. | A low-level programming language specific to a particular computer architecture where each statement corresponds directly to a machine instruction. |
Usage | Primarily used to design web pages, with embedded scripting languages to manage presentation and interactivity. | Generally used only in situations where high-level languages are inefficient or not desirable, such as real-time systems and very low-level hardware control. |
Code Example |
<div>Hello, World!</div> |
mov ax, num1 |
From this chart, it is apparent that assembly operates at a lower level than HTML, granting more computational control but less ease in development while HTML is mostly utilized for designing visually appealing web interfaces. Keeping this distinction in mind, it becomes clear that one simply cannot ‘do’ assembly with HTML.
The comparison might feel like trying to piece together an automobile (assembly) using a paintbrush (HTML). Each tool has its specific purpose and area of operation, and while they might indirectly contribute to the same broad objective (creation of functional, effective software), they cannot substitute for each other.
To quote Donald Knuth, “Each new user of a new system uncovers a new class of bugs.” The sharp lines dividing these two tools invoke the necessity of using them appropriately within their designated roles, for any attempt to misuse or blur the boundaries could lead to unwanted outcomes or ‘bugs’.
While it might feel tempting to imagine a seamless integration of several programming tools into one super-efficient entity, it is important to acknowledge and respect the individual strengths and limitations inherent in all programming languages, which propel the technological world forward through their own unique contributions.
For related resources please check: [Mozilla Developer Network](https://developer.mozilla.org/en-US/docs/Glossary/HTML) and [Intro to x86 Assembly Language Programming](https://www.cs.virginia.edu/~evans/cs216/guides/x86.html) for further in-depth study and research.
Exploring the Boundaries: HTML and Assembly Language
Diving into the underpinnings of HTML and assembly language helps illuminate the divergent purposes and capabilities these two languages serve in the realm of programming. Despite this, a pressing question often posed is “Can You Do Assembly With Html?” By analyzing the innate features of each language can we unravel this query.
Understanding the intrinsic nature of these two languages is crucial – both HTML and assembly are pivotal for their distinct tasks.
HTML (HyperText Markup Language) is primarily a markup language that forms the backbone of the web. It’s design revolves around structuring the content on the web, dictating how elements such as text, images, and links are presented on a webpage. To illustrate HTML, see the snippet below:
My First HTML Page Hello World!
Conversely, assembly language is a low-level programming language designed for a specific computer architecture. Unlike HTML, assembly code directly reflects the specific hardware functionalities and isn’t concerned with styling or formatting. It operates at an elemental level, enabling direct control over individual instructions sent to the CPU. Here’s an example of a simple assembly code that would print “Hello, World!” to the console:
section .data hello db 'Hello, World!',0
The crux of the question reveals itself here: Assembly language has the power to instruct the processor, while HTML enforces structure upon the content of a page. The fundamental gap between their functionalities directly implies that you cannot do assembly with HTML.
Our conclusion matches the thought expressed by Donald Knuth, who pointed out, “A program should be light, should seldom make you wait, and should not ingratiate itself. It should be capable of being used in many ways; otherwise it’s extravagant.” A true evaluation of these languages hints that using one to accomplish the task of another would be rather extravagant and inefficient in real-world applications.
While HTML and assembly language are considerably different, they contribute significantly within their respective domains, shaping our digital experience in more ways than one can perceive. [1].
Hence, even though the fusion of HTML with assembly is implausible, knowing both will undoubtedly magnify your versatile skill set as a programmer.
The Role of WebAssembly in Bridging the Gap
WebAssembly, often abbreviated as WASM, has emerged as a potent tool that bridges the technology gap between client-end web applications and system-intensive native software. It is poised to revolutionize how developers leverage resources and expand capabilities of web browsers.
To offer insight into how WebAssembly relates to HTML, it is vital to understand their symbiotic relationship within web development environments. While HTML interprets encoded semantics for web content structuring to produce user interfaces, WebAssembly functions as binary instructions to execute high-speed computation tasks on the web browser.
Using code delivered in efficient binary format, WebAssembly can manipulate the Document Object Model (DOM) similarly to JavaScript but operates at near-native speed, making it ideal for performance-critical operations such as gaming, CAD rendering, media conversion, and real-time collaborative platforms.
Yet, it must be clarified that you cannot directly use assembly code in HTML. A traditional assembler like NASM cannot interpret HTML and vice versa – HTML parsers cannot interpret low-level assembly language either.
However, through compilation to WebAssembly, languages like C, C++, and Rust allow you to use algorithms authored typically in systems programming to accomplish powerful tasks within a web browser context. When compiled to WebAssembly, these mitigate the limitations of web-based environments by executing binary code with near-native performance.
Take the following simplified workflow for transforming written C/C++ code into WebAssembly for execution in a browser:
//Step 1: Write your C/C++ program. #includevoid main(){ printf("Hello, WebAssembly\n"); } //Step 2: Compile the C/C++ program to WebAssembly using Emscripten. emcc hello.c -s WASM=1 -o hello.html //Step 3: Load and execute the WebAssembly module in an HTML file. <script> fetch('hello.wasm') //fetch the wasm file .then(response => response.arrayBuffer()) .then(bytes => WebAssembly.instantiate(bytes, {})) //turn bytes into a runnable wasm module .then(result => result.instance.exports.main()); //execute function from the module </script>
The above process demonstrates how languages commonly associated with systems programming can effectively bridge the performance gap in web environments, thanks to the WebAssembly ecosystem. The result is dynamic and efficient web applications that remain lightweight, yet powerful.
As Steve Jobs put it, “Design is not just what it looks like and feels like. Design is how it works.” This dictum beautifully encapsulates the emergence of WebAssembly in the world of web design and development, steering us towards creating more robust, responsive, and interactive web applications.
Comparing Features: Assembly vs HTML Coding Techniques
Understandably, one might consider comparing Assembly and HTML coding techniques as akin to contrasting the language of mathematics with that of poetry. They serve inherently different purposes, in fundamentally different domains. Assembly is a low-level programming language closely related to the machine code within your device’s hardware. HTML (Hyper Text Markup Language), on the other hand, is a higher-level markup language intended specifically for designing web pages.
Both present unique characteristics, advantages, and challenges. Let’s probe further into these features:
Assembly HTML ◾ Being a low-level programming language, it runs directly on your personal computer's processor.
◾ Facilitates better control over system resources enabling you to write highly efficient programs.
◾ It can interact with hardware, execute I/O operations at a granular level.
◾ A higher-level markup language primarily for designing web pages.
◾ HTML is simpler to learn, read, and write, presenting a clean structure for site content.
◾ User-interface and UX driven focusing on browsers' interpretation and display.
In response to your query ‘Can You Do Assembly With HTML?’—the answer is no. HTML and Assembly perform entirely distinct roles. HTML does not have capabilities to control system resources or conduct hardware interaction like Assembly, nor are they designed to do so. HTML applications run within web browsers which act as an abstraction layer between the application and the actual operating system and hardware. This vital separation ensures better security, stability, and interoperability across various devices and platforms.
Here is the man himself, Tim Berners-Lee founding father of the World Wide Web and creator of HTML, explaining its purpose: “Anyone who has lost track of time when using a computer knows the propensity to dream, the urge to make dreams come true and the tendency to miss lunch.”
As should be evident, Assembly and HTML aren’t meant to replace each other; instead, they supplement one another toward creating flexible, dynamic, and robust systems, elegantly blending efficiency with expressivity—a testament to the aphorism that diverse tools allow for distinctive tasks.
Practical Applications of Using HTML with WebAssembly
WebAssembly (WASM) is a binary instruction format that functions as a virtual machine, operating at near-native speed by using common hardware capabilities that are available on various platforms. While HTML is predominantly used for creating the structure and content of web pages, WebAssembly brings about new possibilities when it comes to executing performance-critical tasks directly from within your browser.
The question “Can You Do Assembly With HTML?” might require a bit of clarification. Typically, ‘assembly’ refers to assembly language, which is low-level programming done very close to the computer’s machine code. HTML, on the other hand, is a markup language primarily utilized for structuring content on the Web. Consequently, conducting ‘assembly’ with HTML won’t be possible; they serve completely different purposes in the context of web development.
However, if we’re discussing the possibility of utilizing WebAssembly (a different concept than classic ‘assembly’) along with HTML, then indeed, this becomes a fascinating and practically applicable proposition.
Web Technology | Primary Function |
---|---|
HTML |
Creating the structure and content of web pages. |
WebAssembly (WASM) |
Running high-performance applications in a browser setting. |
When considering functional examples of merging HTML with WASM, consider an application that requires condensed computations – such as a video game or a complex visualization. In such scenarios, WebAssembly can perform critical functions rapidly while the rest of the interface (buttons, game intro screens, etc.) can be composed utilising regular HTML and CSS.
Here is an example of how you can use WebAssembly with HTML:
html
In this specific sample, our HTML page fetches a WASM file, compiles and instantiates it, and ultimately uses the exported function from our WASM module to process some input value taken from an HTML form.
As Alan Kay once articulated: “People who are really serious about software should make their own hardware.” Similarly, developers who are determined to extract the most from their web applications would find it worthwhile exploring what WebAssembly has to offer in tandem with traditional web technologies like HTML.
References:
1. [MDN Web Docs: WebAssembly](https://developer.mozilla.org/en-US/docs/WebAssembly)
2. [W3C: HTML A vocabulary and associated APIs for HTML and XHTML](https://www.w3.org/TR/html52/)Developing a profound understandings on whether one can do assembly programming with HTML takes a detailed analysis on the fundamental nature of each. For this purpose, let’s delve into their individual characteristics and purpose.
“Hyper Text Markup Language, or HTML, is the standard markup language for documents designed to be displayed in a web browser.”
– W3 Schools
HTML basically facilitates the structuring of the web content by defining its components and how they’re presented on screen. With HTML, you manipulate text, images, links and even multimedia within any web page.
HTML Components
Feature | Description |
---|---|
Text | HTML manages all the text content of the webpage. |
Images | HTML allows embedding images in the webpage. |
Links | HTML facilitates navigation between webpages through hyperlinks. |
Multimedia | HTML enables the use of audio and video elements on a webpage. |
On the other hand, Assembly language is a low-level programming language where there is a very strong correspondence between its instructions and the architecture’s machine code instructions. It is used predominantly for direct hardware manipulation, accessing specialized processor instructions, or addressing critical performance issues. Here provides a simple example of assembly language:
MOV R0, #2 LDR R1, =#3 STR R2, [R1]
However, linking these two vastly different languages brings light to our main query — is it possible to do assembly programming with HTML? Succinctly put, it is not possible. Although both are languages in their respective fields, they serve fundamentally different purposes. In essence, HTML is for formatting the web pages’ content, whereas Assembly deals with the minute particulars of a computer architecture by means of direct commands. Hence, intertwining them wouldn’t serve functional coherence.
Although from a holistic perspective this might seem limiting, there is an avenue that brings us closer to combining the concepts of HTML and Assembly. That is WebAssembly. As per Mozilla Development Network (MDN), “WebAssembly is a new type of code that can be run in modern browsers — it is a low-level binary format that has a compact binary representation which executes at native speed.”
By adding layers of complexity, in-depth knowledge, one can navigate through a contemporary web environment. And who knows what further innovations will make possible in the spheres of coding and web development technologies, opening possibilities we currently cannot fathom.
Read more about WebAssembly here