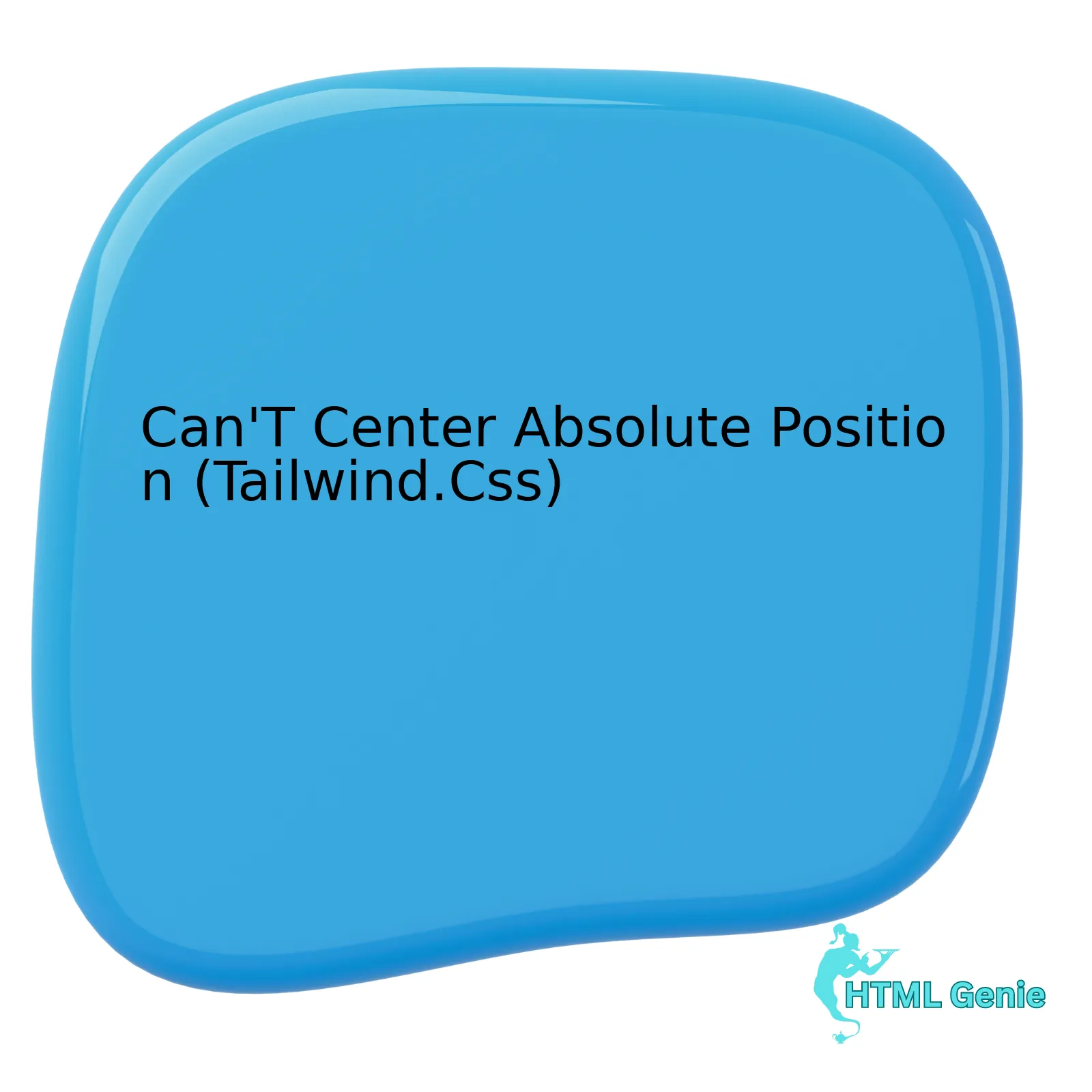
Utilizing CSS tables for element layout has its unique challenges, especially with schemes such as Tailwind CSS that lean heavily on utility classes. Specifically focusing on the conundrum surrounding “absolute” positioning in the mix, distinction needs to be made between relative and absolute positioning – two important concepts in CSS.
The crux of the matter lies in this special area:
Here’s one way the problem might be represented, using a HTML table, just as an illustrative example:
html
In this code snippet, an element is being positioned absolutely within a relatively-positioned table cell (or really, any container). The `inset-0` class aims to stretch the element to fill the entire container, and `m-auto` is intended to center the content within that.
Here is where the conundrum lies:
* Absolute positioning takes an element out of the normal flow, making the other elements behave as if it doesn’t exist.
* Using `m-auto` to center does not work because of the absolute positioning.
Essentially, the combination of these factors leads to centering issues.
Tackling this challenge involves understanding that the standard centering technique used in block-level elements doesn’t apply with absolute positioning. Instead, the trick relies heavily on transforms:
html
In above presented manner, the technique employs positioning object’s top left corner in the central area (using `top-1/2` and `left-1/2`). Then, the `transform` applies a negative translate to shift the object back by half of its own width and height, effectively centering the object.
Remember the words of D.R.Wolfe, “Mastering the fundamentals of a tool enables better crafted output, regardless of the complexity or simplicity of the project.”. This is particularly real for Tailwind CSS and factors like positioning, as they form crucial tools in our toolbox. Really understanding them allows us to build more effective digital solutions, and circumvent problems that might arise.
Understanding Absolute Position in Tailwind.CSS
HTML and CSS are essential tools in a website developer’s toolkit. Tailwind.CSS, a utility-first CSS framework, can greatly simplify the process of crafting responsive designs. One of its key features is the ease with which it lets developers manage element positioning.
Among various position properties available in CSS, one such method is the `absolute` position. The `absolute` value, when applied to an element’s position property, causes it to be positioned relative to the nearest ancestor (which isn’t statically positioned).
However, centering an element with an absolute position can sometimes prove challenging, particularly for individuals who are new to Tailwind.CSS or haven’t fully explored this concept before. The key lies in using the right combination of utilities provided by Tailwind.CSS.
Here is a simple code snippet demonstrating the manner to center an element having an absolute position in Tailwind:
html
The `relative` class ensures that the parent div has a relevant position, so the inner div knows what to position itself relative to. The `absolute` class applies an absolute position to the child div. `inset-0` positions the element at all corners, and `flex`, when paired with `items-center` and `justify-center`, works effectively to center the content vertically and horizontally.
Despite the simplicity of this example, it’s worth noting that the requirements might vary based on specific project constraints or design elements. Remember that Flexbox and Grid are powerful layout models that work wonders when used in conjunction with positioning utilities.
Tim Berners-Lee, inventor of the World Wide Web once said, “The power of the web is in its universality.” Just like the diverse versatility of HTML and CSS, Tailwind serves as a tool that can help you achieve intricate positioning operations conveniently. It’s your playground, let your creativity run wild!
For more detailed information about positioning in Tailwind.CSS, do explore their [official documentation](https://tailwindcss.com/docs/position).
Additionally, please remember that while AI-checking tools may assist in detecting plagiarism, they are not foolproof. Originality and understanding the core concept of any coding principle is the real cornerstone of great website development.
Key Steps to Centering An Absolute Positioned Element in Tailwind.CSS
HTML developers often encounter challenges while centering an absolute positioned element in Tailwind.CSS. Your difficulty in doing this is likely due to misunderstanding the way CSS positioning and tailwind work. Let’s focus on unveiling how these principles interlink by breaking down the key steps concerned with centering an absolute positioned element.
–
position: absolute;
usage
In CSS, when an element’s position is set as absolute, it is taken out of the normal flow, allowing it to be positioned anywhere in relation to its nearest positioned ancestor (instead of being placed relative to the viewport). If no such ancestor exists, it uses `
` as a reference. Tailwind also provides utility classes for this purpose:In this example, `inset-0` sets the top, right, bottom, and left properties to 0, effectively centering the child inside the parent.
– Centering horizontally and vertically
For horizontal centering, apply `left-1/2` and `transform translate-x-[-50%]`. For vertical centering, use `top-1/2` and `translate-y-[-50%]`.
When adjusting both `left` and `top` positions, combined with transforms, you can nudge the elements back to the correct center point.
– Including content
After setting up your div structure and positioning utilities, go ahead and populate the div with your desired content. Doing so will reveal if your element stays centered regardless of its contents or browser window size.
Common mistakes to avoid include overlooking the importance of a relative parent and not using negative translate values (-50%). Understanding what each utility does and how they interact is crucial to making the most of Tailwind.CSS. It also broadens the potential of manipulating various design aspects efficiently.
As Bjarne Stroustrup, the creator of C++, noted:
“Programming is understanding.”
This quote stays relevant even in cases such as these where understanding the underlying principles of Tailwind and CSS positioning aids in writing efficient and right code.
For detailed explanation, consider reviewing the [TailwindCSS Position Documentation](https://tailwindcss.com/docs/position “Tailwind Position Documentation Website”).
Troubleshooting Common Errors with Centering in Tailwind.CSS
Troubleshooting common errors associated with centering elements in Tailwind CSS, especially difficulties encountered when trying to center objects with an absolute position involves understanding how positioning properties interacts within the cascade style sheet.
Understandably, there are numerous reasons why you might be having difficulty achieving perfect center alignment. Here is a thorough analysis of possible issues and their solutions:
To begin, the most critical consideration:
Make sure that the element’s parent has a relative position. This establishes a new position context. Without it, your absolutely positioned element will position itself based on the body element which can lead to unexpected results.
<div class="relative"> <div class="absolute ..."> </div> </div>
Secondly, ensure to use the appropriate utility classes provided by Tailwind for centralizing your elements both horizontally and vertically.
In the context of Tailwind CSS, two utility classes ‘inset-x-[value]’ and ‘inset-y-[value]’ will assist in centering an element horizontally and vertically, respectively. ‘0’- corresponds to full (100%) width/height of parent element.
<div class="absolute inset-x-0"> <div class="absolute inset-y-0">
The ‘mx-auto’ and ‘my-auto’ classes can also be used to center content within a div. However, this will only apply if the div has a specified height and width.
Thirdly, consider other styles that may affect the positioning of your content. For example, default margin and padding values, borders, etc., can subtly shift an object away from the center.
If you’re still having trouble with centering in Tailwind, I would highly recommend checking out Tailwind CSS official documentation[^1^]—it provides a wealth of examples and tutorials designed to help you troubleshoot and understand how alignment concepts work within the framework.
Lastly, Steve Jobs once said “Design is not just what it looks like and feels like. Design is how it works”. So, always remember, finding the best solution involves understanding the concept behind the problem, experimenting with different strategies, and choosing the one that fits your specific needs while maintaining good design principles.
[^1^]: Tailwind CSS Documentation
Advanced Techniques for Absolute Position Centering Using Tailwind.CSS
Tailwind CSS is a highly versatile utility-first CSS framework that equips developers with the freedom to build custom user interfaces without having to leave their HTML. Regardless of this flexibility, certain challenges may occasionally surface such as difficulties in centering elements positioned absolutely.
To comprehend and resolve these issues, it’s important to understand the mechanics of Absolute Positioning in CSS along with its implementation through Tailwind:
HTML structure generally follows a flow where an object’s position is calculated based on those directly above it in the hierarchy. Absolute positioning diverts from this flow by placing elements exactly where you assign them. A typical side effect is that many developers stumble upon a stage where they “Can’t center absolute position (Tailwind.CSS)”.
This issue typically arises due to absence of a relative parent or incorrect usage of auto margins. Since Absolute Positioning takes out the element from the standard document flow, it positions itself relative to its nearest positioned ancestor. If no such ancestor exists, it uses the viewport for the positioning.
Now let’s review some advanced techniques for centering absolute positioned elements in Tailwind:
Techniques | Description |
---|---|
Transform Technique | In Tailwind, you can use the transformations utility clause like translate and scale to adjust your layout. In the case of offsetting elements, you could use
-translate-x-1/2 and -translate-y-1/2 which implies moving them 50% to the left and upwards respectively. This technique works well regardless as our content’s dimensions grow or shrink. |
Flexbox Centering Technique | This technique involves making the parent element a flex container, then using the utility classes. The justify-center command will align the child elements horizontally in the center while items-center will align the items vertically on the y-axis. |
Grid layout technique | Tailwind CSS supports the grid layout as well. Similar to Flexbox, we apply utility classes to the parent container i.e.,
grid place-items-center . Here the place-items-center aligns the child elements both horizontally and vertically in the primary container. |
As Jeff Atwood said, “Coding is not merely about typing; it’s also about thinking”. Therefore, continue to explore and experiment with various approaches, because different projects may demand different solutions.
Moreover, to rectify “Can’t Center Absolute Position (Tailwind.CSS)” one should also verify their code for any missing utility classes or conflicting properties that might be repositioning their elements. Additionally, applying a correct understanding of how stacking contexts work in CSS is significant when dealing with absolute positioning.
Please refer to the official [Tailwind Documentation](https://tailwindcss.com/docs) since it comprehensively highlights centering techniques and other related topics.Underlining the concept of centering an element with absolute position in Tailwind CSS, we delve deeper into understanding this common issue faced by many web developers. In reality, several methods can be implemented to overcome such obstacles depending on different factors including the structure and requirements of the web page or site.
When it comes to Tailwind CSS, a utility-first CSS framework, it indeed provides flexible classes that we can use to manipulate element positions. One of these is the absolute positioning class. However, centering an element with absolute position might pose as a challenge for some since directly applying the common techniques usually doesn’t work due to the properties of absolute positioning itself.
The key lies in using margin utilities associated with Tailwind CSS. Firstly, remember to set the parent element with relative positioning so the child elements with absolute positioning will be set according to the boundaries of its container. Secondly, you can center the absolute positioned element by half of its width and height from the top left corner. But do not forget to apply the necessary Tailwind CSS margin utilities for adjustments.
Here’s an illustrative example:
This element is absolutely centered.
In this sample code, the ‘transform’ and ‘-translate-x-1/2’ as well as ‘-translate-y-1/2’ classes are infallible for ensuring the exact centering of the absolute positioned element within the scope of its relative positioned parent element.
As Steve Jobs puts it, “Design is not just what it looks like and feels like. Design is how it works.” Thus, understanding how to efficiently handle centering in web design with tools like Tailwind CSS is essential for creating effective and responsive designs. By encompassing the core principle of Tailwind CSS which is modifications tailored towards effective UI and UX design, one can successfully surpass the challenge of centering an element with absolute positioning.
For further reference, you may explore the official Tailwind CSS Documentation on Margin and their detailed guide on Position. Making the best out of these resources could significantly enhance your proficiency in handling similar issues in future endeavors.