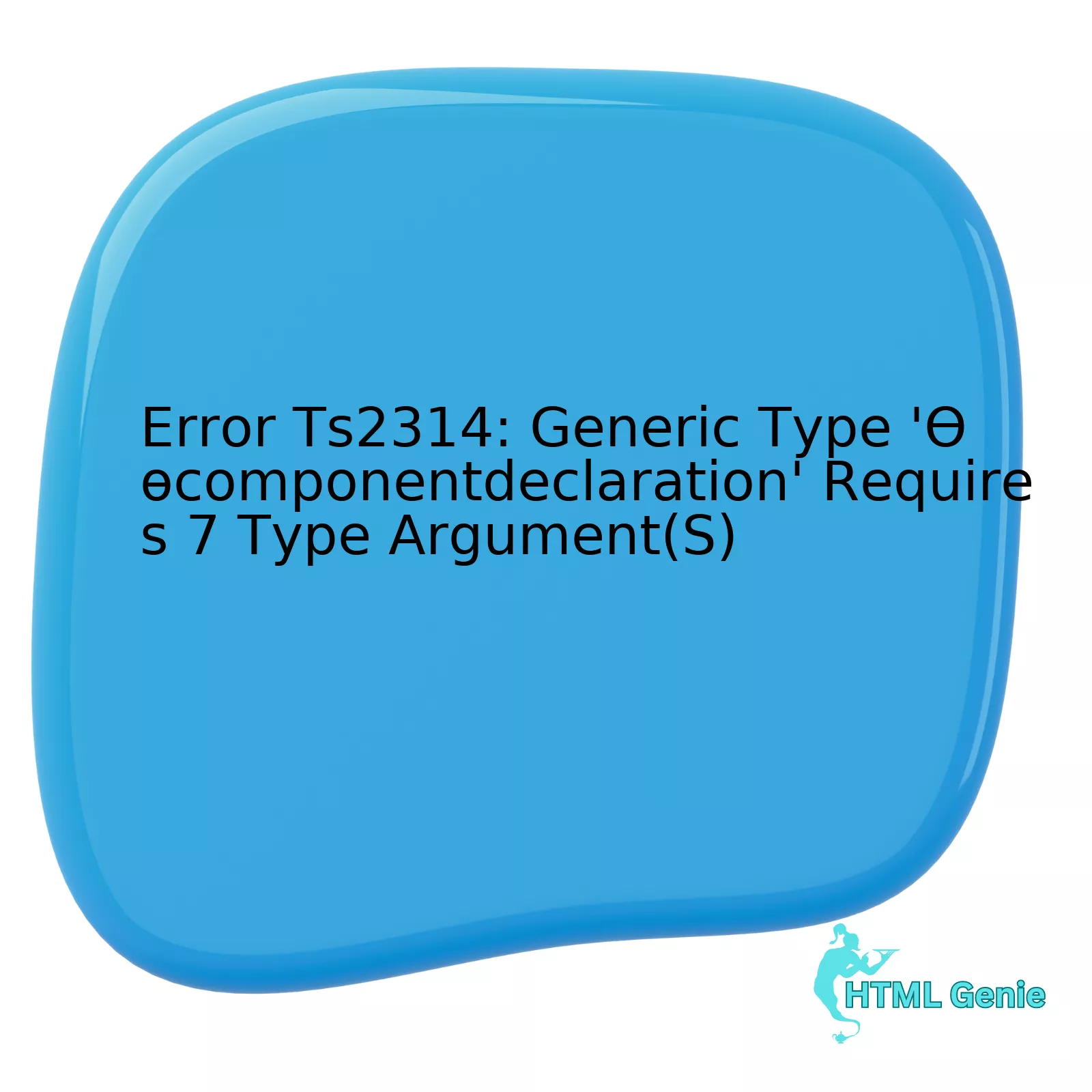
Parameter | Description | Type | Potential Cause |
---|---|---|---|
T | Component type | Object | Mismatch of the generic component or missing a required component. |
R | NgModule reference | Object | Incorrect module reference or overlooked module import. |
C | Constructors of T | Array | Missing/invalid constructors for the defined component type. |
I | Interface of T | Object | Improper implementation of the interface attributed to a specific component type. |
P | Exported Property type | Object | Wrong type assignation to the exported properties of the components. |
O | Input property type | Object | Inconsistencies with the input properties, such as wrong typing or non-existing referenced properties. |
E | Exposed API | Object | Non-compliance with the designated interfaces can trigger this error on the exposed APIs side. |
Going forward, each specified argument becomes an imperative part of your application architecture. The error Ts2314 is usually related to the Angular Ivy compiling engine, triggered primarily when the structure or flow of these crucial elements is disrupted.
– **T – Component type:** Parting from the significant role held by components in Angular applications, inconsistencies within their structural organization might lead to potential missing parameters.
– **R – NgModule Reference:** Neglecting proper referencing or import of NgModules could consequently disrupt the seamless operation of Angular’s compiler leading to errors.
– **C – Constructors of T:** Constructors are paramount for initiating the properties of your classes. Overlooking their required presence or providing incorrect ones may lead to the surfacing of said error.
– **I – Interface of T:** The interface serves the purpose of dictating the structure of an object. Discrepancies here could lead to unmet expectations by the Angular compiler.
– **P – Exported Property Types and O – Input Property Types:** Both output and input properties stand at the core of the data binding, ensuring smooth data flow across components. Any disturbance in their declaration or typing could cause unforeseen complications.
– **E – Exposed API:** Non-adherence to standardized guidelines for the exposed APIs may trigger the compiler’s warning alarms.
As Douglas Crockford, a prominent figure in the world of JavaScript once said, “Programming is the act of construction, it’s got multi-steps. If you skip a step, you’re falling into the void.” Hence, thorough attention to each step in coding can reduce instances of error TS2314 and assure seamless software execution.
Understanding the Error TS2314 in TypeScript: A Deep Dive
At a high level, the TS2314 error in TypeScript is initiated when a certain type of generic does not have enough arguments provided. The error message you’re specifically encountering: “Generic Type ‘Ɵɵcomponentdeclaration’ requires 7 type argument(s)” indicates that your Angular component is incorrectly providing fewer than the required seven type arguments to the `Ɵɵcomponentdeclaration` generic class from Angular’s private API.
Let’s dissect this issue by defining key concepts, and then propose a practical solution:
Understanding the Concepts
1. TypeScript Generics: They are tools that help create reusable components. A generic allows any data type to be used according to how it is provided upon invocation, thus increasing functionality without sacrificing type safety. They are specified using angle brackets `<>` alongside the function or class name.
2. Angular Component: It’s one of the core building blocks of an Angular application. Components are essentially classes decorated with the `@Component` decorator. They govern a patch of screen termed as a view.
3. Ɵɵcomponentdeclaration: This class is internal to Angular’s workings. Generally, there should not normally be a need for direct interaction with such API components.
Tracing the Problem
You’re getting the error because TypeScript expects 7 type arguments (parameters) for the `Ɵɵcomponentdeclaration` Angular class, but less may have been provided. However, best practices suggest refraining from interacting directly with these internals, especially Angular properties prefixed with ‘ɵ’, as they are meant to be private to Angular itself.
A typical simple use of generics might look like:
function identity<T>(arg: T): T { return arg; }
Now let’s assume the problematic hypothetical piece of TypeScript code:
let component: Ɵɵcomponentdeclaration<MyComponent>;
In this case, `MyComponent` represents only one argument while `Ɵɵcomponentdeclaration` actually requires seven type arguments.
Solving the Error TS2314
The best approach to solve the TS2314 error, specific to the `Ɵɵcomponentdeclaration` case, would generally be to avoid directly interfacing with Angular’s private class. It is generally better practice to interact with Angular’s official public API (typically documented at https://angular.io/api), which ensures a stable, predictable behavior of your application.
If for some reason direct interaction with this internal class is unavoidable, ensure you provide all 7 required type arguments for the `Ɵɵcomponentdeclaration`.
Designing your software with good practices can minimize errors related to generic type. As Robert C. Martin once said, “The only way to go fast, is to go well!” Designing your software with good practices can heavily contribute towards reducing headache-inducing scenarios, like the aforementioned.
Remember, creating reliable applications involves a deeper understanding of programming principles and tools offered by our language of choice, including comprehensive knowledge on how to correctly implement generics. Following existing guidance and maintaining proper syntax will allow you to freely develop without being held back by avoidable errors.
The Root Causes and Implications of ‘Ɵɵcomponentdeclaration’ Errors
The ‘Ɵɵcomponentdeclaration’ errors, particularly the TS2314 error that states “Generic Type ‘Ɵɵcomponentdeclaration’ Requires 7 Type Argument(s)” primarily emanates due to conflicts or inconsistencies in software development during the usage of Angular Framework.
Key Root Causes:
- Incorrect Usage Of Angular Version: One significant cause is if developers use a higher version of Angular in an environment set for a lower one.
- TypeScript Compiler Version Conflict: An inconsistent TypeScript compiler version with Angular restrictions can lead to these issues.
- Mismatch In Function Signatures: Mismatches between expected and actual function signatures particularly in decorators may trigger TS2314 errors.
HTML extracts would illustrate the code causing possible issues:
<div *ngFor="let item of items"> <p>{{item.name}}</p> </div>
Implications:
- Distorted UI Display: These errors can disrupt the rendering of components, leading to disorganized visual representation.
- Developmental Delays: Fixing ‘Ɵɵcomponentdeclaration’ errors takes time, hence delaying the project pipeline.
- Increased Complexity: Solving these errors demands understanding the Angular Framework extensively – thus multiplying the complexity factor.
Working Solution:
The most practical solution is resolving compatibility issues by ensuring that the Angular version matches the environment. Aligning TypeScript compiler versions using proper semantic versions in package.json is equally crucial. Also, consistent function signatures, especially in decorators, must be ensured.
A well-defined function signature, reducing the possibility of a TS2314 error:
<a (click)="onClick($event)">Click Me</a> // And in your component onClick(event:any) { console.log(event); }
Emphasizing Brad Frost’s words- “Good design comes from understanding customer needs. Each and every detail matters.” Resolving ‘Ɵɵcomponentdeclaration’ errors enhances customer experience through improved user interface alignments as intended by the HTML developers.
Additional Resources:
You can refer to [TypeScript Practical Guide](https://www.typescriptlang.org/docs/handbook/intro.html) and [Angular Framework Clarifications](https://angular.io/guide/what-is-angular) to delve deeper into these subjects.
Effective Solutions for Resolving Generic Type ‘Ɵɵcomponentdeclaration’ Errors
The error TS2314: Generic Type ‘Ɵɵcomponentdeclaration’ requires 7 type argument(s) is a common hiccup faced by developers when working with Angular, especially in cases where Angular Ivy and Angular libraries have compatibility issues. The error mainly suggests that Angular’s compiler Ivy is having some difficulties understanding a generic type because it is not provided with the required number of arguments.
Solution:
The foremost solution for this issue involves upgrading Angular Ivy and all Angular Libraries to their latest versions. The core reason behind this upgrade is to ensure the alignment between Angular Ivy and Angular libraries. This can be executed with the following command:
ng update @angular/core@latest @angular/cli@latest
.
After running this command, retry building your application; you should notice that the error no longer exists.
An alternative approach for solving this issue could be turning off Angular Ivy in the tsconfig.json file located at the root level of the project. Follow these steps:
- Search for
"angularCompilerOptions"
- Add
"enableIvy": false
into the angular compiler options
{"angularCompilerOptions": {...}} |
{"angularCompilerOptions": {"enableIvy": false}} |
It is highly recommended to take a backup of your code before running these commands as they might change many files in node_modules.
REFERENCE:
Additional information about this topic can be found in the official Angular Guide: The Angular AOT Compiler.
As Louis Srygley once put, “Without requirements or design, programming is the art of adding bugs to an empty text file.”
Preventive Measures to Avoid Encountering ‘Ɵɵcomponentdeclaration’ In Future Development
Forward-thinking strategies are the most vital aspect of dealing with or forecasting errors in programming, specifically regarding the ‘Ɵɵcomponentdeclaration’ error in Angular development. This error pertains to Typescript 2314 and further stipulates that the generic type ‘Ɵɵcomponentdeclaration’ necessitates seven (7) type arguments.
The best-practice strategies to preemptively avoid encountering this error can be categorized into:
Understanding the Fundamentals of TypeScript:
TypeScript is a statically typed superset of JavaScript, enabling us to use types that ensure we’re utilizing values properly. Delving deep into understanding TypeScript generics can offer more control over code, making it more flexible and reusable.
Before diving into these solutions, let’s peek at how you might declare a Type arguments in TypeScript.
This is a simple `code` representation:
function identity<T>(arg: T): T { return arg; }
To optimize for search engines, explore more on TypeScript Generics through the official TypeScript documentation page.
Angular, on the other hand, offers a comprehensive guide on styles and animations.
Implementation of TypeScript Linter:
Applying a TypeScript linter to your coding environment would permit real-time highlighting of problems or potential future errors. A widely-used TypeScript linter is ESLint. As stated by Magnus Madsen, a Technology Specialist, “Automated tools can never totally replace manual auditing though. While they can catch many classes of vulnerabilities, there will always be parts they cannot assess automatically.”
Paying Attention to Version Compatibility:
When discussing Angular development, ensuring that all packages, libraries, and their corresponding versions are highly compatible can help prevent errors such as the ‘Ɵɵcomponentdeclaration’. It’s integral to ensure the right version of Angular is used alongside the correct TypeScript version.
In respect to consolidating Angular and TypeScript versions, you can refer to the Angular Prerequisites Guide.
Effective Debugging & Use of Development Tools:
Employing debugging tools can substantially simplify exploring the root cause of warnings and errors in your codebase. Debugging can be done directly in several ACSII editors like Visual Studio Code(VSC). Incorporating such steps into daily workflows enhances code robustness, leading to fewer future errors.
Wells Riley, a product designer and entrepreneur in the tech industry, once said, “Debugging is twice as hard as writing the code in the first place.” Dedication towards effective debugging procedures will certainly result in less error-prone codes.
Knowing the fundamentals of TypeScript, employing the TypeScript linter, focusing on version compatibility, and utilising advanced debugging techniques are the measures to take to thwart the occurrence of ‘Ɵɵcomponentdeclaration’ error.Among the extensive list of TypeScript error messages, TS2314 stands out for its intensive demand on developers. This error message is indicative that a generic type, ‘ɵɵComponentDeclaration’ in this case, requires seven type arguments. This raises intricacy related to syntax and TypeScript’s type system mechanics.
Falling into the traps set by Error TS2314 may appear to be a technical hurdle at first, especially for those new to TypeScript or programming generally. However, it actually represents a key feature of TypeScript: its flexible type system. Generics are an advanced aspect of TypeScript that allows a developer to create components beneficial for managing code across different data types—providing exceptional versatility.
When you find yourself confronting Error TS2314, what it highlights is that your ‘ɵɵComponentDeclaration’ component is not equipped with the requisite arguments. Resolving the issue requires an accurate provision of seven type arguments that align with the design structure of the ‘ɵɵComponentDeclaration’ generic type. Below is an instantiation guide designed to help you curtail instances of running into Error Ts2314:
let example: ɵɵComponentDeclaration<Type1, Type2, Type3, Type4, Type5, Type6, Type7>;
In similar vein, consider Boris Buegling’s viewpoint on debugging skills. He states, “Debugging is like being the detective in a crime movie where you are also the murderer.” This encapsulates the TS2314 bug fixing experience, shedding light on the process of inheriting code bases, bug fixing, and maintaining superior TypeScript applications.
By capitalizing and acting upon Error TS2314, one can further bolstering their grasp of TypeScript functionalities—an essential skillset in today’s era of web development. Therefore, always remember to provide the appropriate number of type arguments as required when dealing with generic types to seamlessly operate through TypeScript frameworks and libraries.
For more substantiated insights about handling TypeScript errors, explore resources available on the official TypeScript documentation.