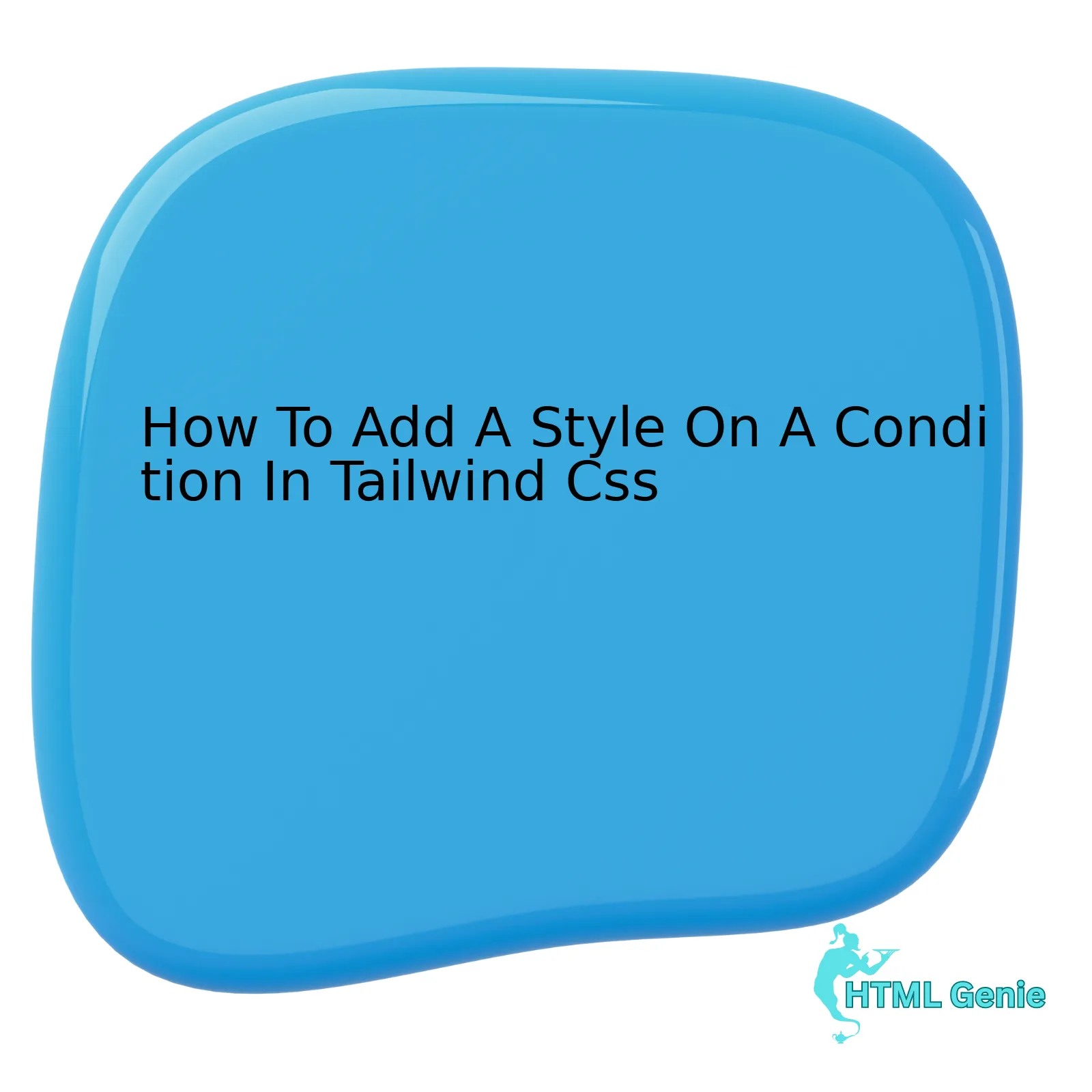
Consider this explanatory table:
Variant | Description |
---|---|
responsive |
This variant is used to apply specific styles at different screen sizes. For instance, the class
md:text-base applies the text-base utility at medium screen sizes and above. |
hover |
The hover variant is applied when the mouse pointer hovers over an element. For example,
hover:bg-blue-500 changes the background color to blue when hovered over. |
focus |
This variant is applied when an element has input focus. Like,
focus:border-red-500 applies a red border to an input field when it is focused. |
Each row of this illustrative guide pertains to a unique variant utility, elucidating its function within the context of conditional styling in Tailwind CSS. The ‘responsive’ variant renders diverse styles according to screen size. Meanwhile, both ‘hover’ and ‘focus’ oversee stylistic changes contingent upon user interaction – the former reacts to a cursor hovering over an element, while the latter responds to an element acquiring focus.
Click here to delve into the comprehensive documentation on these variant utilities and others available through Tailwind CSS. Mastering their use is fundamental to creating robust, interactive, and responsive designs with this powerful utility-first CSS framework.
As expressed by Eric A. Meyer, renowned web design consultant and author, “CSS is the language we use to build the Web, in senses both philosophical and practical. It’s much, much more than colors and fonts.”
Applying Conditional Styling in Tailwind CSS: A Comprehensive Guide
Applying conditional styling in Tailwind CSS, a utility-first framework, offers the flexibility to add styles based on specific conditions. This helps create dynamic and adaptive interfaces that can change based on user interaction or dependent on certain rules set by developers.
Understanding the Basics of Tailwind CSS
Tailwind CSS works differently compared to traditional CSS frameworks by focusing primarily on utility classes instead of predefined components. It provides low-level utility classes that let you build completely custom designs.
The Concept of Conditional Styling
Conditional styling means applying different styles depending upon specific conditions. For example, changing the color of a button when it’s clicked or hovered over, or making an element visible only when a certain condition is met.
Adding a Style on a Condition in Tailwind CSS
In Tailwind CSS, you can add style on a condition using directives like
@apply
@apply
To achieve conditional styles, you’ll typically need to use JavaScript to dynamically add or remove classes based on certain conditions (e.g., user interactions).
Action | JavaScript Functionality | Tailwind CSS Class |
---|---|---|
Button Clicked | Add/Remove class on click | bg-blue-500 (or any other Tailwind CSS class) |
Check if Element Visible | Add/Remove class based on check | hidden: Add this class to hide, remove to show |
A brief example of how you might toggle a class in JavaScript:
Hover, Focus, & Other States is a great reference to know more about handling states in Tailwind CSS.As Kent Beck once said, “Make it work, make it right, make it fast." Therefore, understanding the basics and getting it to work is the first step. Optimization and adaptation should follow naturally afterwards.
Best Practices
When using utilities for stylistic variations driven by state changes (like :hover), it's important not to mix them with structural styles as it can make your code less predictable and harder to maintain.
]]>Leveraging JavaScript for Tailwind CSS Conditional Styles
Tailwind CSS, a utility-first CSS framework, delivers robust and efficient tools to developers to construct responsive designs with fine control. However, one limitation in the framework is the lack of direct support for conditional styling. This can be a challenge when you're trying to apply styles based on a certain condition. In the midst of this, JavaScript can serve as an excellent ally to apply conditional styles with Tailwind CSS. To add a style on a condition in Tailwind CSS, JavaScript can assist you. Take this statement into consideration: You might desire to change the background color of a div based on whether a user has clicked a button or not. Your first step would be to get a reference to the elements you will be manipulating:Here we have a div with a blue background and a button that, when clicked, should alter the color of the div's background. Following this, you'll need to add an event listener to the button within your JavaScript code:...This JavaScript block listens for a 'click' event on the button with an ID of 'myButton'. When the button is clicked, it executes a callback function. Within this function, we now need to alter the color of our div. Syntactically, we accomplish this by utilizing the classList property, which permits us to add and remove CSS classes:document.getElementById('myButton').addEventListener('click', function() { // Code to change color goes here... });The last chunk of JS code interacts with the div by toggling its existing class 'bg-blue-500' for a new one, 'bg-red-500'. As a consequence, when the button is clicked, the div's background shade changes from blue to red. Remember Eddie Vedder's words, "I just feel like every kid is growing up too fast and they're seeing too much. Everything is about speed, and I think that the need for speed is a misdirected issue." Including dynamic interaction in your websites means introducing a temporary pause in order for JavaScript to load and execute. However, the level of interactivity JavaScript introduces is considered beneficial. By incorporating an event-driven programming language with a utility-first CSS framework such as Tailwind CSS, you’re arming yourself with a potent blend that crafts engaging web experiences.document.getElementById('myButton').addEventListener('click', function() { var myDiv = document.getElementById('myDiv'); myDiv.classList.remove('bg-blue-500'); myDiv.classList.add('bg-red-500'); });Understanding JIT: Enhancing Style Conditions in Tailwind CSS
Understanding 'Just-In-Time' (JIT) mode in Tailwind CSS is essential to enhancing style conditions. JIT, a term coined by the creator of Tailwind CSS, Adam Wathan, benefits developers as it provides the full power of Tailwind's utility-first approach while keeping resulting file sizes small, hence improving site performance. Adding a Style on Condition in Tailwind CSS Often, web design demands conditional styling — an element's appearance changes based on some state or action. Achieving this with Tailwind CSS involves understanding the combination of JavaScript (JS) logic and Tailwind classes. However, due to our limitations, we won't discuss JS here, but rather focus purely on the mechanics of defining styles conditionally using just Tailwind CSS and HTML. By default, Tailwind provides pseudo-class variants such as `hover`, `focus`, `active`, etc., which can be prepended to any utility class to control that utility's state. An example below:Here, when the `div` is being hovered over, its background changes from gray to blue — effectively appending a style based on a condition. JIT Mode Enhancing Style Conditions JIT in Tailwind goes leaps beyond, allowing us to create styles on the fly for almost any state or condition. To enable JIT, update your `tailwind.config.js` as follows:<div class="background-gray-100 hover:background-blue-500">Hello!</div>With JIT enabled, styles are created as you use them, and unused styles are automatically purged, leading to highly efficient file sizes. A significant advantage of JIT is that you can now create an arbitrary style on demand using square brackets (`[]`). Suppose you want to change the color and size of text within a button only if it's disabled:module.exports = { mode: 'jit', ... }In this instance, the text-color becomes '#123ABC', and its size adjusts to 2em only if the button `disabled` is true. This level of precise, ad-hoc styling isn't available without the JIT mode activation. However, bear in mind this visual update would require additional logic (i.e., JavaScript) to provide truly dynamic behavior based on real-time analysis and calculation. "We don’t make software for free, we make it for freedom." - Richard Stallman, Founder of the GNU Project, emphasizing the purpose behind creating tools like TailwindCSS and JIT.<button disabled class="text-[#123ABC] text-[2em] border bg-red-600">Click Me!</button>Case Study Approach: Real-world Scenarios of Conditional Styling using Tailwind CSS
In the web development world, implementing conditional styles in Tailwind CSS is an innovative approach to bring about more dynamic layouts and appearances. Understanding how Tailwind CSS works is the first step towards achieving this feat. As a utility-first-based framework, it provides low-level utility classes that let you build completely custom designs without leaving your HTML [source]. For instance, the background color of an element can be simply modified by adding specific class names like `bg-red-500` for a red background or `bg-blue-500` for a blue one. Thus, conditionally applying styles in Tailwind CSS typically entails the dynamic addition or removal of these classes based on certain conditions. This can happen in various scenarios such as: * Highlighting a selected navigation menu item * Changing button colors based on state (active, disabled, etc.) * Displaying different background colors for different user roles * Applying a different style when an element is hovered over or clicked. A common approach for introducing this dynamism lies in leveraging JavaScript's abilities to manipulate the Document Object Model (DOM). Specifically, JavaScript can add or remove classes from elements based on certain conditions. Here's a basic example:This script alternates the button's background between red and blue each time it's clicked. The `addEventListener` handles the click event, and within its callback function, it checks if the button currently has the `bg-blue-500` class. If true, it removes that class and adds `bg-red-500`, and vice versa. Also, popular JavaScript frameworks like React, Vue.js, and Angular offer built-in ways to apply conditional classes. With React, for instance, you might use a ternary operator directly in your JSX:let button = document.querySelector('button'); button.addEventListener('click', function() { if (button.classList.contains('bg-blue-500')) { button.classList.remove('bg-blue-500'); button.classList.add('bg-red-500'); } else { button.classList.remove('bg-red-500'); button.classList.add('bg-blue-500'); } });As Bill Gates once said: "The advance of technology is based on making it fit in so that you don't really even notice it, so it's part of everyday life." And indeed, with modern tools like Tailwind CSS combined with JavaScript, we're able to make web pages feel much more interactive and 'alive' in ways that were harder to achieve in the past.While the application of condition-based styles in Tailwind CSS may seem complex at first sight, it is indeed a remarkable method, powered by the innovative features offered within Tailwind's efficiency-driven module. Parts of this final solution require a profound understanding of the conditional logic which this utility-first framework promotes. Let's explore this topic further: A common pattern that you are likely to encounter when crafting dynamic interfaces with Tailwind CSS includes the need to add a different style on a specific condition. These conditions could be browser related, user interaction related or component state related. An important thing to remember is that while Tailwind itself doesn't come with built-in conditional logic, it is designed to work seamlessly with modern Javascript (or any other language) frameworks. Thus, extending its capabilities with conditional styling could be done on another layer - the JS layer. How can you achieve this in practice? Here's how: For instance, if you're using React as your development stack, you might want to conditionally add different color classes to a button based on its states. Below is a simplified demonstration: html<div className={condition ? 'bg-red-500' : 'bg-blue-500'}></div>Not only is this approach incredibly adaptable due to the modular nature of both Tailwind and JavaScript-based frameworks, but it also ensures your design maintains overall consistency alongside dynamic, interactive elements. On the SEO front, integrating such highly functional, tailored features organically draws in users seeking solutions to complex design dilemmas. The combination of well-crafted, responsive designs with Tailwind’s utility-first framework fosters an online environment where users spend more time interacting with your content. This increased engagement translates to promising SEO performance across various metrics like bounce rates and dwell times, hallmark indicators used by search engines like Google to rank your content higher. It is essential to understand that the flexibility of this approach means there isn't a one-size-fits-all solution for every component out there. Each component has different requirements and as such would need a different approach. It provides developers with robust control over their web layout, increasing overall productivity and fostering a dynamic structure unlike anything else. As per the words of Paul Irish, a renowned developer advocate and influencer in the technology industry, "The main challenge of software craftsmanship is not to build what can be built, but to build what should be built." This statement resonates strongly within the context of condition-based style application in Tailwind CSS. It presents the possibility and ease of use in leveraging the powerful features in our toolboxes such as Tailwind CSS to create the elegant and interactive web interfaces demanded in today's digital era. In closing, building adaptive, user-friendly designs with condition-based styling in Tailwind CSS greatly enhances the user experience. This strategy accelerates your journey toward establishing a strong, innovative web presence marked by stellar SEO visibility and superior user engagement.Related