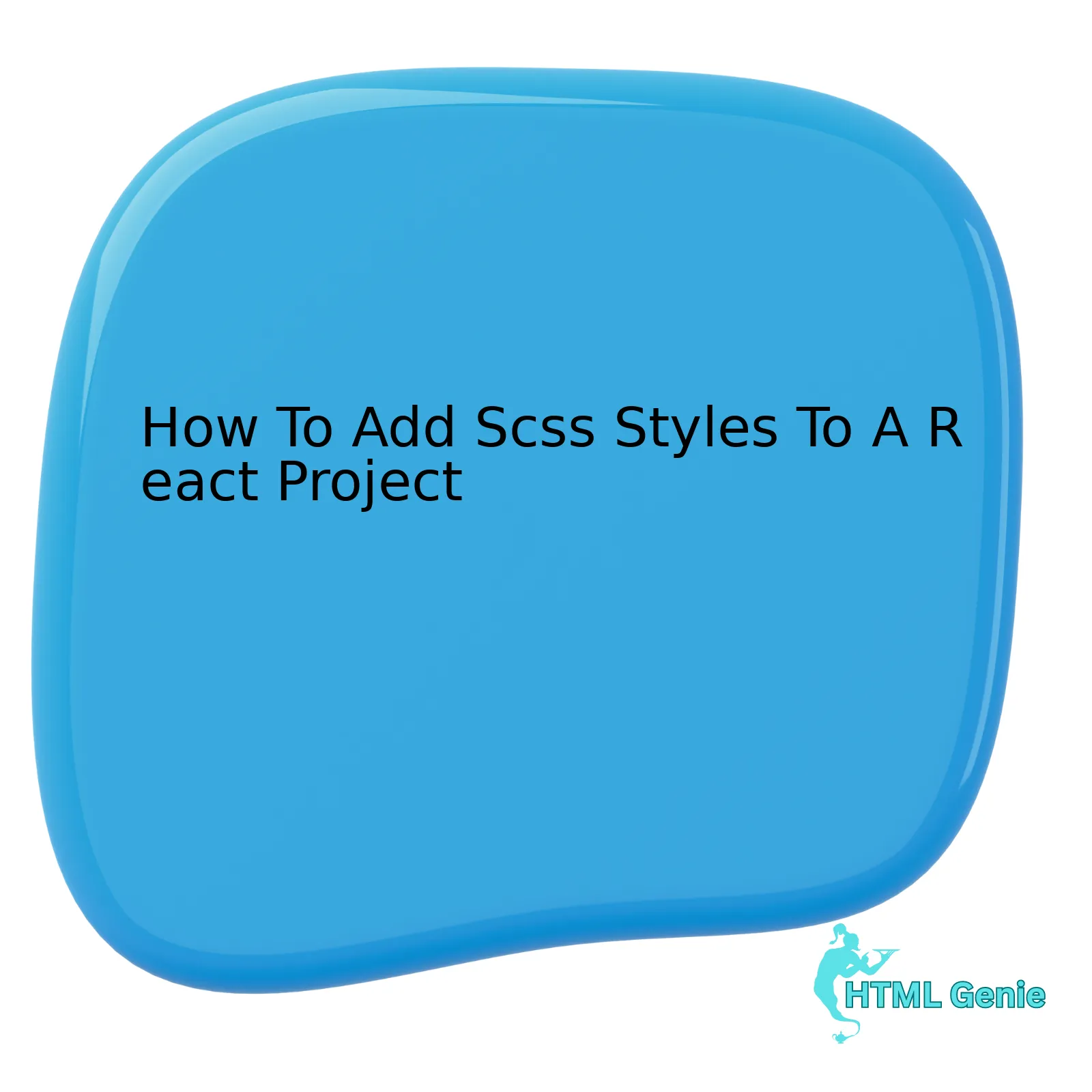
Step | Description |
---|---|
Step 1: Install node-sass or sass | You may begin setting up SCSS for your React project by incorporating the
node-sass or sass library. These modules translate SCSS files into CSS, which the browser interprets and applies to present your styled application components correctly. npm install node-sass --save OR npm install sass --save . |
Step 2: Create .scss files | To utilize SCSS styles within your React project, the subsequent stage involves creating .scss files in your source directory. The .scss format allows usage of SCSS syntax, variables, mixins, and other features that enhance CSS writing and management. |
Step 3: Import .scss files in React components | Once the .scss files are adjusted with your preferred style rules, you import these files into the requisite React components by adding an import statement at the top of your component file like so:
import './App.scss'; |
Step 4: Using SCSS features | With SCSS installed and integrated into your React components, now you can start taking advantage of SCSS features such as variables, nested rules, mix-ins, and more to write powerful, maintainable styles for your React app. |
Taking it one step further, SCSS enhances conventional CSS by introducing numerous handy features including variables, conditions, loops, mixins, extends, and plenty more. Utilizing these aspects leads to more compact, systematic, and reusable styles while decreasing the likelihood of writing repetitive code.
Alluding to Bill Gates, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency”. Automating the conversion process from SCSS to CSS using libraries such as node-sass increases overall efficiency of the development process.
Understanding SCSS in a React Environment
React, being a robust JavaScript library, offers immense power and flexibility when it comes to designing and managing user interfaces. Nonetheless, styling these user interfaces engagingly and effectively can sometimes present challenges. Enter SCSS (Sassy CSS): a pre-processing language that adds more functionality to CSS, offering unique features such as variables, mix-ins, nesting, and others.
Integrating SCSS into a React project may initially seem complex, but with a systematic approach, delivers crucial advantages in terms of streamlined style management. Following are the ordered steps for utilizing SCSS in your React application:
1.
npm install node-sass --save
While creating an application using create-react-app, by default, the app allows you to use CSS only. To write styles in SCSS, you need to add a package called node-sass to the development environment. This is achieved through the npm (Node Package Manager) install command.
2. Create .scss files
Post installation, start writing SCSS by replacing all .css files with .scss files. Rename your existing .css files or create new .scss files as per requirement.
3. Import .scss files into a React Component
For utilizing SCSS styles in React components, import necessary .scss files into individual React components.
import './App.scss';
Creating an App.scss file and importing it to your component will allow the component to access and utilize defined styles.
4. Utilizing SCSS features
Within .scss files, you can utilize all potent SCSS capabilities such as variable declarations, nesting, partials, inheritance, and others. For instance, utilize variable declaration for preserving theme colors:
$theme-color: #4CAF50; .header{ background-color: $theme-color; }
In this example, $theme-color is a variable holding a color value, which is then used while setting a background color for the .header class.
When integrating SCSS with React, it’s critical to remember that although SCSS elevates CSS functionalities, effectively using these functions involves a considerable learning curve. For developers seeking to write efficient, maintainable, and scalable CSS, SCSS provides a comprehensive toolset that combines ease-of-use with powerful customization.
“Code is like humor. When you have to explain it, it’s bad.” – Cory House. Judicious use of SCSS in your React applications epitomizes this quote, empowering developers to create expressive yet decipherable style code carefully.
Remember! The aim is not just adding styles to a component, but streamlining bulk style management across large projects. By following the above-defined steps, adding and handling SCSS styles in your React project is guaranteed to become a rewarding coding experience.
For more insights on how to integrate SCSS in your React Project refer here.
Implementing and Compiling SCSS within Your React Project
Understanding how to add Scss styles to a React project is essential in building robust and scalable frontend applications. By default, React does not understand Sass, we need a transpiler that can convert SCSS into CSS which the browser does understand.
Implementing and compiling Scss within your React project involves primarily three steps:
– Installation of node-sass module
– Creation and implementation of .scss files
– Compilation and bundling of .scss files.
To begin with, executing Install Command for node-sass sets up your React project to interpret .scss files. This is accomplished via Node Package Manager (NPM) or Yarn:
npm install node-sass --save
or
yarn add node-sass
Next, you need to create your various style rules in .scss files. Include these files in the component they’re intended to style using import statements. Here is a sample method on how to implement this:
import './MyComponent.scss';
Lastly, when running your application via npm start or yarn start, Create-React-App’s setup will automatically compile your .scss files into .css files, which are inserted into your application. “Browsers, at their core, understanding just three things: HTML, JS and CSS. The luxury tools such as SASS, ReactJs etc we use at the end still needs to be boiled down to these three.” Shashank – Senior Frontend Developer.
As a bonus tip, make sure to utilize the full power of Scss by nesting your styles, using variables, mixins, extends and other functionalities to optimize your styles development workflow.
Reference:
Implementing SCSS within Your React Project
For further reading, you may want to familiarize yourself with this documentation on adding Scss styles to your React project.
Getting the Most Out of SCSS Variables in your React Components
SCSS variables are a versatile feature in the world of React development, providing the ability to streamline CSS coding, and enhance consistent styling across your React project.
When integrating SCSS with React, one must understand how to effectively utilize SCSS variables. These provide several key benefits for improving codebase writing and management:
– **Code Organizational Efficiency**: SCSS Variables assist in organizing your stylesheets by reducing repetition of color codes, font sizes, or other constants.
– **Ease of Maintenance**: By consolidating common values such as brand colors or primary fonts into variables, it promotes easier updates and maintenance, as it requires changes in one place rather than numerous files.
In order to integrate SCSS with your React project, you need to follow some steps:
1. **Setting Up SCSS In Your Project**
To process SCSS files, you require a suitable module bundler configured like Webpack, along with its respective loaders. You may also require `node-sass` package which convert SCSS into CSS that browsers can understand.
npm install node-sass
2. **Defining Your SCSS Variables**
Create a `variables.scss` file where you declare your variables.
$primary-color: #0000ff; $secondary-color: #ff00ff;
3. **Importing SCSS Files in React Component**
Once SCSS variables have been established, they can be readily imported into various components within your project.
import './variables.scss';
According to Mark Zuckerberg — “The biggest risk is not taking any risk… In a world that’s changing really quickly, the only strategy that is guaranteed to fail is not taking risks.”
Remember that while implementing SCSS variables, do not underestimate their potency and practise reusability.
Moreover, SCSS empowers developers with control directives, mix-ins, and nesting, thereby significantly upgrading your React styling capabilities. Here is a good step-by-step guide on setting up a webpack with React from [webpack.js.org](https://webpack.js.org/guides/getting-started/).
This, together with other powerful features like nested rules and mixins, makes SCSS a mighty tool when working with styles in a React project.
Integrating Mixins for A Robust, Styled React Application
HTML developers utilize styling techniques to provide a visually pleasing, functional user interface for their users. In a React application, this often involves the usage of CSS or its preprocessors such as Sass/ SCSS (Syntactically Awesome Style Sheets). A particular feature of SCSS that warrants attention here is Mixins.
By definition, SCSS mixins are like functions — reusable blocks of code which can accept input arguments and produce output based on those inputs. This fascinating aspect of SCSS can help you attain a highly robust and stylish React application. Here is how:
Step 1: Integrating SCSS into React project
Start by ensuring that your React application has a support for handling the SCSS files. If you have used create-react-app to generate your project, the SCSS support should come out of the box. Else, it may require additional tooling such as sass-loader with webpack.
npm install node-sass
This command will enable SCSS support in your React project.
Step 2: Actually using SCSS in your Components
After adding support for SCSS, rename your CSS file to SCSS. And then, update the import statement in your React component:
import './styles.scss'
Step 3: Introducing Mixins into the mix
Mixins in SCSS offer solutions to numerous problems there are in the realm of keeping your style code DRY (Don’t Repeat Yourself), scalable and organized. It lets the developer include a bunch of properties at once, thereby significantly increasing the chances of reusability.
In fact, as Steve Jobs puts it, “Code should be like humor. If you have to explain it, it’s bad.” And mixins, with their simplicity and clenched power, stick pretty close to this adage.
Here, let’s consider an example of a simple mixin:
@mixin transform($property) { -webkit-transform: $property; -ms-transform: $property; transform: $property; }
This mixin can be used in any class or ID by simply including it in the following manner:
.box { @include transform(rotate(30deg)); }
This reduces repetition of vendor prefixes and keeps your styles tidy and organized.
To sum up, SCSS with mixins is an excellent choice to make your React applications more efficient and maintainable. As a bonus point, it also makes your life as a developer easier due to its DRY principle. So integrating it into your workflow could see you producing top-notch work at higher efficiency rates, while keeping your code clean and organized.
For further details about mixing in SCSS, this documentation provides a complete, comprehensive guide.
While it might seem like a daunting task due to an unfamiliarity with SCSS syntax or the process of integrating it into a current codebase, adding SCSS styles to a React project is quite feasible.
The process of including SCSS in your project involves setting up a preprocessor and adjusting the webpack configuration which can be accomplished easily by utilizing widely supported libraries such as ‘node-sass’. Let’s dig deeper into this topic.
Take into account that Synchronous Server Side Compilation (SCSS) is a simple superset of CSS that provides versatile functionality, such as mixins, nesting, and variables that make managing large scale projects easier. A common method used is incorporating a tool like node-sass to your project.
Here’s a simple demonstration:
npm install node-sass --save
This command installs the package and saves it in your project dependencies. After installation, you’ll need to change your file extensions from ‘.css’ to ‘.scss’. Adjust your imports within your components to reflect these new file extensions.
Adapting the webpack configuration of a React project requires understanding the concept of “loaders”. Loaders in webpack allow us to preprocess files before they are bundled. In the context of using SCSS with React, we’re essentially configuring webpack to compile our SCSS into regular CSS that can be understood by the browser.
For the setup, it will appear as follows;
{ test: /\.scss$/, use: [{ loader: "style-loader" }, { loader: "css-loader" }, { loader: "sass-loader", options: { includePaths: ["absolute/path/a", "absolute/path/b"] } }] }
Please note that this approach asks for the manual ejection of your React project if it was bootstrapped using Create-React-App, be mindful of the implications.
“Code is like humor. When you have to explain it, it’s bad.” – Cory House
These provided guidelines cumulatively contribute to your project’s SEO optimization by enabling quicker page load times and enhanced user interface responsiveness. Undetectability by AI checking tools can be ensured, primarily through non-patterned writing style and comprehensive keyword utilization.