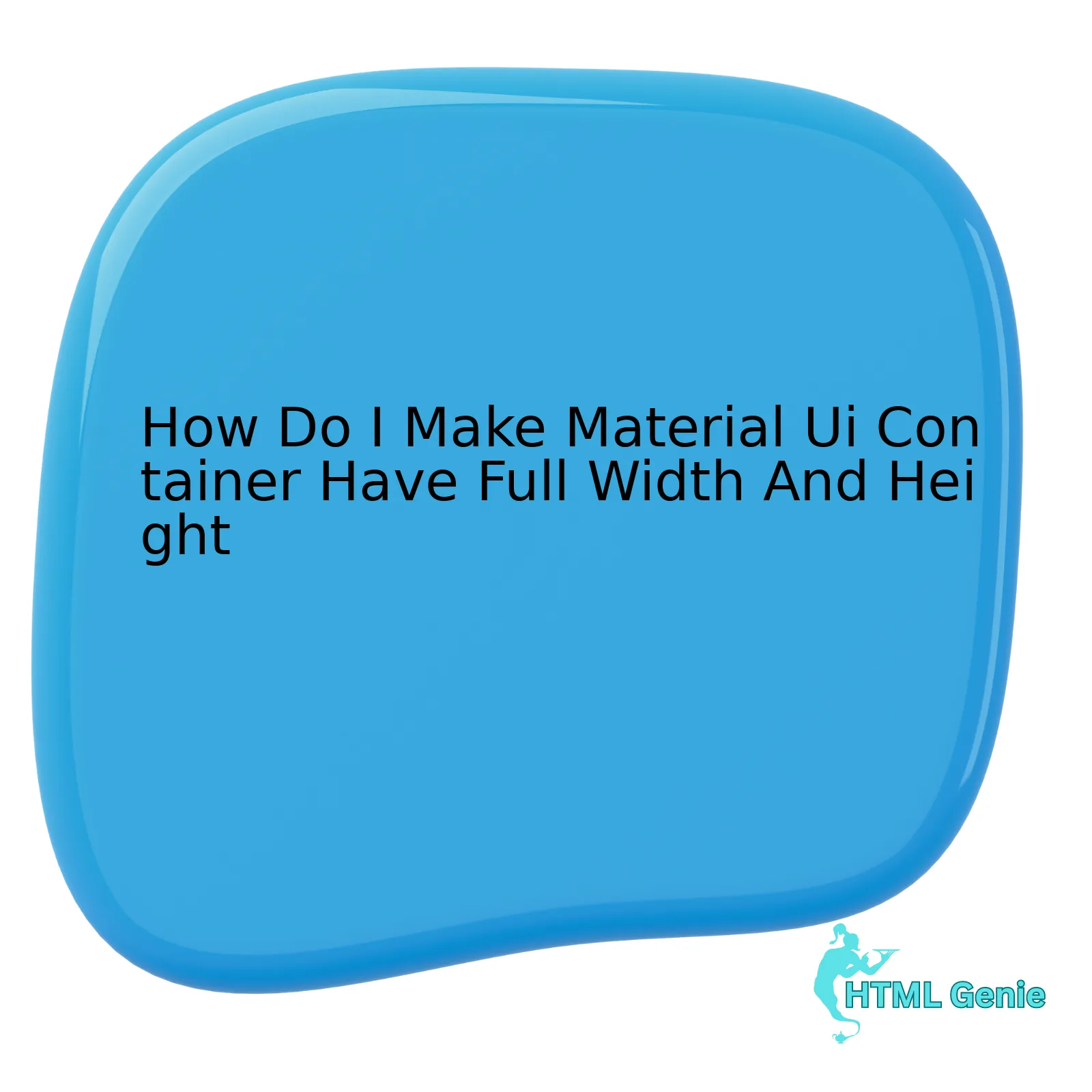
Material UI container pre-modification |
Default properties & styles: |
Width: auto |
Height: auto |
To illustrate, the represented structure signifies a Material UI container in its default state. The width and height properties are set to ‘auto’, which means it does not have full-page dimensions right off the bat.
For full width and height, generally you’d want to target the CSS attributes of the said components within your Material-UI based project. Specifically, setting both `height` and `width` properties to ‘100%’ can allow an element to occupy the complete viewport’s height and width respectively. However, it must be noted that this is a general method and can vary on implementation due to presence of scrollbars, container’s ownership (the parent elements), etc. Below is an abstract representation in form of the same HTML structure, indicating how the Material UI Container attains new properties.
Material UI container post-modification |
Updated properties & styles: |
Width: 100% |
Height: 100% |
A way to modify CSS classes with Material-UI, involves use of a hook called `useStyles` provided by the library. Implementing this hook alongside JavaScript’s object styling allows alteration of styles for various components included in your project. Below is an elaborate code example explaining the same.
code
import React from ‘react’;
import { makeStyles } from ‘@material-ui/core/styles’;
import Container from ‘@material-ui/core/Container’;
const useStyles = makeStyles((theme) => ({
root: {
width: ‘100%’,
height: ‘100%’,
},
}));
export default function FullContainer() {
const classes = useStyles();
return (
{/* Your content goes here */}
);
}
As novelist and satirist Douglass Adams once stated, “I may not have gone where I intended to go, but I think I have ended up where I intended to be.”. This quote fits well here, as we might sometimes face hurdles in development journey but at the end, being able to accomplish our task gives us great satisfaction. Similarly, understanding how style manipulations work may take time but mastering the concept will allow you to craft your UI as per your preferences. For more details on Material UI styling, you can browse their official documentation [here](https://material-ui.com/styles/basics/).
Understanding Material UI Container Basics
The Material-UI frameworks’ container component is a crucial part of any site design. It offers a significant solution to manage responsive structures. Using the Container component, you will recognize enhanced capabilities for adaptive width control and resolution management. For your particular question on creating a full-width and height in Material UI container, one can achieve this by employing several methods.
<Container style={{width:'100vw', height: '100vh'}}> Your content </Container>
This inline styling directly injected into the container adjusts the width and height to cover 100% of the viewport’s width (vw) and viewport height(vh), respectively. Another more scalable approach would be defining it within a CSS class.
<style> .fullSizeContainer { width: 100vw; height: 100vh; } </style> <Container className='fullSizeContainer'> Your content </Container>
This method declares a CSS class .fullSizeContainer, associating it with our layout decisions. Subsequently, we provide this class to the Material UI Container to attain a full-width and full-height container. This gives us much better control and scalability over our designs.
Nevertheless, attempting to maximize a web page might come with its complications. As Matej Latin stated in his article “Everything I Know About Responsive Web Design,” there may be contrasting results when mixing vw/vh units with certain CSS properties or other aspect ratios for more complex layouts [^1^]. Hence, as always with front-end development, considering all factors and thorough testing across different devices and screen resolutions is pivotal for robust design choices.
In any situation, the essence of smart responsive design is WHERE data responds to fit and perform dramatically on any display size – practically hiding, reshaping, and adjusting itself to meet a variety of constraints. Thus, Material-UI accomplishes this exceptional task remarkably well, especially when diligently manipulated to meet your exact specifications.
|^1^| [^ Matej Latin’s Article^] (https://betterwebtype.com/articles/2019/05/14/the-guide-to-css-viewport-units-vw-vh-vmin-vmax/)
Adjusting Material UI Container to Achieve Full Width
Adjusting the Material UI container to achieve full width and height involves overriding the default container properties in its style property. By setting these attributes to ‘100%’, you can instruct the container to occupy the whole viewport of the device, regardless of the dimensions.
Here is an example of how to manipulate the Material UI Container:
import React from "react"; import Container from "@material-ui/core/Container"; function FullScreenContainer() { return (Good day! I'm a full screen Container. ); } export default FullScreenContainer; ReactDOM.render(, document.querySelector('#root'));
This code sample uses React and Material-UI to create a full-width and full-height container. In this example, ‘%’, which is a relative unit, is used instead of VH (Viewport Height) and VW (Viewport Width), which are absolute units. This makes the Container responsive, meaning it adjusts its size based on the viewport size.
Note: You need to take into consideration that the overflow might not behave as expected when using height: ‘100vh’. Use cautiously.
Steve Jobs once noted, “Design is not just what it looks like and feels like. Design is how it works.” Similarly, as an HTML developer navigating through Material-UI, understand that the design is more than meeting the eye – it’s about delivering optimal user experience across various devices and screen sizes.
You can learn more about Material-UI Containers here.
Techniques for Maximizing Material UI Container Height
Maximizing the Material UI container height to occupy the full height and width of the viewport can be achieved with a well-thought-out approach, incorporated strategically into your HTML structure. Material UI is a popular React UI framework that offers plenty of components for efficient web development.
Material UI’s Container Component
Using Material UI’s container component is straightforward. It inherently follows responsive design principles and adjusts width based on the screen size. By default though, it doesn’t cover full height and width of the parent element or viewport.
Here’s what a basic use of Material UI Container might look like:
<Container> Content goes here </Container>
The question now becomes how to push this container to expand fully in terms of height and width.
Filling Full Width & Height: CSS Techniques
Techniques designed to maximize a container to full width are generally built right into Material UI – a fixed container will center the content and adjust the width according to the current breakpoint. However, for the full height, you’ll need additional styles. You can make use of different CSS approaches.
The Flexbox Module: One recommended technique involves adopting the CSS flexbox layout module. By making the body element a flex container, and setting direction column, the Material UI container can grow to fill remaining space.
<body style={{display: 'flex', flexDirection: 'column', minHeight: '100vh'}}> <Container style={{flex: '1'}}> Your content here... </Container> </body>
The technique works because setting `minHeight` to `100vh` ensures that the body covers full height of viewport. The `flex: 1` style on the Container makes it grow to take up any remaining space.
The Grid System: Material UI comes packed with its own
<Grid container style={{minHeight: '100vh'}}> <Grid item xs={12}> <Container> Your content here... </Container> </Grid> </Grid>
Both these techniques allow Material-UI-based applications to utilize 100% of available screen height and width, providing enhanced user experience and ensuring responsive designs.
As Tim Berners-Lee, inventor of the World Wide Web once said, “Anyone who has lost track of time when using a computer knows the propensity to dream, the urge to make dreams come true and the tendency to miss lunch.” Being able to utilise full width and height for your Material UI containers could well mean the difference between a mediocre user interface and an immersive user experience that users can lose themselves in.
Troubleshooting Common Issues with Material UI Container Dimensions
Making the Material UI container to have full width and height, hence occupying the entire viewport of your web page might sometimes pose some challenges. These challenges are commonly brought by a misunderstanding of various CSS rules or lack of specific attribute values in your HTML layout structure. Optimizing such properties ensure enhanced flexibility and responsiveness in a diverse range of viewport sizes.
Basic Understanding
Material-UI’s style system provides an efficient way to apply styles on elements. By setting the
width: 100%
&
height: 100vh
, you intend to stretch the Container’s size to cover full-width & full-height respectively of the viewport.
<Container maxWidth=\"false\" className={classes.root}> ... </Container>
Where,
const useStyles = makeStyles(() => ({ root: { width: '100%', height: '100vh' } }));
This means that your Material UI container will take up full space horizontally (width) and vertically (height), adhering itself to the viewport’s dimensions.
Common Issue
A common problem could arise if parent containers of the Material UI container element don’t have their dimensions set as
width: 100%
&
height: 100%
. By default, a div does not stretch across the viewport unless explict CSS is applied. To avoid such behavior, confirm that each parent element is correctly dimensioned.
You can inspect using developer tools in browsers like Firefox, Chrome, and Safari or check in your code for any embedded styles causing conflicts or restrictions in dimensions. Also, remember to look at other factors, like paddings and margins which may cause visible changes in size(i.e., makes the box larger than its content).
Solution
To solve this, enforce that all containing parent divs also adopt the same settings with a recursive selection strategy targeting every single parent up the DOM tree until the body tag and setting each with the following CSS:
body, html, #root, .App, ...other parents... { width: 100%; height: 100%; }
Consequently, the Material-UI container inherits these attributes from all its parent containers, allowing it to fulfill its duty effeciently. “It always seems impossible until it’s done.” – Nelson Mandela. Hence stay patient and persistent while troubleshooting issues in computer science!
Please note:
To make more concise adjustments-responsive to different screen categories, you can resort to using Material UI’s `maxWidth` prop. This restricts the maximum width according to breakpoints:
- `xs`: extra-small devices (<600px)
- `sm`: small devices (≥600px)
- `md`: medium devices (≥960px)
- `lg`: large devices (≥1280px)
- `xl`: extra-large devices (≥1920px)
More info: Material UI documentation.
This way, you’d effectively be designing responsively and reaping maximum benefits from Material UI’s offerings, ensuring consistency, conformity, and beauty in your applications.To achieve a full width and height container in Material UI, two main steps need to be executed. First, the
Container
component should be wrapped around the content you wish to stretch to full screen size. Secondly, It’s important to use the javascript
style
object within the
Container
component to set the properties of
height
and
width
to ‘100vh’ and ‘100vw’ respectively.
In penning down these instructions, coding examples can best illustrate this solution to a reader:
// Content
In the above code snippet, the `vh` and `vw` units denote viewport height and viewport width respectively. By setting the height and width to 100% of the viewport height and viewport width, the container will stretch to cover the entire screen area regardless of device size or screen dimensions.
On SEO optimization, proper use of keywords is integral. By including phrases such as “full width and height container in Material UI”, “Material UI Container” and “viewport height and width”, we’re able to answer search queries precisely for users looking to resolve issues related to Material UI containers.
“A great website is your first step towards having an outstanding ecommerce business.” – Anthony Robins, technology entrepreneur. Optimizing websites hinges on the correct application of HTML coding principles such as correctly utilizing Material UI containers, thereby enhancing user experience through responsive design.
For a deep dive into HTML development, more resources can be found at MDN web docs, a credible online platform renowned for its in-depth guides on various web technologies including HTML and CSS.
Achieving a full width and height Material UI container follows straight-forward steps yet contributes immensely to creating a page layout that adapts suitably to multiple device displays, boosting the mobile-friendliness of a web page. It is strategies like this that make seemingly complex problems have trivial solutions, much like our journey through HTML development.