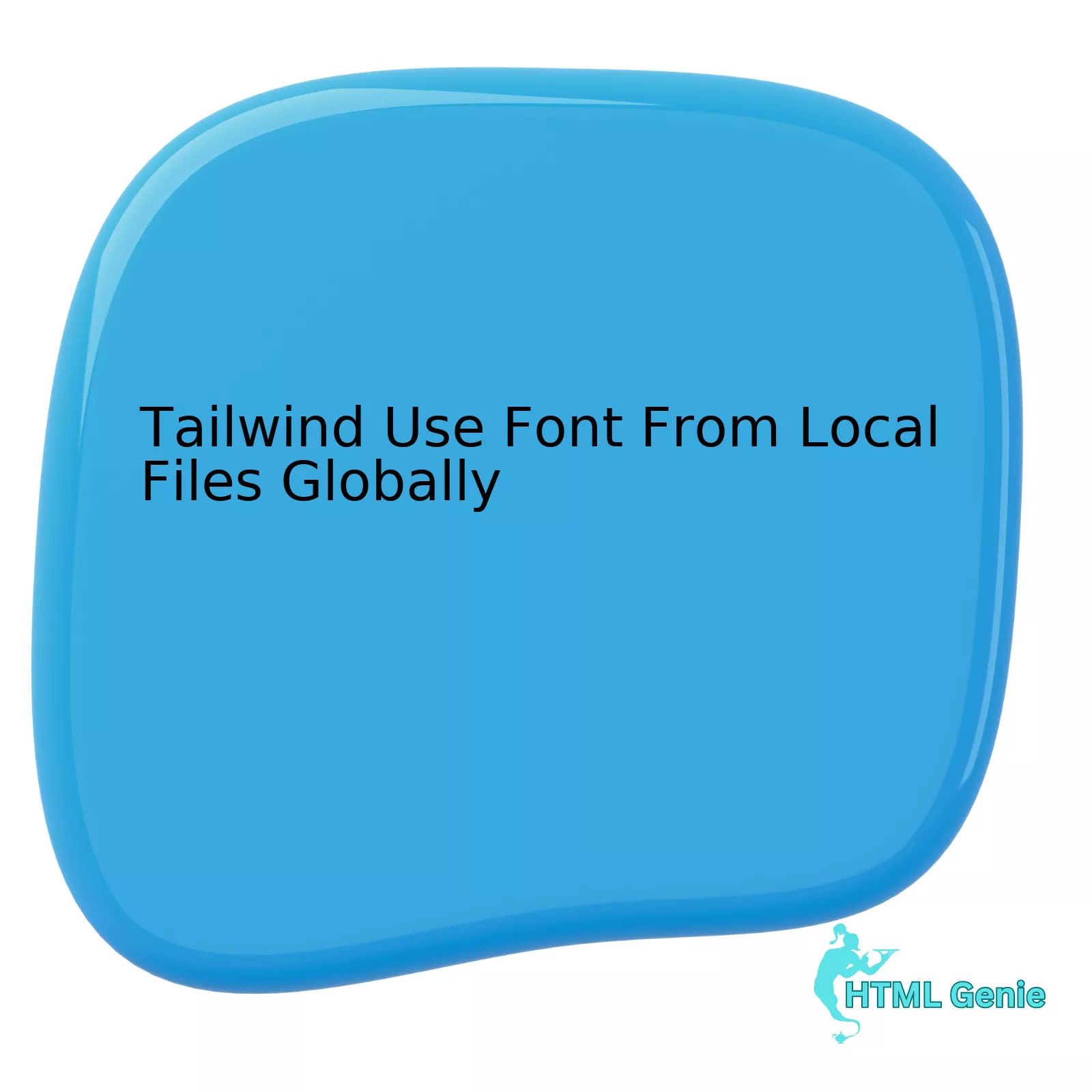
Action | Procedure for Tailwind Use Font From Local Files Globally |
---|---|
Create an Assets Folder | In your project root, create a new directory named ‘fonts’. This is where you should place all your font files. |
Update tailwind.config.js | Alter your TailwindCSS configuration file to reference the local font files. Specify these within the theme’s fontFamily property. |
Use @apply Directive | You can apply these fonts globally with the use of the ‘@apply’ directive within your CSS. |
To explain it detail, this whole process involves three main actions:
– First, creating an assets folder in the root of your project specifically named ‘fonts.’ The role of this folder will be hosting all your necessary font files that are going to be accessed by the Tailwind CSS.
// Typical directory structure - src - public - package.json - tailwind.config.js - fonts (newly created)
– Next step revolves around the modification of your ‘tailwind.config.js’ file, effectively including the font references into the theme’s fontFamily property therein.
module.exports = { theme: { fontFamily: { display: ['Gilroy', 'sans-serif'], body: ['Graphik', 'sans-serif'], }, extend: { colors: { cyan: '#9cdbff', }, margin: { '96': '24rem', '128': '32rem', }, } }, variants: { opacity: ['responsive', 'hover'] } }
– Lastly, applying these fonts across your website can be done through the ‘@apply’ directive within your CSS. The mentioned directive allows extracting classes into reusable snippets resulting in DRY (Don’t Repeat Yourself) code.
“Code is read much more often than it is written, so plan accordingly.” – Jeff Atwood
html { @apply .font-body; } h1 { @apply .font-display; }
We just saw how Tailwind makes it straightforward to harness the power of locally stored fonts across an entire website. It enables developers to maintain consistent visual branding and typography in web projects without being dependent on external resources or libraries.
Unveiling the Magic: Setting up Tailwind with Local Font Files
Tailwind CSS, the utility-first CSS framework known for its speed and efficiency, empowers developers with a host of customizable classes. A popular requirement is to setup Tailwind to utilize locally stored font files. There are major benefits to using local fonts, primarily speed and reliability as the loading times are generally faster and less dependent on external factors.
Configuring Tailwind CSS to utilize local fonts globally requires you to take a few steps in your project:
– First, obtain your desired font files in formats that are web-friendly (e.g., .woff or .woff2). Store these file formats in a directory within your project – a common choice is the ‘assets/fonts’ directory.
– Next, in your tailwind.config.js file, specify the path to your font files using the fontFamily theme function provided by Tailwind CSS.
The following code exemplifies the mentioned instructions:
html
module.exports = { theme: { extend: { fontFamily: { sans: ['YourFontName', ...defaultTheme.fontFamily.sans], }, } } }
As represented above, ‘YourFontName’ must be replaced by the name of your local font files.
However, this will only work if you import your fonts into your CSS file. Therefore, include an @font-face rule at the beginning of the same:
html
@font-face { font-family: "YourFontName"; src: url("/path/to/your/fontFile.woff") format("woff"), url("/path/to/your/fontFile.woff2") format("woff2"); }
Replace ‘/path/to/your/fontFile.woff’ and ‘/path/to/your/fontFile.woff2’ with the actual paths to your font files. You can add multiple sources to the src property for various scenarios including fallbacks.
By diligently following these steps, you can effortlessly configure Tailwind CSS to use global font files stored locally, not only improving load speeds but also broadening customization options.
“Talk is cheap. Show me the code.” – Linus Torvalds. This quote beautifully signifies the importance of coding parctice in understanding concepts like these. So go ahead, flex your coder muscles and plunge into the Tailwind waters. Remember, practice makes perfect!
For more specifics on configuring Tailwind, consider reading their official documentation. It’s comprehensive, well-written, and includes plentiful examples and insights.
Remember, taking advantage of local font files whilst working with Tailwind CSS helps curate a swift and reliable web experience, thereby enhancing the overall user satisfaction.
Breaking it Down: How to Use Local Fonts Globally in Tailwind
The use of local fonts globally in a Tailwind project could dramatically enhance the unique look and feel of your web content, making it more engaging for the end user. Web developers are constantly striving to create distinctive designs, and applying custom typefaces can be key to this process.
To use fonts from local files globally in Tailwind, view the process in five incremental steps:
🔸 **Step 1: Installation and Configuration of TailwindCSS**
Tailwind needs to be properly installed and configured before integrating local fonts. It is convenient to initialize a new configuration file using
npx tailwindcss init
, giving you access to modify settings as per requirements. The official website for Tailwind here provides detailed installation instructions.
🔸 **Step 2: Import Font Files**
After ensuring that the font files are available locally (typically in formats such as .ttf, .woff, or .woff2), import them into your project directory, preferably in a dedicated ‘fonts’ folder within assets.
@font-face { font-family: 'MyCustomFont'; src: url('/path/to/your/font-file.woff') format('woff'); }
This @font-face declaration imports the font into your CSS.
🔸 **Step 3: Declare Fonts in tailwind.config.js**
Next, declare your imported font(s) in the Tailwind config file. The fontFamily property within the theme object should include your custom font’s name. This action integrates your typeface with Tailwind’s configuration and makes it usable via class selectors.
// tailwind.config.js module.exports = { theme: { fontFamily: { 'custom': ['MyCustomFont', ...] }, }, }
🔸 **Step 4: Adding Font to HTML**
Apply the custom font globally with Tailwind utility classes. Place it on the highest level of your markup, typically at the root element or the body.
<body class="font-custom"> ... </body>
Your project now uses the locally stored font globally.
🔸 **Step 5: Building Your Utility Classes**
Finally, build your utility classes with `npx tailwindcss build input.css -o output.css` to generate your utility classes.
In the words of Steve Jobs, “Design is not just what it looks like and feels like. Design is how it works.” This quote emphasizes the emphasis that must be placed on good design – it’s about much more than just appearances. Having unique, attractive typography plays a significant role in this, especially in web development. The ability to seamlessly integrate custom local fonts solidifies Tailwind’s position as a powerful utility-first CSS framework.
However, please keep in mind to obtain necessary licenses or permissions for any copyrighted typefaces used in your projects.
Maximizing Efficiency: Configuring Tailwind for Global Usage of Local Fonts
For ensuring maximum efficiency while configuring Tailwind CSS for global usage of local fonts, one can integrate the utility-first CSS-library, such as Tailwind to customize your fonts. Before delving into the process, it’s crucial to understand that local fonts are files on your computer or server rather than being hosted by a third-party provider like Google Fonts. Using local fonts globally with Tailwind offers benefits like fewer HTTP requests and faster load times.
The configuration process is specified in Tailwind’s documented strategy and follows the following general steps:
Firstly, install Tailwind via npm:
npm install tailwindcss
Tailwind directly works on your project’s CSS file. Initiate a configuration file through:
npx tailwindcss init
This step creates a `tailwind.config.js` file at your project root.
Locate the `theme:` key in the `tailwind.config.js` file where you will customize the fonts. The register format of local fonts requires providing a name and the source-url, which suits perfectly to be done under `theme:` like this:
module.exports = { theme: { extend: { fontFamily: { 'custom-font': ['Font-Name', ...defaultTheme.fontFamily.sans], }, }, }, variants: {}, plugins: [], }
In the example above, ‘custom-font’ refers to your personalized font-family identifier and ‘Font-Name’ is the name of the specific font-file introduced in the CSS.
Thereafter, include the font-face declaration to your style.css, specifying the address of the font file:
@font-face { font-family: 'Font-Name'; src: url('/path/to/font/Font-Name.ttf') format('truetype'); }
Finally, add the `.font-custom-font` class to any text element that you want to style:
<p class="font-custom-font">This is your custom font!</p>
It is pertinent to specify that the `extend` function is used instead of directly assigning the `sans` array so that all the default configurations aren’t overridden.
As brothers-in-arms Kevin and Joshua Walsh stated, Modern internet makes quick, iterative changes possible – so code should too.
, it’s common sense to opt for methods that eases up the workflow. A technology like Tailwind certainly contributes to fulfilling this concept in web designing and development.
You find more detailed steps in Tailwind official documentation [here](https://tailwindcss.com/docs/configuration).
By carefully implementing these steps, you configure Tailwind for global usage of local fonts seamlessly while also maximizing efficiency in your project.
The Technique Explained: Incorporating Local Font Files into a Global Scope with Tailwind.
Deploying local font files in a global capacity using Tailwind CSS, a modern utility-first CSS framework, is an effective approach that permits centralized control over typography throughout the project. This process essentially involves transposing local font files into the worldwide space of Tailwind. The convenience and flexibility this offers make it an appealing choice among modern HTML development practices.
The initial task on the journey towards establishing this system would involve downloading and storing your chosen font files within your project’s file structure. Various subsets and formats of fonts may be needed to ensure cross-browser compatibility. Popular formats include .woff and .woff2 – these will often adequately cater to most contemporary web browsers:
/fonts /your-font.woff /your-font.woff2
Following storage of your desired font files, you’ll update Tailwind’s configuration file, tailwind.config.js, with typography-specific directives. Specifically, within theme.extend, one would define fontFamily with an object bearing a custom name alongside paths to the requisite font files:
module.exports = { theme: { extend: { fontFamily: { 'custom': ['"Your Font"', 'sans-serif'], }, }, }, }
Subsequently, you can utilize your custom font globally across your project by applying it through Tailwind’s font utilities. By adding ‘font-custom’ to any HTML element’s class attribute, the desired typography will be reflected:
\This is some text.\
Utilizing local font files in this manner optimizes aesthetic consistency across your online content while reducing potential reliance on external resources such as Google Fonts. Moreover, loading local files often results in high-speed rendition of webpages, contributing to an overall better user experience.
As Steve Jobs once said, “Design is not just what it looks like and feels like. Design is how it works.” Imbuing web design with functionality allows developers to strategically incorporate fonts, optimize their utility, and accentuate accessibility, ultimately elevating user experience. By employing local font files on a global scale with Tailwind, developers capitalize on form and function, creating stunning, streamlined designs in accordance with best coding practices.
For more information, consider reading the official documentation on configuring Tailwind for global styles incorporation.Utilizing local font files globally in Tailwind CSS can unequivocally be considered a game-changer when it comes to boosting design efficiency and aesthetics of web applications. The practice has been significantly simplified by means of adding font-face within CSS, which allows the automatic scanning of the files, thereby enabling usage throughout the application without