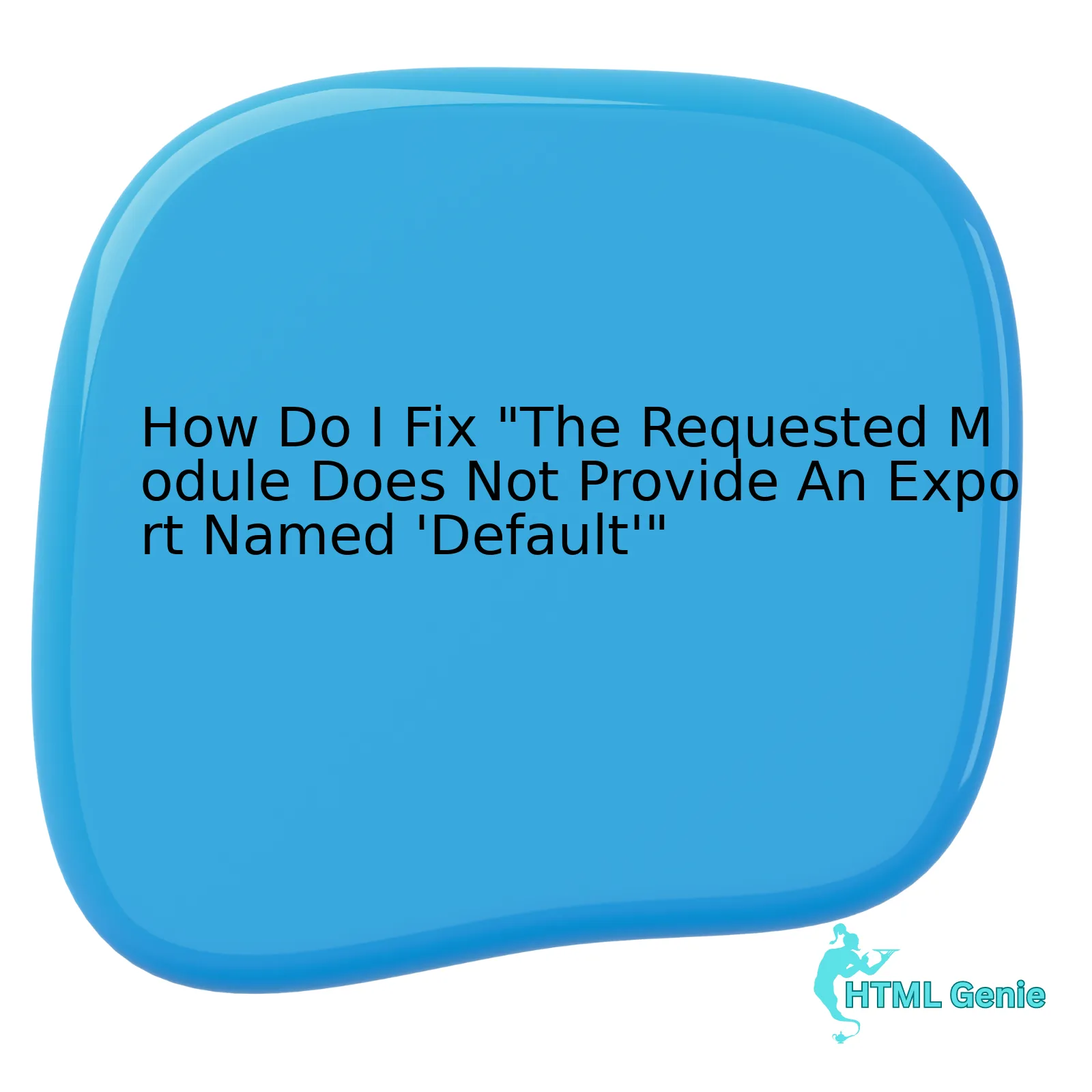
Here’s the table depicting the fundamental steps to fix this problem:
html
Step | Procedure |
---|---|
Identify the Issue | Review your code to confirm if the imported value is indeed a default export. |
Isolate the Code | Find the specific module presenting the issue and isolate it for targeted debugging. |
Rectify the Import Statement | Modify the import statement appropriately, according to whether or not the exported module contains a default export. |
Test the Code | After the changes, test again to be certain that the issue is resolved. |
Firstly, identify the problematic area by reviewing your code. Check if the imported module does actually contain a ‘default’ export. If it doesn’t, then this could be causing the error. For instance, if our module named ‘customModule.js’ doesn’t use default export like `
export default function something(){}
`, but we try to import it with `
import myFunction from './customModule'
`, the error will appear because `myFunction` expects a default export which isn’t available.
Secondly, after spotting the misalignment in code, focus on that specific module, isolate it for precise debugging. Isolation will avoid any misleading errors or messages coming from other parts of the application.
Thirdly, rectify the import statement to match the export type present in the module. If non-default exports are used in the module, the solution is to import as Named imports. This is done using curly braces around the import like: `
import { myFunction } from './customModule'
`.
Lastly, following these modifications, ensure to properly test your code once more to confirm that the error has been fixed completely.
Moreover, perusing [MDN documentation](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/import) on import statements is recommended for better comprehension of default and named imports.
And remember what Linus Torvalds mentioned about coding – “Talk is cheap. Show me the code”. It implies every developer needs to step up and create solutions to problems, just like resolving module export issues.
Understanding the “Module Does Not Provide An Export Named ‘Default'” Error
The “Module does not provide an export named ‘Default'” error primarily occurs when one is working with JavaScript ES6 modules within an HTML setup. It may transpire when attempting to import a feature from a module that has not been exported correctly or perhaps not been exported at all.
import defaultExport from "module-name";
In the above piece of exemplar code, `defaultExport` refers to the feature that you are seeking to import from the concerned module, denoted as `”module-name”`. If this characteristic hasn’t been properly delineated for export in the module file, you will confront the stated error.
As Steve Jobs famously said about technology, “Design is not just what it looks like and feels like. Design is how it works.” This perspective can be quite insightful while troubleshooting this issue; contemplate on the design, the organization of each module, to understand why it isn’t functioning as expected.
To rectify the situation, one needs to consider two primary components: the import statement and the export statement.
1. The **Import Statement**: You should investigate if you’re importing the correct attribute from the exact module.
import { specificFeature } from "module-name";
In the code snippet mentioned, change the `defaultExport` to the particular attribute you desire to import, encapsulated within curly brackets `{}`.
2. The **Export Statement**: Explore the affected module and validate whether the chosen feature has been accurately exported.
export function exampleFunction() { /*function content here*/ }
If your exported element is a function, such as `exampleFunction()`, ensure it is highlighted for export as demonstrated.
Let’s now investigate solving the same problem in the context of incorporating HTML with Node.js. While operating within an HTML and Node.js setup, you’ll have to explore the approach you use for exporting and importing modules.
1. **Verify Adequate Exporting**: The syntax for exporting modules in Node.js differs slightly from that in standard JavaScript.
module.exports = exampleFunction;
If `exampleFunction` was the module you were struggling with, this would be the correct way to promote export in Node.js.
2. **Confirm Correct Importing**: When importing the module, be sure to employ the consistent syntax as shown:
const exampleFunction = require('./module-file-path');
As an additional resource, MDN Web Docs provides [an in-depth guide](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Modules) regarding the utilization of JavaScript Modules. Referencing such reputable coding sources improves confidence and understanding while performing complex operations such as dealing with JS modules in an HTML platform.
Overall, troubleshooting errors like ‘Module Does Not Provide An Export Named Default’ involves scrutinizing both sides of the equation – nothing more than adhering to the classic coding practice of “checking, validating, and refactoring when needed”.
Exploring Causes of the Module Export Named ‘Default’ Issue
The ‘Requested Module Does Not Provide An Export Named ‘Default” error in HTML can be a stumbling point. This issue arises due to the way the JavaScript module system is set up and how it handles default exports. Grasping essential concepts like named and default exports, the difference between `import` / `export`and `require`, and the application of asynchronous loading can prove instrumental in resolving this issue.
The primary cause for encountering this error message is using an incorrect import syntax when attempting to import a CommonJS module. It’s important to remember that importing CommonJS modules often requires different syntax compared with ES6 Modules (ESM).
For instance, if you are trying to import a CommonJS module using default import syntax:
<script type="module" src="app.js"></script>
This will lead to the aforementioned problem because it’s expecting a ESM style export, whereas the module being imported is employing the CommonJS style. Hence, modifying your code to use correct syntax for CommonJS might look something like this:
import * as pkg from 'package';
Instead of:
import pkg from 'package';
Another potential scenario could be if the exported module doesn’t actually define a default export and only named exports. In such case, the structure of the import must match the structure of the export. You’d need to ensure that you’re matching the exact export name from the module.
Here’s an example where the ‘module1’ does not have a default export:
// module1.js export const namedExport = 42; // app.js import { namedExport } from './module1.js'; console.log(namedExport); // 42
Then there’s the potential complication of the “default” keyword itself. If it has been used as the name of a named export instead of its intended special function – which is to represent the “fallback” export from a module. This might trigger confusion and result in the error.
Gaining deeper insight into module systems is critical for avoiding such issues. As Douglas Crockford, renowned technologist and author suggests, “JavaScript is the world’s most misunderstood programming language.” Thus, continuously enhancing our understanding of JavaScript intricacies helps keep such misunderstandings at bay.
Please note that this explanation does not exhaust all possible causes, but these are some quite common scenarios that lead to the ‘Requested Module Does Not Provide An Export Named ‘Default” error. Detailed debugging, revisiting your module structures, or consulting specific documentation may further aid in rectifying this. And always remember to modify your coding style based on whether your environment uses CommonJS or ESM standards.
Methods to Troubleshoot the “Module Does Not Provide an Export named ‘Default'” Error
Fixing the error “The requested module does not provide an export named default” often requires a keen eye and a deep understanding of JavaScript’s ES6 modules feature. If you encounter this error, it typically means that your application is trying to import a method that doesn’t exist in the exported object of the required module. It’s a common pitfall among many developers transitioning into the new ECMAScript serial.
Below are some analytical solutions to correctly address this issue:
– Directly invoke the non-default function from the module:
Para-phrasing Douglas Crockford, renowned JavaScript developer, “JavaScript’s global scope is a public toilet. You can’t spend all of your time in there, but when you have to go… it helps to know what you’re dealing with.”
In line with the above statement, one of the potential solutions involves directly invoking a non-default function or variable from the module. This method is applicable if you’re attempting to import an entity using the `default` keyword while the item was not exported as default during its declaration.
Follow through the provided example:
// original.js export const foo = 'bar'; //app.js import { foo } from './original';
Notice in the code snippet how the `foo` constant was imported without the use of the `default` keyword since it was not exported as such.
– Ensure correct syntax for export default:
Developers often fall victim to erroneous syntax while writing their `export default` statements. In these instances, one quick remedy would be to verify and ensure proper syntax for declaring the default export in the source file.
Example:
// original.js const foo = 'bar'; export default foo; //app.js import myFoo from './original';
In the provided example, observe the `default` syntax in both the export and import statements. Entities declared as `export default` should correspondingly be imported without the use of curly braces {}.
– Misnamed import statement:
Review the spelling and syntax of your import statements. A mismatch could result in the mentioned error. In the programming world, an improperly named identify is akin to dialling the wrong phone number; understandably, nobody answers!
Consider the following cases:
// original.js const johnDoe = 'JD'; export default johnDoe; //app.js import johnDough from './original';
Despite our johnDoe variable having been exported correctly as default, we wrongly attempt to import johnDough, resulting in the aforementioned error.
References:
1. MDN Docs on [Modules](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Modules)
2. Tutorial on [JavaScript Import vs Require](https://www.sitepoint.com/understanding-es6-modules-via-their-history/)
3. CommonJS vs. AMD vs. RequireJS vs. ES6 Modules – [This article](https://appendto.com/2017/06/basics-javascript-module-systems-working-modules/) explains the various JavaScript module systems and other essential technicalities behind them.
4. Book: “Speaking JavaScript: An In-Depth Guide for Programmers” by Dr. Axel Rauschmayer. The section on [ES6 modules](http://speakingjs.com/es5/ch10.html) gives a detailed overview on this topic.
Keep in mind, no error encountered is an ultimate barrier but a gateway to deeper learning. Use these steps laid out above for better outcomes when dealing with such errors. With a deeper grasp of JavaScript ES6 modules feature through diligence and patience, gone will be the days this error haunts your development endeavours.
Pro Tips for Preventing Similar Issues in Future
Understanding and resolving the “The Requested Module Does Not Provide An Export Named ‘Default'” error in contemporary coding environments involves a meticulous focus on module imports and exports. Below are professional tips to prevent encountering similar issues in future:
Comprehend ES6 Import/Export
Firstly, developing an understanding of ES6 module import/export syntax is crucial. Because this error typically arises due to syntax constraints in ES6, it’s paramount to understand how default and named exports work in JavaScript. As Kyle Simpson, the author of “You Don’t Know JS” mentions, “If you’re dealing with modular JavaScript, understanding ES6 syntax is not a luxury, it’s a necessity”.
Utilize Named Exports Accurately
Named exports should be used accurately, whereby the name of the imported module from one JavaScipt file matches the name of the exported module in another.
export const variableName = value;
When importing it in another module:
import {variableName} from './fileName';
Incorrectly using these leads to the aforementioned error.
Exercise Care with Default Exports
For default exports, there can only be one default export per module and no braces are required when importing the default value.
export default variableName;
And while importing:
import variableName from './fileName';
Care must be taken to ensure that these rules are followed to avoid common pitfalls.
Regular Code Review and Testing
Regularly reviewing your code and conducting testing will aid in early detection of potential issues, including import/export mistakes that could cause the error. Tools like ESLint can be invaluable, because they help enforce best practices and spot potential problems before execution.
Continued Learning and Skill Enhancement
Lastly, continuous learning and skill enhancement in JavaScript (especially newer versions such as ES6 and beyond) can preemptively counter these errors. Adoption of industry best practices and staying updated with changes in language specifications will inculcate stability and resilience in your codebases.
Consider these expert tips in establishing a reliable, robust and scalable HTML development environment, minimizing instances of modular malfunction and essentially preventing the “The Requested Module Does Not Provide An Export Named ‘Default'” error.Investigating the error message “The Requested Module Does Not Provide An Export Named ‘Default'” points us to a discrepancy between your ES6 JavaScript code and the set-up of the module you’re attempting to import. This arises when you’re trying to implement a default export, but the module either doesn’t contain one or isn’t configured correctly to allow it.
Fixing this requires a good understanding of how ES6 modules work and are logged by the Node.js runtime. Naturally, remedies can be different based upon the unique circumstances surrounding your problem.
If the assertion is that the faulty module does not offer a default export, it indicates that you are orchestrating an import with syntax intended for default exports where the module export methodology isn’t consistent with that intention.
`
import myModule from './myModule.js';
`
For example, in the script above, the keyword `from` tells your Node.js application to anticipate a default export. Should there be none available, the “export named ‘default'” error occurs.
Subsequently, the initial step should always focus on inspecting the module you’re importing. Ensure it utilizes the correct syntax and adequately sets up a default export. If the module’s exported feature is not default, adjust the import construct to reflect this:
`
import { myModule } from './myModule.js';
`
In the revised code snippet provided, the import statement now adjusts accordingly for named rather than default exports.
Consider the enjoyable complexity of discovering myriad possibilities beneath the surface of “The Requested Module Does Not Provide An Export Named ‘Default’.” The problem is as much about understanding how JavaScript organizes its elements as it is about troubleshooting what went wrong. This task is likened to unfolding a map of potential pathways to illuminate the route that reconnects severed links, mending the code so seams no longer disrupt functionality.
Remember to align your modules, their exports, and how they are imported. Check default and named exports, ensuring each aligns with the corresponding import statements in your main JavaScript file. Doing so will help circumvent such issues and enhance the reliability of your software applications.
As Tim Berners-Lee, the father of the World Wide Web said, “Anyone who has lost track of time when using a computer knows the propensity to dream, the urge to make dreams come true and the tendency to miss lunch”. In similar fashion, while solving programming errors might seem daunting initially, overcoming them through persistent troubleshooting strengthens our coding capabilities.