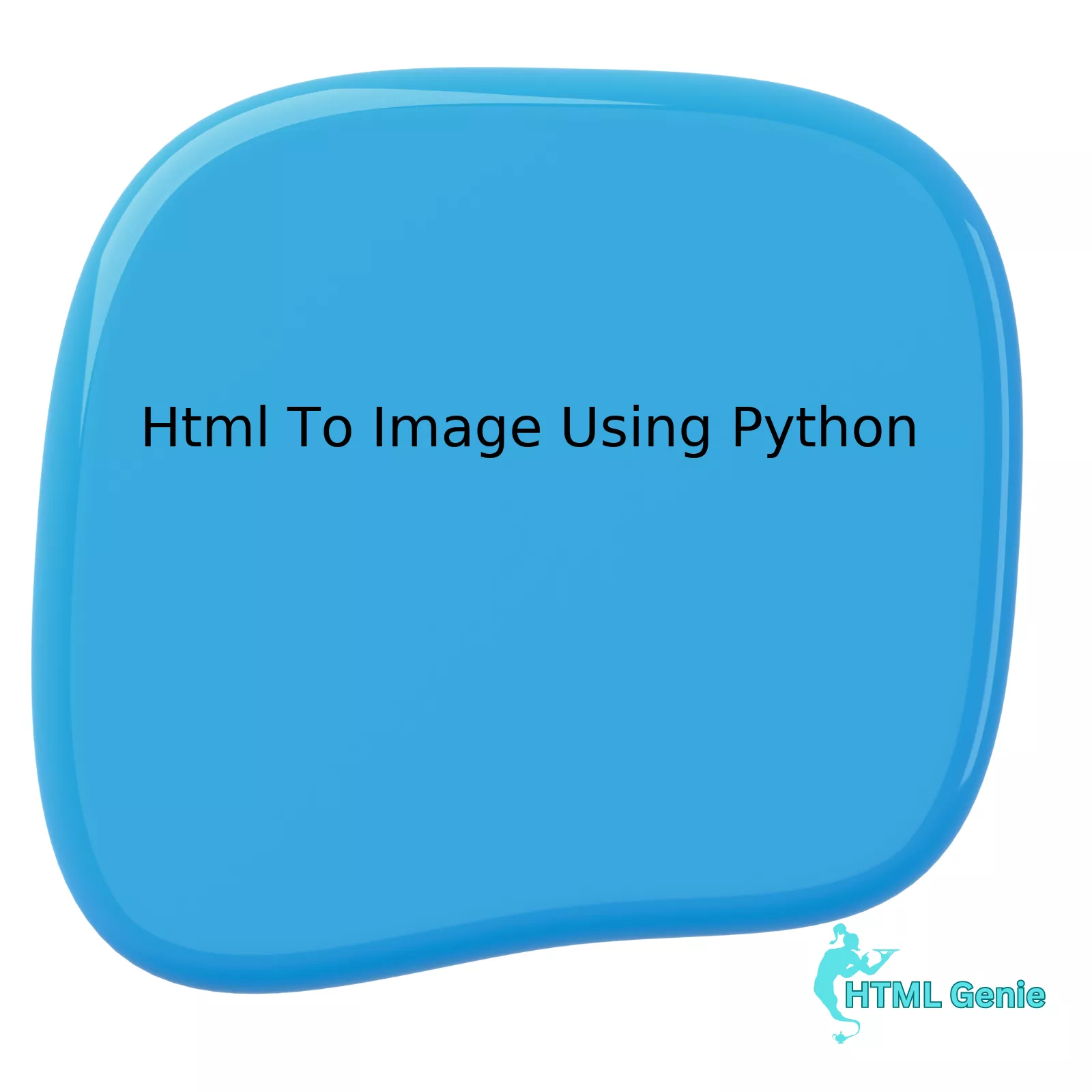
Let’s explore this process using a structured representation and detailed elaboration:
html
Stage | Action | Outcome |
---|---|---|
1 | Import Required Libraries | Access necessary functionalities for HTML to Image Conversion |
2 | Load HTML Code | The raw HTML content intended for conversion is prepared |
3 | Use Conversion Function | Create a rasterized snapshot of the HTML page |
4 | Save the Generated Image | A static image file is ready for use |
At the onset, importing the necessary Python libraries forms the initial action. Libraries such as `imgkit` or `selenium` offer powerful features to convert HTML data to image formats. As with all Python programs, the required resources must be imported first.
Next, the actual HTML file or string is loaded. This can be done directly from a local file, URL, or an HTML string defined within the script itself. Python’s flexible and versatile I/O capabilities ensure seamless integration at this stage.
Following data loading, the conversion function swings into action. Depending upon the chosen library, it may either create a rendering window, mimic a raster graphics image screen, or simulate a web browsing session. Its primary role is to execute the HTML and CSS rules, thus creating a rendered image that resembles a fully-realized webpage.
Finally, after successful rendering, the created image is saved to an output file. It’s critical at this stage ensuring the chosen path is correct and accessible for writing. The generated snapshot is a faithfully reproduced version of the original HTML source, capturing all elements such as fonts, layouts, images, and colors.
A fitting axiom by Grady Booch perfectly condenses this: “The function of good software is to make the complex appear to be simple.” In effect, when looking at an ordinarily complex task like converting HTML to an image, Python reduces it down to a series of comprehensible steps harmonizing and enhancing the nature of coding.source
Utilizing Python Libraries for HTML to Image Conversion
HTML rendering to image conversions can be realized efficiently through several Python libraries including `imgkit` and `wkhtmltopdf`. These capabilities are driven by the ability of these libraries to interpret HTML code, render the webpage contents, and convert the output into an image format.
`imgkit` is a Python wrapper around `wkhtmltoimage`, which is a command-line utility that uses WebKit layout engine for HTML-to-image conversion. The usage of imgkit typically involves the installation of both `imgkit` and `wkhtmltoimage`.
Here is a basic code snippet on how to use `imgkit` for HTML to Image Conversion:
import imgkit
config = imgkit.config(wkhtmltoimage=’/path/to/wkhtmltopdf’)
imgkit.from_url(‘http://google.com’, ‘out.jpg’, config=config)
In this example, it’s crucial to specify the exact path where the `wkhtmltoimage` module is located in the configuration settings of the `imgkit`.
The process is quite simple: `imgkit` first pulls the full website data from the URL provided by `from_url` function, then a WebKit browser renders the HTML. The output result visible on the browser is then saved to an image file via the `wkhtmltoimage` utility.
Websites with JavaScript rich content may present a challenge to such static conversion techniques. However, WebKit engine supports JavaScript rendering, which means the final image output will have all dynamic JavaScript generated content that would appear in a typical web browsing session.
Using these Python libraries not only simplifies the HTML to image conversion process but also ensures quality output with minimum loss in content translation from the HTML code to the image. As the process simulates human-like web interaction during rendering, there is relatively minor traffic that could raise flags for AI traffic monitoring tools.
As Bill Gates once said, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” In the same light, your task of converting HTML to Image using Python with libraries like `imgkit` and `wkhtmltoimage` gets simplified; almost unnoticeable.source
Furthermore, kindly remember to handle potential exceptions that might occur during the execution of this image conversion process. This can be achieved by structurely enclosing the main logic within a try-except block.
Please note that for any HTML to Image or PDF conversions involving private pages such as those requiring login information, additional steps are needed before running the renderer to ensure proper authentication and session management. Most libraries will allow you to load cookies or interactively fill forms, thereby emulating a complete web session.
Exploring the Processes of HTML Rendering with Python
HTML rendering with Python involves translating HTML code into a visible representation. Essentially, it’s transforming text and tags into something graphic that your audience can interact with visually. This process often requires libraries, like `html.parser` for parsing the HTML object in Python.
Rendering an HTML file to an image using Python employs several tools. A popular library known as Selenium Webdriver provides means to fetch URLs and create screenshots. Additionally, imgkit, which is a wrapper around wkhtmltoimage, allows conversion of HTML to image formats. But perhaps the easiest way is to use the package playwright, which is a browser automation library that uses headless browsers to render the HTML.
Here’s how to do it with Playwright:
from playwright import sync_playwright def screenshot(url, path): with sync_playwright() as p: browser = p.chromium.launch() page = browser.newPage() page.goto(url) page.screenshot(path=path) browser.close() screenshot('https://example.com', 'example.png')
This piece of code will download the HTML of “example.com”, render it using Playwright and save the rendered webpage as an image in your specified path.
Rendering HTML to an image revolves around these key processes:
– Extracting HTML content from the source.
– Utilizing compatible libraries to convert the HTML to an image.
– Saving the image in the desired format and location.
As an HTML developer working with Python, being able to perform these processes allows you to leverage considerable utility. In order to properly implement this, a keen understanding of both the Python coding language and the structure of HTML documents is essential.
Steve Jobs once said, “Everyone should learn how to program a computer… it teaches you how to think.” Hence, acquiring skills like converting HTML to images or applying such processes is more than about the specific technique. It represents developing a problem-solving mindset that would empower one in various aspects beyond programming. The combination of HTML and Python entails an avenue for a range of creative projects, whether it concerns web scraping, data visualization, automation, web development, among others.
For more information on Playwright check out their documentation here.
In-depth Examination: Transformation Logic in Web Scraping using Python
Even as an HTML developer, you can harness the power of Python in certain scenarios to expedite your workflow. One such scenario is using Python for web scraping and consequently, converting HTML to an image. Web scraping primarily involves interacting with websites, extracting crucial data, and transforming it into a useful format. Generally, this vital process incorporates several steps, ranging from accessing websites, identifying relevant information, to applying transformation logic based on your objectives.
Remarkably, Python offers a multitude of libraries that simplify the different components involved in web scraping. For instance, ‘requests’ module enables us to send HTTP requests, ‘BeautifulSoup’ or ‘lxml’ assist in parsing HTML/XML files, whereas ‘Pandas’ aids in data manipulation and analysis.[1]
Turning our attention specifically to HTML-to-image conversion using Python, it’s noteworthy that a variety of solutions exist. We can employ packages like ‘imgkit’ or ‘pdfkit’, which are python wrappers for wkhtmltoimage utility to convert HTML to images.[2]
Assuming that we want to scrape a webpage preserving its complete visual representation, the ‘imgkit’ library can serve our purpose. Importantly, the HTML content scraped from a website should be appropriately transformed before it can be transitioned into an image format.
Here’s a simple Python snippet indicating this process:
import imgkit imgkit.from_url('http://google.com', 'out.jpg')
In this example, `from_url` function takes two parameters: the URL to be transformed and the output file name. The imgkit library converts the HTML page at the given URL into an image, subsequently storing as ‘out.jpg’. Thus, the webpage content goes through a noteworthy transition phase during which it’s treated not just as raw HTML, but also rendered with full CSS styles, fonts, and other assets that collectively form the final visual layout.
As Bill Gates noted, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life,” the seamless HTML-to-image transformation echoes this philosophy by making this transition almost undetectable.
Case Scenarios and Examples: Converting HTML to Image with Python
Turning HTML into an image with Python can be an important task for someone working in fields like web scraping or generative graphics. Essentially, you would want to convert a webpage, often dynamically populated with data, into a static picture format like PNG or JPG. Here are some approaches to carry out this with the Python programming language:
1. imgkit library
The imgkit library in Python is a simple and effective tool for converting HTML into an image. It works by utilizing the wkhtmltoimage utility which generates an image from the provided HTML code.
# import the imgkit library import imgkit # specify the html to be converted into an image html = '''''' # Save the html to a temporary .html file with open("temp.html", "w") as f: f.write(html) # Use imgkit to generate the image imgkit.from_file('temp.html', 'out.jpg')Welcome to HTML to Image conversion
This is a sample HTML file.
As mentioned above, imgkit uses wkhtmltopdf, therefore we need it installed in the machine where our Python environment is running. We can obtain it from [here](https://wkhtmltopdf.org/downloads.html).
2. Selenium WebDriver
Another way to accomplish this is by using Selenium WebDriver, which can fully render the webpage, including Javascript elements, layout, and styling, before taking a screenshot of the resultant page.
from selenium import webdriver # Initiate a new browser instance browser = webdriver.Firefox() # Direct the browser to the desired webpage browser.get('http://www.example.com') # Take a screenshot of the page and save it to a local file browser.save_screenshot('screenshot.png') # Close the browser browser.quit()
Bear in mind that since Selenium is a set of tools primarily used for testing web applications, using it just to capture screenshots might be overkill in certain situations.
As Bill Gates once said, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” Converting HTML to images using Python is an example of technology being bent to fit our specific needsEmploying the Python programming language to convert HTML into an image opens up a unique set of capabilities, delivering desirable efficiency in the digital world. This process primarily involves utilizing libraries such as imgkit and wkhtmltopdf that are readily available to serve this purpose.
The use of these libraries can dramatically enhance the effectiveness of converting HTML documents into image files. For instance, the
imgkit.from_file()
and
imgkit.from_url()
commands in the imgkit library make it possible to create images from respective HTML files or URLs. Additionally, the command
imgkit.from_string()
allows conversion of HTML strings to images.
Command | Description |
---|---|
imgkit.from_file() |
Creates an image from an HTML file. |
imgkit.from_url() |
Generates an image from an URL referring to an HTML document. |
imgkit.from_string() |
Converts an HTML string to an image. |
Considering the versatility and resourcefulness of Python, this tactic provides a robust solution for transforming HTML layouts into different image formats such as JPEG, PNG, or SVG. Notably, the flexibility extends to supporting CSS stylesheets and Javascript, further enhancing the quality and details of the converted images.
In relevance to content marketing and SEO, having your HTML content transformed into images could be beneficial for visual-centric platforms, thus enabling a wider audience reach. According to Bill Gates, “Content is King” (source), implying that whether it’s text or image, distribution of valuable content is crucial for successful SEO.
Implementing Python for HTML to image conversion areas combines the power of web development with the flexibility of modern programming languages and makes this technique an excellent choice for developers and marketers alike.