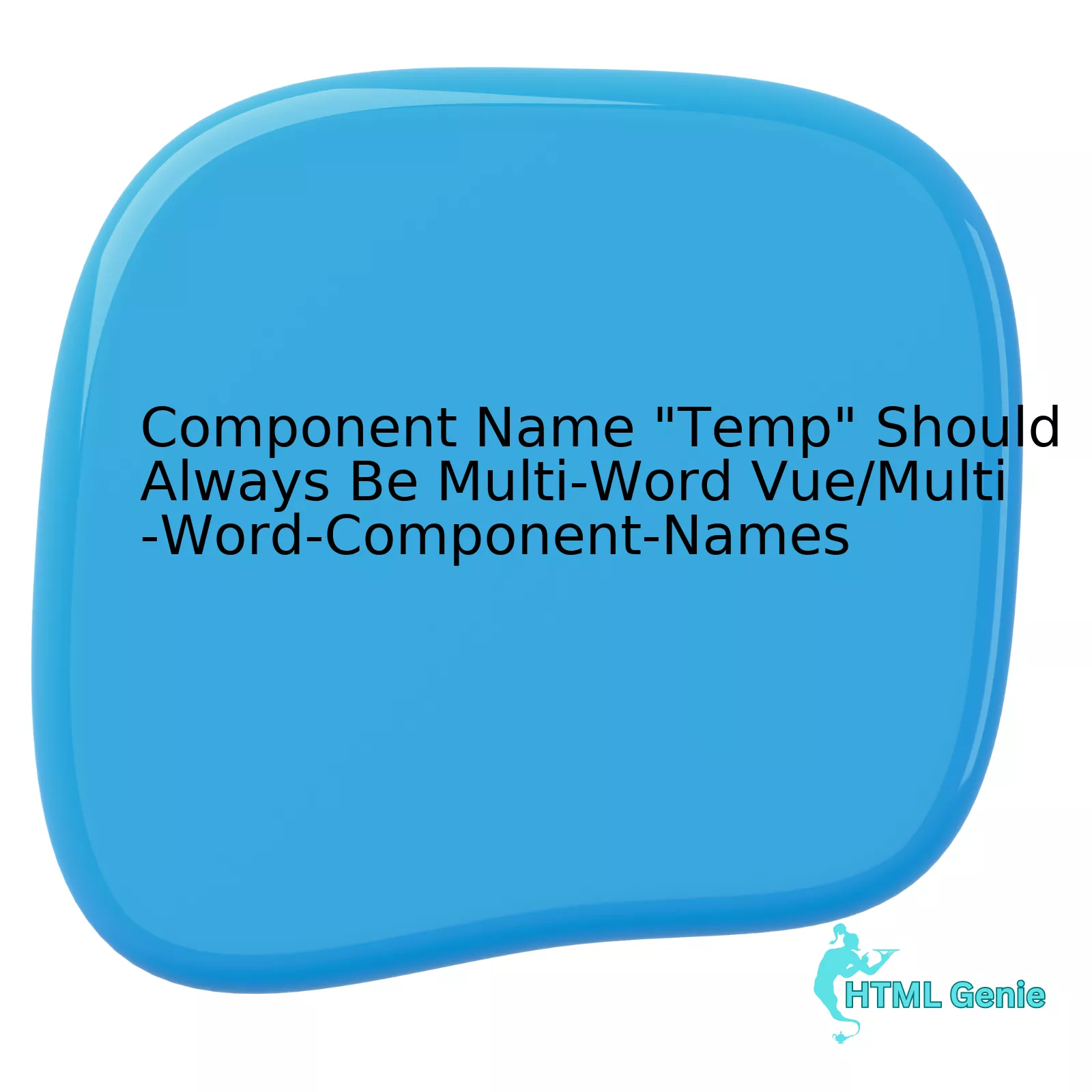
html
Vue.js Component Best Practice
Single Word Name | Impact |
“Temp” | Potential conflict with HTML Elements |
The table above pinpoints potential problems of using single-word names like “Temp”. Used in isolation, these names can easily collide with standard or upcoming HTML elements. Developers must ensure the resilience of their codebase by avoiding such clashes.
Vue.js, an incremental framework for building user interfaces, has prevented this issue against the backdrop that it uses custom elements in areas where the JavaScript framework cannot predict updates. While new elements could be added to the HTML specification over time, selecting unique, descriptive multiple-word names for components minimizes the risk of overlaps.
Complying with this principle, for instance, implies that rather than naming your Vue.js component ‘Temp’, you go for something more complex and definitive such as ‘TemperatureDisplay’ or ‘ClimateReading’. This approach significantly lowers the likelihood of collision with any existing or new HTML tags.
html
<!-- Single-word component name --> <temp></temp> <!-- Multi-word component name--> <temperature-display></temperature-display> <climate-reading></climate-reading>
As noted by Chris Fritz, a member of the Vue.js core team:
“The most important aspect of writing maintainable, easy-to-understand code is clarity. Using multi-word names makes your intent clearer, minimizing chances for confusion later on.”
This guideline not only adheres to the web components specification but also enhances transparency in coding – a cornerstone in software development.
Following good practices like multi-word component names ensures that our Vue.js applications are robust, adaptable, and future-proof. It helps us generate sustainable code, which at the end of the day, is what every developer strives to achieve. References: [Vue.js official guide](https://vuejs.org/v2/guide/components-registration.html#Component-Name-Casing)
[Web Components](https://www.w3.org/TR/components-intro/)
Understanding the Importance of Multi-Word Component Names in Vue
The practice of using multi-word component names in Vue is a significant aspect to take note of when developing applications. The unique and distinguishing feature of multi-word component names is the crucial role they play in preventing overlap with HTML elements, both existing and future ones. According to the official Vue documentation, it is essential that all Vue components should be named with at least two words, with the root App component and Vue’s built-in components being the only exceptions.
Now, let us delve deeper into the specifics of why the name “Temp”, or any single-word naming methodology, might not be as optimal in Vue’s context:
- Avoiding Confusion with HTML Elements: Since HTML does not currently recognize multi-word tags and is unlikely to do so in the future, there would be no conflict between your Vue component and an HTML element.
“Temp”, being a single word, if used as a tag (e.g.,) could potentially collide with future HTML updates.
- Better Readability: Multi-word names aid developers in understanding the intent, type, and functionality of a component just by looking at its name.
A generic name like “Temp” can be ambiguous about the component’s purpose or functionality, making code maintainability more challenging. - Promoting Best Practices: Using multi-word component names reinforce best practices for component registration and name casing in Vue.
Single-word names like “Temp” typically don’t provide sufficient contextual information and may trigger casing-related issues.
As Bill Gates once said, “Measuring programming progress by lines of code is like measuring aircraft building progress by weight”. It’s about establishing efficient, clear and maintainable coding practices rather than simply getting the job done. With this in mind, utilising multi-word component names allows you to achieve clarity and avoid potential conflicts, promoting effective development within your Vue applications.
Let’s look at an example of how “Temp” could be turned into a useful multi-word name. Consider you have a component that temporarily stores user data for a session. Instead of calling it “Temp”, you could go with the more descriptive
. This immediately gives anyone reading your code a clearer idea of what the component does.
In essence, adopting multi-word component names such as “UserSessionTemp” as opposed to single-word names like “Temp,” is a cornerstone of producing qualitatively superior, human-readable, and SEO-friendly Vue.js components.
Exploring Common Mistakes with Single Word “Temp” Components
Developers frequently employ temporary components in their Vue.js code, conventionally naming them with a single, generic term such as “Temp”. While this might seem efficient at first glance, it can lead to several issues that complicate the development process. This prevalent practice contravenes the official Vue style guide’s recommendation for multi-word component names.
- Namespace Clashes: Single-word component names like “Temp” are more prone to conflicts with current or future HTML elements. Browsers inherently approach unknown tags as basic HTML elements, affecting your Vue application’s functionality.
<Temp>
could thus clash with a potential future standard HTML tag named
<temp>
. It’s wiser to utilize multi-word component names to prevent this risk.
- Poor Semantic Meaning: In vue applications, component names offer a high-level breakdown of your application’s structure and logic. A name like “Temp” doesn’t convey specific information about the component’s function or content. Multi-word component names capture much more context, enhancing both readability and maintainability.
- Challenge in Debugging: As applications scale, debugging becomes increasingly challenging with ‘temps’ scattered across your app. Deeper insight into each component’s role is provided by thoughtful, descriptive multi-word names, enabling faster isolation and resolution of glitches.
Let’s review an example demonstrating better practice in-line with Vue’s guidance on multi-word component names.
<template> <UserProfileCard /> </template> <script> import UserProfileCard from './UserProfileCard.vue' export default { components: { UserProfileCard } } </script>
In the quote from Dave Cheney, “Clarity is king in software, and there’s nothing clearer than a well-chosen name.” Remember, choosing a good component name not only adheres to Vue’s style guide but also brings clarity and comprehension for developers, thereby increasing the efficiency of development.
Insights into Best Practices for Naming Vue Components
Vue.JS, an innovative and flexible JavaScript framework, paving the way for efficient and effective web development. The use of a multi-word naming convention for components is a trend consistent throughout Vue’s ecosystem. This practice possesses multiple benefits that extend beyond mere readability and extends into code maintainability and conflict mitigation.
## Why Use Multi-Word Component Names?
There are three primary reasons to adhere to a multi-word component naming convention:
• It helps prevent conflicts with existing and future HTML elements, as HTML’s specification stipulates that all standard tags must be formulated as single words.
• It promotes understanding and clarity within the code base, freely communicating a component’s purpose through its name.
• It supports the scalability of application structure, making it easier to locate and manage individual components within larger projects.
The benefit of maintaining distinct boundary lines between HTML and Vue components becomes apparent when dealing with component names like “Temp.” In isolation, “Temp” can lead to direct overlap with HTML tags or other single-word component names.
## Example
In practical terms, this best practice would see a Vue.js template titled simply ‘temp’ evolve into something more distinct:
<template> <div id="temperature-display"> //... </div> </template>
Avoiding such ambiguities is critical to reducing debugging time and promoting effective communication among developers. Notably, the official Vue Style Guide advocates one-word component names as an error, further establishing the significance of multi-word component naming in this technology domain.
## Iterating Towards A Future-Proof Component Naming Convention
“Every great developer you know got there by solving problems they were unqualified to solve until they actually did it.” – Patrick McKenzie
The solution isn’t about avoiding names like “Temp,” but rather refining them towards distinct multi-word equivalents. By respecting these considerations, software engineers do not merely work on the code – they converse through it. They lessen ambiguities, mitigate potential conflicts, and foster an environment conducive to scalable and sustainable application development.
Realizing the Benefits and Effectiveness of Multi-Word Component Names
Utilizing multi-word component names in Vue presents several benefits, enhancing the effectiveness and usability of complex applications through reduced ambiguity and improved organization. Focusing on ‘Temp’ as a single-word component name, let’s dive into this topic more thoroughly.
HTML elements are increasingly numerous and diverse, encompassing a wide array of built-in tags. Subsequently, a single-word component name like ‘Temp’ is likely to conflict with existing or future HTML element names, causing unnecessary confusion which might result in errors. Multi-word naming strategy could easily fix the potential issue, such as renaming ‘Temp’ to ‘TempComponent’ or ‘AppTemp’.
Already established best practices in Vue development advocate for multi-word component names (See official Vue style guide). The aim being a more semantic, understanding and maintainable code base, which benefits both the developers and end-users in the long run.
<temp></temp> <app-temp></app-temp>
Comparatively, multi-word component names can significantly enhance clarity amid extensive codebases, especially when applied consistently across a project. Each word provides an additional layer of specificity, aiding discernment between components at a glance. For instance, consider ‘TempDisplay’ and ‘TempInput’. Immediately, one can infer that these components relate to displaying and inputting data respectively, simplifying navigation and maintenance within the project scope.
The argument for adopting multi-word component names aligns with Microsoft’s Bill Gates saying, “Measuring programming progress by lines of code is like measuring aircraft building progress by weight.” Just like counting lines of code is a defunct measure of program complexity, using single-worded component names is a poor reflection of thought-through architecture design.
Embracing this standard strengthens cohesiveness within teams and fosters a more intuitive, self-explanatory coding environment optimized for growth and scalability. Bearing these considerations in mind, it stands to reason that ‘Temp’, along with any other single-word component names, should indeed always be articulated as multi-word.Strong adherence to best practices in Vue.js development can significantly increase the efficiency, readability and maintainability of your codebase. One key practice is using multi-word component names, especially for components with a generic name like “Temp.”
Vue.component('temp-component', {...});
The rationale behind this convention stems from the reality that HTML’s specification includes a number of single-word elements. By adhering to the principal of using multi-word component names, developers are able to avoid potential conflicts down the line.
Consider the following:
– **Prevention of Conflicts**: Multi-word component names help prevent conflicts with existing and future HTML elements or custom elements. It helps ensure that your Vue components do not clash with standard HTML tags or those of other libraries.
– **Intuitive Naming Conventions**: Using multi-word component names makes your component names more descriptive and self-documented, which helps to convey their role within the application. This can be particularly valuable when working in large teams where multiple developers may volunteer in the same codebase.
For optimal SEO optimization, you can use kebab-case (hyphen-separated words) for your component names. This follows the standard naming convention for custom HTML attributes as set by W3C. For example, a hypothetical conversion tracking form could be named
'conversion-tracking-form'
rather than just ‘form’ to assure easy comprehension and SEO advantage.
“Programs must be written for people to read, and only incidentally for machines to execute.” – Hal Abelson
The component name essentially should always have more than one word with exception for root App components. Noteworthy is that Vue’s style guide also recommends that strongly. Lower-casing multi-word components provides clarity for developers so they can differentiate between components that mirror native HTML elements, and those that do not.
To summarize, respecting Vue’s recommendation about multi-word names in Vue component enhances the robustness and clarity of your software, which contributes to its ongoing usefulness and integrity. The impact on keyword-rich associated content is another factor favoring multi-word component names from an SEO perspective. It means easier SEO optimizations with more scope of micro-optimization, better crawlability and avoidance of indexation issues caused by single-word names.