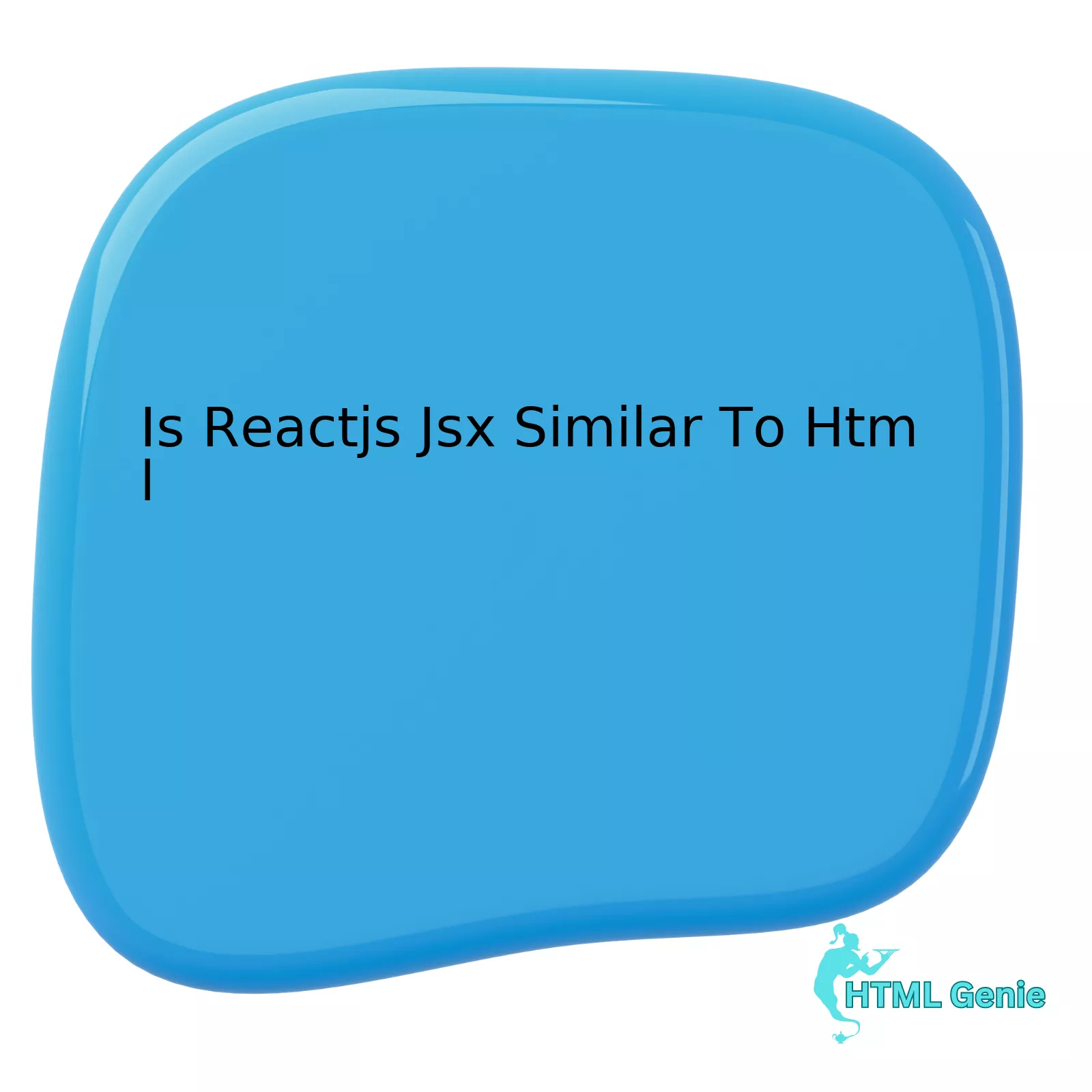
Aspect | Reactjs JSX | HTML |
---|---|---|
Syntax | Close to HTML but not exactly the same. | Defined by W3C, a well-established standard. |
Naming Convention | CamelCase is used for attributes and tag names (for example, className). | Hyphen-separated or lowercase for attributes and tag names (for example, class). |
JavaScript Interactions | Embedded JavaScript expressions directly using braces { }. | Requires separate script tags or linked JavaScript files. |
Self-closing Tags | All elements must close, even those traditionally self-closing in HTML (e.g., img). | Not necessary to close some tags (e.g., img). |
What emerges from this comparison is that Reactjs JSX is similar to HTML in general terms; however, there are some key distinctions. The syntax of Reactjs JSX closely resembles that of HTML, making it convenient for developers familiar with HTML. However, it’s crucial to recognize that while the syntax may appear similar to HTML, JSX is actually closer to JavaScript.
Naming conventions also differentiate JSX from HTML. While HTML uses lowercase or hyphen-separated names for its attributes and tag types, JSX opts for CamelCase. This difference could potentially result in an initial learning curve when switching from HTML to JSX, as developers will need to acclimate to this new naming convention.
In terms of interaction with JavaScript, JSX offers far more flexibility and ease-of-use than HTML. By allowing JavaScript expressions to be embedded directly into its syntax using braces {}, JSX paves the way for dynamic content rendering. By contrast, HTML requires a separation between the markup and the JavaScript code, often necessitating the use of separate script tags or linked JavaScript files.
Further distinguishing feature lies in their handling of self-closing tags. In HTML, tags like ‘img’ don’t require explicit closing – they’re self-closing. But in JSX, every element must be closed, including those that are traditionally self-closing in HTML.
As Steve Jobs said, “Technology is nothing. What’s important is that you have faith in people, that they’re basically good and smart, and if you give them tools, they’ll do wonderful things with them.” Using ReactJS JSX, developers obtain a powerful tool they can leverage to create intricate user interfaces and to ensure a smooth transition between states in a web application. Even though one might liken JSX to HTML due to their syntactical similarity, it’s important to remember that at its core, JSX is a JavaScript-based technology that empowers developers to generate dynamic and complex applications.
Understanding the Fundamental Differences between ReactJS JSX and HTML
Just as it becomes increasingly important to distinguish between related but separate notation and programming languages in web development — like HTML and JSX employed by React.js— a clear understanding of the characteristics that set JSX apart from HTML is crucial.
Differences Between JSX and HTML
While both JSX and HTML share similar syntax with their pairing of tags, they differ significantly in key areas. Let’s explores these fundamental differences:
- JSX is Javascript: JSX is component-centric and part of JavaScript. As such, it can make use of logic processing with JavaScript functions whereas HTML lacks this facility.
// Example: var name='John'; return ( <h1> Hello, {name}! </h1> );
- Case Sensitivity: HTML is case-insensitive, meaning that tag names can be expressed in either uppercase or lowercase letters. Conversely, JSX is case sensitive.
// JSx element <MyComponent /> // Not <mycomponent />
- Referencing CSS Classes: In HTML, we use the ‘class’ attribute to reference CSS classes, while in JSX, we utilize ‘className’, due to ‘class’ being a reserved keyword in JavaScript.
// JSX uses className instead of class: <div className="myClass">Hello, world!</div>
For more information on individual how-to and techniques, refer to React.js official documentation provided here.
As notable coding expert Marco Angelo pointed out: “Understanding the distinctions between HTML and JSX isn’t just good for day-to-day coding tasks, but it gives you a deeper appreciation for the underlying design choices made by development teams.”
Digging Deeper: How ReactJS JSX Transforms into JavaScript Functions
ReactJS JSX finds a remarkable similarity to HTML due to its visually simple and intuitive design. It can often be mistaken for being rendered as HTML; however, this interpretation is unable to grasp the underlying complexity driving the whole process.
To understand how JSX functions and transpires within React.js, knowledge regarding transpilation, babel and JavaScript’s createElement function becomes indispensable.
Transpilation
The usage of Babel plays an instrumental role in transforming ReactJS JSX into vanilla JavaScript. The transpiler (like Babel), takes up the responsibility of converting code written in JSX to ES5 or ES6 compatible JavaScript.
// JSX Code const element =Hello, world!
; // Transpiled Code const element = React.createElement( "h1", null, "Hello, world!" );
Above given is a simple demonstration on how your JSX code dissipates into basic JavaScript using Babel.
createElement Function
The transformation occurs through the ‘React.createElement()’ method. This method churns out objects that seamlessly structure React elements. In simpler terms, whenever a JSX element gets invoked, it translates into a call to the ‘React.createElement()’.
// JSX CodeHello, World!
// Transformed Code React.createElement( 'h1', {className: 'greetings'}, 'Hello, World!' ); // Final Object { type: 'h1', props: { className: 'greeting', children: 'Hello, World!' } }
As visible from the above example, every attribute within a JSX element translates itself into a key:value pair within the object’s ‘props’. CSS classes are denoted using the className attribute instead of ‘class’. Finally, text within JSX tags translate into ‘children’ property of the ‘props’ object.
It is noteworthy to mention American Software Engineer Yehuda Katz’s precis on the remarkable resemblance yet different between computer language and human language – “Language designers want to make programming easier, they have to base it on concepts we already understand well and not require us to map those onto entirely new mental models.”
Thereby emphasizing the need for languages such as JSX, which on the surface level harbors a striking resemblance to HTML, thereby eliminating the steep learning curve for newcomers. Yet, under the hood it offers JavaScript’s full power by transpiling down to pure JavaScript using ‘React.createElement()’.
For further insights on transformations of JSX into JavaScript, I suggest visiting the official React Documentation. Here you get more details on working with JSX and its internals.
Comparing Syntaxes: Highlighting Key Differences between HTML and JSX in React Js
The components of React.js, a popular JavaScript library for building user interfaces, often rely on JSX. JSX, or JavaScript XML, presents the confluence of JavaScript and HTML-like syntax into one language. This merging contrasts yet compares to traditional HTML in unique ways, providing developers with a powerful tool for building complex applications.
One key difference:
HTML is itself a markup language, while JSX is a syntax extension for JavaScript. This essentially means that while HTML describes the structure of web pages, JSX is used within React.js to express HTML-like code directly in your JavaScript code. Here’s an example:
// HTML <div>Hello, world!</div> // JSX let greeting = <div>Hello, world!</div>;
This direct integration of JSX into JavaScript can enhance the usability and efficiency of your code, concepts solidified by Facebook engineer Tom Occhino: “It’s a big win to co-locate your styles, your Javascript logic, your sub-component references in a single file.”
Others include:
– Attribute Naming: HTML uses kebab-case (all lowercase letters with hyphens) for attributing names. However, JSX opts for camelCase. For instance, classname in HTML is written as ‘class’, but in JSX, it becomes ‘className’.
// HTML <div class="box"></div> // JSX let box = <div className="box"></div>;
– Closing Tags: JSX necessitates closing all tags, unlike HTML where some elements (like <input>, <br>, etc.) don’t require explicit closure.
// HTML - fine <img src="image.jpg"> // JSX - error let picture = <img src="image.jpg">; // JSX - correct let picture = <img src="image.jpg" />;
– Expressive Flexibility: JSX allows direct embedding of JavaScript expressions within braces {}, a feature incomparable to static HTML.
let name = 'John'; // JSX let greeting = <h1>Hello, {name}!</h1>;
In contemplation, the differences between JSX and HTML are monumental in shaping their functionality and scope. However, they both maintain a similar syntactical appearance. To truly appreciate these nuances, one must refine their understanding of both JSX and HTML and how they function within different frameworks and libraries. To delve deeper into the subject, the [React documentation](https://reactjs.org/docs/introducing-jsx.html) is the perfect starting point.
In-Depth Analysis: How to Efficiently Utilize React Js JSX in Place of HTML
React.js, a popular JavaScript framework, introduces a syntax extension named JSX that might seem familiar if you’re well-versed with HTML. However, there are key differences and advantages to using JSX instead of traditional HTML in a React environment.
To understand how JSX is leveraged within the React ecosystem to promote efficiency, let us examine some aspects where it excels:
Digestibility: The resemblances between JSX and HTML aid in rendering your components more readable and digestible. These similarities allow new developers to quickly grasp the structure of the component and its implied functionality. As expressed by Mark Zuckerberg, “Code is like poetry — if you can write code effectively, you can likely work mindfully”.
Embedding JavaScript expressions:
Unlike HTML, JSX allows embedding JavaScript expressions inside your UI logic. Wrapped in curly braces, these expressions may include variables, functions, or complex JS logic.
<div>{1 + 2}</div>
Component Composition:
In JSX, HTML Tags are replaced with React Components promoting reusability and clean code. Each `Component` serves as a self-contained entity that manages its state and view.
<NavBar />
Foster Performance:
JSX does not have direct correspondence with actual HTML elements. Instead, it compiles down to React.createElement() calls, facilitating a smooth transition to efficient code compared to traditional HTML strings.
Inline Styles:
Though this isn’t a natural HTML approach, inline styling often becomes necessary while dealing with dynamic styles in React. JSX facilitates this through an object-oriented approach.
<div style={{ color: 'blue' }}>This is a blue text.</div>
Where HTML and JSX differentiate is primarily around attributes such as `className` (vs. “class” in HTML), `htmlFor` (vs. “for” in HTML), and event attribute names (`onClick`, `onChange`, etc.) which follow camel-casing convention.
Granted, there is a slight learning curve when transitioning from pure HTML to JSX, being able to write JavaScript within your markup opens up powerful possibilities, leading several developers to prefer JSX over HTML for React-based applications.
A deeper understanding of the changes introduced by JSX can be found in the official React Documentation.
“As William Zinsser said, writing is a craft that requires both enthusiasm and discipline. With JSX, we get to enforce that discipline in our components, making our code reusable, maintainable, and efficient.”
While HTML and React’s JSX display syntactical similarities, underlying differences set them apart. Both employ a tag-based structure, succinctly segregating different parts of the content — tags for headers, paragraphs, lists, and so forth in HTML, while React employs JSX as its syntax extension.
When comparing the two dialects:
– React JSX carries extra flexibility, utilizing JavaScript power to dynamically render elements based on varying states and even include functions inside tags. When declaring an element attribute in JSX, camelCase convention is favored instead of the hyphen-separated strings typically used in HTML:
<div className="myClass"></div>
– On the contrary, HTML strictly adheres to a static context, primarily serving as a tool for structural layout. Moreover, HTML attributes utilize kebab-case:
<div class="my-class"></div>
Further, rendering child elements differs between the two. In HTML, these are simply represented as additional nested tags. Conversely, React allows expression wrapping in braces({}), permitting dynamic child injection.
React’s virtual DOM provides performance optimization by minimizing actual DOM interaction. However, HTML, inherently binds to the DOM, causing full-page reloads with minor changes, won’t stand up to this efficiency.
Therefore, understanding these discrepancies proves crucial for developers exploring front-end libraries like React, diverging from standard HTML frameworks. The reasons mentioned above should provide sufficient elucidation.
In a fitting quote to summarize the distinguishing points, Brian Holt from Microsoft poignantly stated, “HTML is static because your browser reads HTML and renders it directly, whereas JSX is dynamic because it converts your code into JavaScript, which then generates the HTML.”
Even though HTML and JSX appear similar, treating them as equivalents could lead to misunderstanding of React’s benefits and yield less than optimal development results. Rather, embracing the differences—indeed, the improvements—that JSX offers over simple HTML permits developers to take full advantage of the power and flexibility inherent in modern JavaScript libraries.(source)