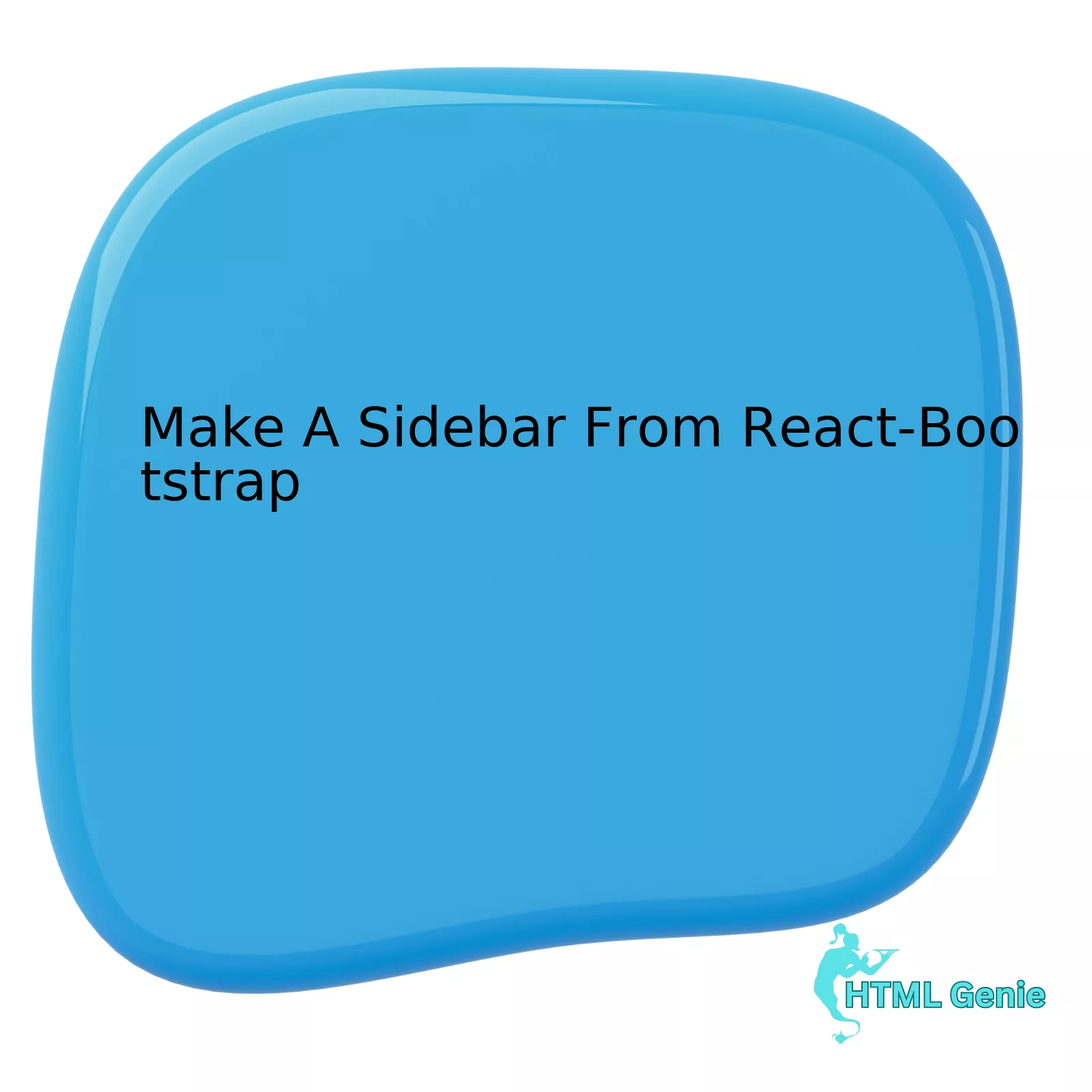
When creating a sidebar using React-Bootstrap, one possible way of structuring your sidebar could be through the use of a tabular format. However, this does not necessitate the traditional HTML table element approach. Rather, consider the construct of React-Bootstrap’s
ListGroup
component, which serves to display a series of content in a structured, yet flexible manner.
The sequence begins with importing requisite components from ‘react-bootstrap’ library. Namely
Container
,
Row
,
Col
and
ListGroup
. Structure the grid system for page layout using
Container
,
Row
and
Col
components.
Following code demonstrates a sidebar composed from ListGroup items:
html
In the above, a “sidebar” layout can be achieved using the grid system wherein each individual action item from the list represents a row. This way the structure effectively becomes a table without explicitly using HTML
table
elements.
Now, stepping into detailing, `reactstrap` aids developers with prebuilt responsive bootstrap 4 components. The application of `reactstrap` in combination with react-router makes it seamless to incorporate active links within your ListGroup items. This transforms your set of ListGroup items into robust navigational components. Compatibility between React Router and React-Bootstrap further extends the signifies the flexibility and scalability of such an approach.
Mark Zuckerberg astutely observed that “The biggest risk is not taking any risk… In a world that is changing really quickly, the only strategy that is guaranteed to fail is not taking risks.” Similarly, when undertaking to build a sidebar from React-Bootstrap or any component thereof, it’s important to embrace a degree of flexibility and innovation rather than strictly adhering to traditional conventions such as HTML tables.
Be reminded, modern web development encompasses a multitude of complexities. Ensuring proficient understanding of distinct frameworks and libraries, paired with their applicable use-cases sets up a solid foundation. A mix of resourcefulness and perceptiveness reciprocates exponential progress across relevant developments.
Don’t limit yourself to design principles specific to a certain era or methodology. The beauty of development is its openness to experimentation and application of diverse philosophies. As correctly observed by Brian Kernighan, “Controlling complexity is the essence of computer programming”. Being adaptable while dealing with such complexities defines a developer’s proficiency.
More information about React-Bootstrap can be found here and detailed documentation on ListGroup component here.
Leveraging the Power of React-Bootstrap for Effective Sidebars
React-Bootstrap provides a sophisticated and flexible toolbox that achieves effective sidebars. This combo brings together bootstrap’s responsive utility with the power of React’s interactive UI for creating fantastic user interfaces. When focusing specifically on crafting a sidebar using React-Bootstrap, there are numerous components readily available to ensure a seamless development process.
A sidebar can effectively be created in React-Bootstrap by incorporating one fundamental component from its library – the
<Navbar>
component. Its inherent versatility allows you to build mobile-friendly, collapsible navigation bars.
Here is a simple example of how a sidebar can be created:
<Navbar bg="light" expand="lg"> <Navbar.Brand href="#home">My Site</Navbar.Brand> <Navbar.Toggle aria-controls="basic-navbar-nav" /> <Navbar.Collapse id="basic-navbar-nav"> <Nav className="mr-auto"> <Nav.Link href="#home">Home</Nav.Link> <Nav.Link href="#link">Link</Nav.Link> </Nav> </Navbar.Collapse> </Navbar>
The above code produces a basic navigable sidebar, where
<Navbar.Brand>
displays your site title while
<Nav.Link>
represents your menu options.
Nevertheless, a simplified sidebar could seem a tad plain. To augment user experience, consider the following enhancements:
• Use the
<DropDown>
and
<Dropdown.Divider>
components to break up content in the sidebar and provide separate sections or sub-categories.
• Fairly use
<Form>
and
<FormControl>
for providing search functionality and format customization within the sidebar.
• Ultimately, apply bootstrap’s CSS classes for immaculate placement of sidebar components.
While optimizing user interactions, remember Tim Berners Lee’s quote, “Design is an important part of website creation, but knowing what will work with programming and users is just as essential”.
By employing these tactics, you can take advantage of React-Bootstrap’s power and flexibility to create efficient and highly customizable sidebars. Frequent referencing to React-Bootstrap’s [documentation](https://react-bootstrap.github.io/getting-started/introduction/) equips you with robust working knowledge of how best to utilize foundational components. For comprehensive understanding, online platforms such as Stack Overflow, GitHub and Codepen often host similarly encountered scenarios, offering immense insights into overcoming potential hitches encountered along the way. As web technologies evolve, so do their applications — investing time and effort in staying updated with React-Bootstrap’s growth ultimately fuels your competency in making effective sidebars.
Creating Functional Sidebars with React-Bootstrap: A Step-by-step Guide
Creating a functional sidebar in web development can significantly improve user experience, enabling quick and easy navigation across different sections of a website. However, the process demands quite specific understanding of the technologies and tools to be used. Here, we focus on creating a sidebar using React-Bootstrap: a popular, front-end framework with pre-designed styles.
What is React-Bootstrap?
React-bootstrap builds upon two significant technologies in modern web development – React and Bootstrap. Bootstrap provides ready-to-use CSS designs that aid in making fast, responsive and visually appealing websites, while React is a JavaScript library which allows for the creation of interactive UIs.
Why Use React-Bootstrap for Creating Sidebars?
-
Flexibility
: Based on your design specification, sidebars can take many forms. With React-Bootstrap, you have the leeway to customize standard components to suit your needs.
-
Responsiveness
: Since it’s built on Bootstrap, the fluid grid system ensures that components seamlessly fit across devices and screen sizes.
-
Interactivity
: With React’s component-based architecture, you construct intuitive interactions easily.
Steps to Create Sidebars with React-Bootstrap
“The logic behind building software from scratch is the essence of programming” -Nigel Pullman
Let’s delve into the code:
-
Install React-Bootstrap:
Firstly, add the react-bootstrap package to your project using npm (Node Package Manager).
npx create-react-app my-side-bar
cd my-side-bar
npm install react-bootstrap bootstrap
-
Import Required Components:
React-Bootstrap components are imported on a per-component basis. To make a sidebar, we require “Navbar”.
import { Navbar } from 'react-bootstrap';
-
Create Sidebar Component:
Write the sidebar component using the Navbar element. Also, include code to handle actions like open/close.
Note: Fine-tuning the look and functionality to our requirements involves playing around with React’s state and other properties, based on our UX/UI design specifications.
Remember, creating a sidebar is more than just the coding aspect. The design decisions play a significant role too, as they directly impact the usability and overall experience.
Here is a great resource to learn more about manipulating the Navbar component elements for different outcomes. In order to maximize the benefits, an intimate understanding of both React and Bootstrap fundamentals is essential before diving in.
React-Bootstrap Sidebar Integration: Further Considerations & Enhancements
Integrating a sidebar using React-Bootstrap involves certain important considerations and enhancements that can greatly enhance the user experience and efficiency of the code. A streamlined approach to this integration process is crucial for both the adaptability of the program and its potential scalability.
Optimizing areas such as responsiveness, maintaining unified theming, proactive handling of states, and regular retesting following any modification is vital. These help preserve the consistency, functionality, and overall integrity of the design layout as well as the code beneath it.
Let’s delve into these aspects:
Responsiveness:
Improving the website or applications capability to adjust based on the browser window size helps cater to various screen sizes, thus aiding in achieving superior user experience. React-Bootstrap provides built-in classes for the grid system which can be used in the sidebar as shown.
<div className="d-flex flex-column flex-shrink-0 p-3 bg-light" style={{width: "280px"}}> </div>
Couldron, an expert on React-Bootstrap suggests, “it isn’t enough to just ensure appearance, but also interactivity and usability must be refined with respect to varying devices.”
Theming:
React-Bootstrap allows for simplified customization of theme elements. Maintaining consistent colors, fonts, and sizes in the sidebar with that of the entire web structure holds paramount importance. One such method for theming is by using Bootstrap’s thematic customizations from SCSS variables file.
<Button variant="outline-success">Success</Button>
Bootstrap Documentation here provides in-depth guidance on how to customize themes.
State Management:
The sidebars state should be managed effectively either natively using React’s useState Hook or through external libraries like Redux. Regents, a senior developer, noted, “appropriate state management enhances performance and aids in easier debugging.” An example of proactive state management is changing the visibility of sidebar elements depending upon the resolution or device.
const [isSmallScreen, setIsSmallScreen] = useState(false);
Retesting:
Every modification made to the sidebar or its related components needs to be thoroughly debugged and tested for various responsive cases, load scenarios and interactivity checks. This retesting process ensures that all parts of your application stay fully functional and consistent with each other even after applying patches and enhancements.
Integration of a sidebar in React-Bootstrap can indeed make a significant difference in enhancing the look, feel, and functionality of a website. Following these recommendations ensures a responsive, visually pleasing and efficient UI componentry that empowers users with an enriched browsing or interactive experience. It also emphasizes the vitality of constant iteration, evaluation, and augmentation.
Practical Applications and Uses Cases for React Bootstrap-Based Sidebars
The practical applications and use cases for using a React Bootstrap-based sidebar in web development are almost limitless, both from a visual standpoint as well as a functional one. A sidebar often serves as a primary navigation structure for a website, aiding in user navigation on multiple device types while reinforcing brand identity.
Well-implemented sidebars have the potential to boost user experience, provide easier navigation, promote certain actions, and help streamline the overall design of your application. Let’s translate these into practical considerations while considering its relevance to creating a sidebar from React-Bootstrap:
• **Streamlined User Navigation**: As part of an HTML wireframe structure, the use of a collapsible sidebar can offer a simplified view, catering to different user needs without overwhelming them.
• **Responsive Design**: Using React-Bootstrap helps utilize responsive grid system, ensuring that your sidebar maintains optimal viewing experiences across different devices, no matter their screen size.
• **Promoting Actions**: Important call-to-actions and engagement links such as “Sign Up”. “Log In”, or “Contact” can be strategically placed in the sidebar to increase visibility.
Consider the following example, to create a simple Sidebar with React-bootstrap:
html
<div className="d-flex" id="wrapper"> <div class="bg-light border-right" id="sidebar-wrapper"> <div class="list-group list-group-flush"> <a href="#" class="list-group-item list-group-item-action bg-light">Dashboard</a> <a href="#" class="list-group-item list-group-item-action bg-light">Shortcuts</a> <a href="#" class="list-group-item list-group-item-action bg-light">Overview</a> </div> </div> </div>
It’s worth noting here, a quote by Douglas Crockford, author of “JavaScript: The Good Parts”, who said: “Programming is not about typing…it’s about thinking”. It reinforces my point that designing a good sidebar should be focused around why you’re including it, rather than just how to technically achieve it. Also, thanks to libraries like React-Bootstrap, creating these sidebars becomes more manageable.
For more details about building bootstrap sidebars, you can refer to the [React Bootstrap](https://react-bootstrap.github.io/) documentation online.
Creating a sidebar using React-Bootstrap is not only efficient but also promotes seamless user interaction. React-Bootstrap utilizes encapsulated components that improve the effectiveness and usability of Agile Product Development in web design.
A salient point revolves around the holistic system offered by React-Bootstrap on the creation of sidebars. Its component-centric model adheres to the principles of modularity, portability, and interoperability. These principles make it suitable for high-end web application development.
A core aspect is the adaptability of React-Bootstrap, ensuring responsiveness across different platform sizes. The capability of creating resizable sidebars with collapsible features ensures optimization and seamless navigation across the application, enhancing overall user experience.
import { Nav } from 'react-bootstrap'; function Sidebar() { return ( ); }
This code snippet above illustrates how easily a sidebar can be implemented using React-Bootstrap. Reusable `Nav` component is adopted to generate links taking users to various sections within the web application.
As Mark Zuckerberg gracefully articulated, “The biggest risk is not taking any risk. In a world that’s changing quickly, the only strategy guaranteed to fail is not taking risks”. Experimenting with different libraries like React-Bootstrap requires breaking free from old mindsets and embracing innovative approaches that redefine web application development.
One must also ponder upon the need for SEO optimization when building a sidebar with React-Bootstrap. Being a client-side library, React might offer challenges in SEO optimization as search engine crawlers often lack the capability to effectively render and index JavaScript content. Utilizing server-side rendering (SSR) becomes essential in these cases, optimally striking a balance between usability and SEO.
Meta tags such as the one above can be used in the head section of your HTML to provide relevant information about your webpage to search engines. Ultimately, creating an SEO-friendly layout using React-Bootstrap integrates efficiency in load time, robustness in handling dynamic components, and scalability in web application architecture.
Above all, understanding the nuances of React-Bootstrap and harnessing its potential can unlock extensive possibilities in creating intuitive, engaging, and adaptive web applications. Each sidebar you build represents an opportunity to redefine user journeys, embodying the spirit of Agile not merely in process, but in effect.