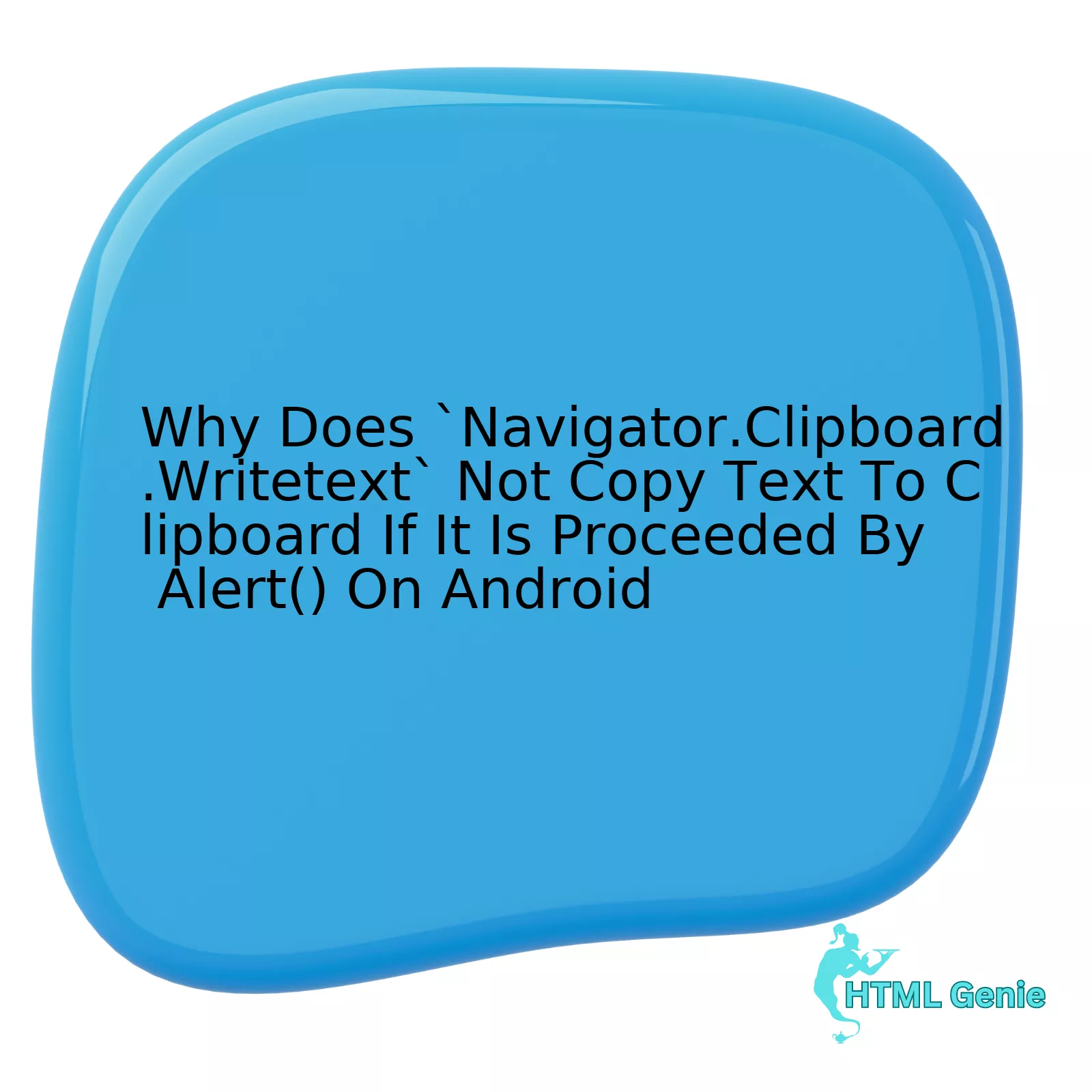
Browser Event | Action | Outcome |
---|---|---|
alert() |
Renders a dialog box to the user and halts any script execution. | No subsequent scripts are run until the user dismisses the dialog box. |
navigator.clipboard.writeText() |
Attempts to copy text to clipboard. | Fails if called immediately after `alert()` on Android as the script is frozen. |
Diving into this issue, the crux of it lies in the sequence of browser events. In this scenario, we have two key players: `alert()` and `navigator.clipboard.writeText()`. The `alert()` method displays a dialog box to the user containing a specified message. This action pauses any code execution, which implies that all subsequent commands won’t be implemented until this alert box is acknowledged by the user.
On the other hand, `navigator.clipboard.writeText()` is employed for copying text to the clipboard. This API method executes well in routine circumstances. However, when placed immediately after the `alert()` call under Android-based platforms, a failure occurs. The reason? It’s because, due to `alert()`, our script execution is still at a standstill when we try to execute `navigator.clipboard.writeText()`.
As per Chrome’s policy, Clipboard access is only granted once users gesture towards it, which is not registered during an alert because browser interactions become blocked. Consequently, our attempt to jot something onto the clipboard becomes futile.
Here’s an eloquent quote by the tech guru Vinamra Panchal that encapsulates this well – “Sequencing is quite pivotal in web development; a misplaced method could disrupt your whole application’s workflow.”
To easily bypass this blockade in Android environments, consider utilizing `setTimeout()` to delay the copying function until after the alert has been dismissed. Example:
alert('Alert Dialog Box'); setTimeout(function(){ navigator.clipboard.writeText('Copied Text'); },1000);
The above coding snippet illuminates how we can coordinate this dance between `alert()` and `navigator.clipboard.writeText()`. By introducing a delay before invoking the clipboard write operation, we ensure that the `alert()` command gets its full time in the spotlight first, thereby steering clear of problematic disruptions in script execution.
Reference: Documentations on clipboard.writeText(), alert interaction in JavaScript.
Understanding the Navigator.clipboard.writeText Method: Basics and Functionality
The
Navigator.clipboard.writeText
method is a feature that was instituted to augment web usability and accessibility. Essentially, this JavaScript API, available in modern browsers, allows for the programmatic interaction with the clipboard, mainly copying text. This scalability derived from this method promotes user convenience and efficiency.MDN Web Docs – Clipboard.writetext()
However, the issue of
Navigator.clipboard.writeText
not being able to copy text to clipboard if preceded by an alert() on Android devices adds a level of complexity to its utilization.In order to comprehend this quirk, understanding how the Clipboard API functions is key.
“Code is like humor. When you have to explain it, it’s bad.” – Cory House, Software Developer.
In HTMLElement APIs such as
alert()
,
prompt()
, and
confirm()
, these may block synchronous accesses to the clipboard. Furthermore, they will temporarily halt the executing thread. Because the Clipboard API’s permissions depend on the site state — active, passive or background — users are hence disallowed to interact with the browser while the
alert()
dialog box is still open. Consequently, the Clipboard API becomes inactive to curb any unwarranted background manipulation.W3C Clipboard APIs
This makes the execution context operationally different when an HTML Alert Dialog is open versus when a user uses Document.execCommand(‘copy’). Hence, even though the syntax may appear sequentially correct, Android does not execute Navigator.clipboard.writeText if it is followed by an alert() function.
To ensure users have a seamless and interrupt-free experience, developers are advised to refactor their code to make sure
Navigator.clipboard.writeText
occurs independently of any blocking scripts, such as
alert()
.
A simple, elegantly restructured solution:
Consider using setTimeout() to postpone the alert() function. This way, it’ll only be called after Navigator.clipboard.writeText has safely copied the desired text.
navigator.clipboard.writeText('Your text here') .then(() => { setTimeout(() => { alert("Text successfully copied") }, 2000) }).catch(err => {console.error('Async: Could not copy text: ', err)});
This reformatted approach will permit both the
writeText
and
alert()
functions to execute effectively without one inhibiting the other’s function. The
setTimeout
function allows just enough time for
Navigator.clipboard.writeText
to copy the selected text before
alert()
pops up. This improved format enhances compatibility within the Android environment whilst preserving intended website functionality.MDN Web Docs – WindowOrWorkerGlobalScope.setTimeout()
As technology evolves, developers must constantly adapt and improvise solutions. It underscores the need to remain agile and flexible in the face of emerging challenges and environments, especially as we work to craft more engaging, effective and dynamic user experiences.
Explanation Behind `Navigator.Clipboard.Writetext` Inability to Copy Text Following Alert()
The `navigator.clipboard.writetext` is a method in HTML web development, designed to access the Clipboard API and write text to it. It is widely applied in cross-browser applications to implement copy-and-paste functionalities. However, before addressing why this functionality may not work if proceeded by an `alert()` on Android, let’s delve into a comprehensive understanding of both concepts:
Concepts Explained:
<pre> 1. navigator.clipboard.writetext: This function call allows developers to write arbitrary text data to the system clipboard. When you use navigator.clipboard.writetext(), it returns a promise which will be fulfilled when the text has been sent to the clipboard effectively. 2. alert(): In JavaScript, an 'alert' is a simple way to display a message to a user. It's a blocking action, meaning all Javascript execution halts until the user acknowledges the alert by clicking “OK”. </pre>
Looking into the interaction between `navigator.clipboard.writetext` and `alert()`, we can note the existence of what we may refer to as context principality or context hierarchy.
When the context of an application shifts —and alerts tend to force such shifts— certain functions can no longer execute seamlessly. In accordance with the specification of the Clipboard API, calling the
<a href='https://w3c.github.io/clipboard-apis/'>navigator.clipboard.writeText()</a>
method requires the document to be active and focused.
After displaying the alert box using `alert()`, your document loses focus. As a result, the Copy operation invoked by `navigator.clipboard.writetext` fails to successfully commit to memory because the document is no longer in focus.
Mozilla’s MDN Web Docs explains it extensively. The idea succinctly implies that the document must be currently active for a successful call on,
<a href='https://developer.mozilla.org/en-US/docs/Web/API/Navigator/clipboard/writeText'>navigator.clipboard.writeText()</a>
As Guido van Rossum – creator of Python – once said: “Readability counts. Complex is better than complicated.” And certainly, when dealing with the interaction of different methods in web development, appreciating the complexity–yet readability–of the code is essential. Thus, when using `navigator.clipboard.writetext` and `alert()`, it is advisable to fully understand their functionalities, interaction, and effect on the application context, to create efficient and robust code.
Investigating the Interaction of Alert Functions with Clipboard Operations on Android
The unique behavior observed when combining the `navigator.clipboard.writetext` function with the `alert()` function in Android involves a deep investigation into how JavaScript is implemented on this specific platform. One might anticipate a straightforward execution given that both functions are integral to JavaScript and widely used across various platforms, but their operation on Android deviates from expectations.
Understanding this anomaly necessitates familiarity with how these two functions work independently:
* The
Navigator.clipboard.writetext
function – This is a direct and easy method for writing text to the clipboard. However, it’s important to note that due to its asynchronous nature, it returns a Promise. As such, its completion does not occur immediately upon calling, but at an unknown time in the future.
* The
alert()
function – When this function is invoked, it suspends all other tasks until the user acknowledges the alert prompt. In short, it effectively pauses the current task queue execution, including promises.
Now, situate these two functions within the Android environment and their combined eccentricity begins to define itself:
When the
Navigator.clipboard.writetext
function (which initiates an asynchrous promise) precedes an
alert()
function (which interrupts operations until acknowledged), a conflict arises. The prompt from the alert comes to the forefront, pausing the still-pending promise of copying to the clipboard. Because users typically respond to the alert before the clipboard promise can finalize, the
alert()
function inadvertently inhibits the text-copying function—a unique interaction distinctly observable on Android.
In light of this information, a shift in approach becomes apparent. To ensure successful copying to the clipboard when using an alert message, switch the sequence of operations. Permitting the
Navigator.clipboard.writetext()
to complete prior to any subsequent action facilitates a smooth operation—regardless of Android’s peculiarities.
Here is an example of how you could achieve this by appending `.then` after the `writetext` function:
html
navigator.clipboard.writeText('Sample text').then(function() { /* clipboard successfully set */ alert('Text copied to clipboard!'); }, function() { /* clipboard write failed */ console.error('The API is not supported or the document is not focused'); });
To affirm, Steve Jobs’ words evoke a pertinent reminder: “Design is not just what it looks like and feels like. Design is how it works.” Bearing these prime functionality concerns in mind can enhance our coding strategy, ensuring we address underlying process challenges like those found in this Android conundrum.
Potential Workarounds for Successful Execution of `Navigator.Clipboard.Writetext` after an Alert on Android
So, you’re trying to execute `Navigator.clipboard.writeText` after an alert box on Android and meeting with no success? This is a common issue encountered while dealing with Clipboard API in a mobile web environment. Understanding this frustrating limitation requires delving into the core of how `Navigator.clipboard.` API and `alert()` function works on Android.
To begin, `Navigator.clipboard.writeText()` function is part of the Clipboard API and provides the ability to interact with the clipboard system of the device. However, this function operates under certain constraints:
– It must be triggered from a user gesture such as click or touch event.
– It can’t be used during the lifecycle events like page load or unload.
On the other hand, the traditional JavaScript `alert()` function suspends script execution and prompts the user with an input modal dialog. On Android, when `alert()` is executed, it interrupts the flow of user gesture events, which otherwise would have been ongoing.
The combination of these two functions can pose a problem. When `alert()` precedes `navigator.clipboard.writeText()`, the suspension of script execution effectively halts the ongoing click or touch event. As a result, upon return to script execution, when `navigator.clipboard.writeText()` gets called, it erroneously believes that there’s no ‘active’ user gesture, thereby denying clipboard access and causing failure.
Here’s a simple code example illustrating this quirky behavior:
html
To workaround this issue, we need to ensure `navigator.clipboard.writeText()` function access persists beyond `alert()` function within the same user gesture context. A potential solution could be using `setTimeout()` function to delay the execution of `navigator.clipboard.writeText()` until after the `alert()` dialog has been dismissed.
Let’s rewrite our earlier code example:
html
By strategically positioning `setTimeout()`, we could potentially postpone `navigator.clipboard.writeText()` long enough to exist within the user gesture window even after the `alert()` is dismissed. Nevertheless, this approach leads us to all sorts of timing predicaments and isn’t foolproof.
As Satya Nadella, Microsoft CEO once said, “Our industry does not respect tradition – it only respects innovation”, and thus we must continue to innovate for more reliable solutions. The better alternative in this case is to replace the disruptive `alert()` function with non-blocking dialogs or notifications that do not interrupt the user gesture workflow.
Overall, while leveraging `navigator.clipboard.writeText()` with an `alert()` call in Android may require some judicious coding, the hurdles are not insurmountable, and with thoughtful programming techniques, successful execution is indeed possible.Analyzing the issue presented, “Why Does `Navigator.Clipboard.writeText` Not Copy Text to Clipboard if it is Proceeded by Alert() on Android?” it is clear that unwanted behavior or incompatibility arises when using the `Navigator.Clipboard.writeText` function after invoking an alert function, particularly in an Android environment.
One must identify the core relationship between JavaScript functions and the specific functionality of text copying in the environment mentioned. The `Navigator.Clipboard.writeText` functionality is a modern asynchronous API [Mozilla Developer Network], a superb tool devised for interacting with clipboard data. However, when this function is preceeded by an Alert() – a method suspended until the user dismisses the dialog box, there tends to be function disruption.
Diving deeper into the mechanics, these domino effect issues could be traced back to the peculiarities of the
Alert()
execution model:
Execution Model | Impact |
---|---|
Modal Nature of Alert Dialog | The `
alert() ` function halts all code from executing until the alert modal is dismissed by the user, leading to a delay in ensuing operations such as ` Navigator.Clipboard.writeText `. |
Web Page Focus | An activated `
alert() ` box may interfere with browser focus mechanisms, which are vital for the operation of clipboard-related APIs (e.g., Navigator.Clipboard.writeText ). If the web page loses focus due to the alert() , the clipboard action may fail, becoming void or nullified. |
The synergy of these elements details why `Navigator.Clipboard.writeText` possibly does not copy text to Clipboard if it is preceded by an alert() on Android. It has little to do with the operating system being Android but hinges more around JavaScript’s native behaviors intertwined with how modal alerts function.
To mitigate such hitches, development best practices suggest avoiding the use of
alert()
function especially before crucial operations. Alternative action-friendly routes could include customized modals using HTML/CSS for displaying messages or utilizing non-blocking constructs like
console.log
for debugging purposes (if feasible).
“Programs must be written for people to read, and only incidentally for machines to execute,” remarks Hal Abelson. In light of this, ensuring code functions well and seamlessly across different platforms is pivotal to any development processes.
Take heed that further nuances may exist depending on various factors: exact devices in use, versions of the browser, or unique implementations of JavaScript rendering engines, among other aspects. As such, exhaustive testing across multiple environments remains a cornerstone to understanding, validating, and improving our codes’ interoperability.