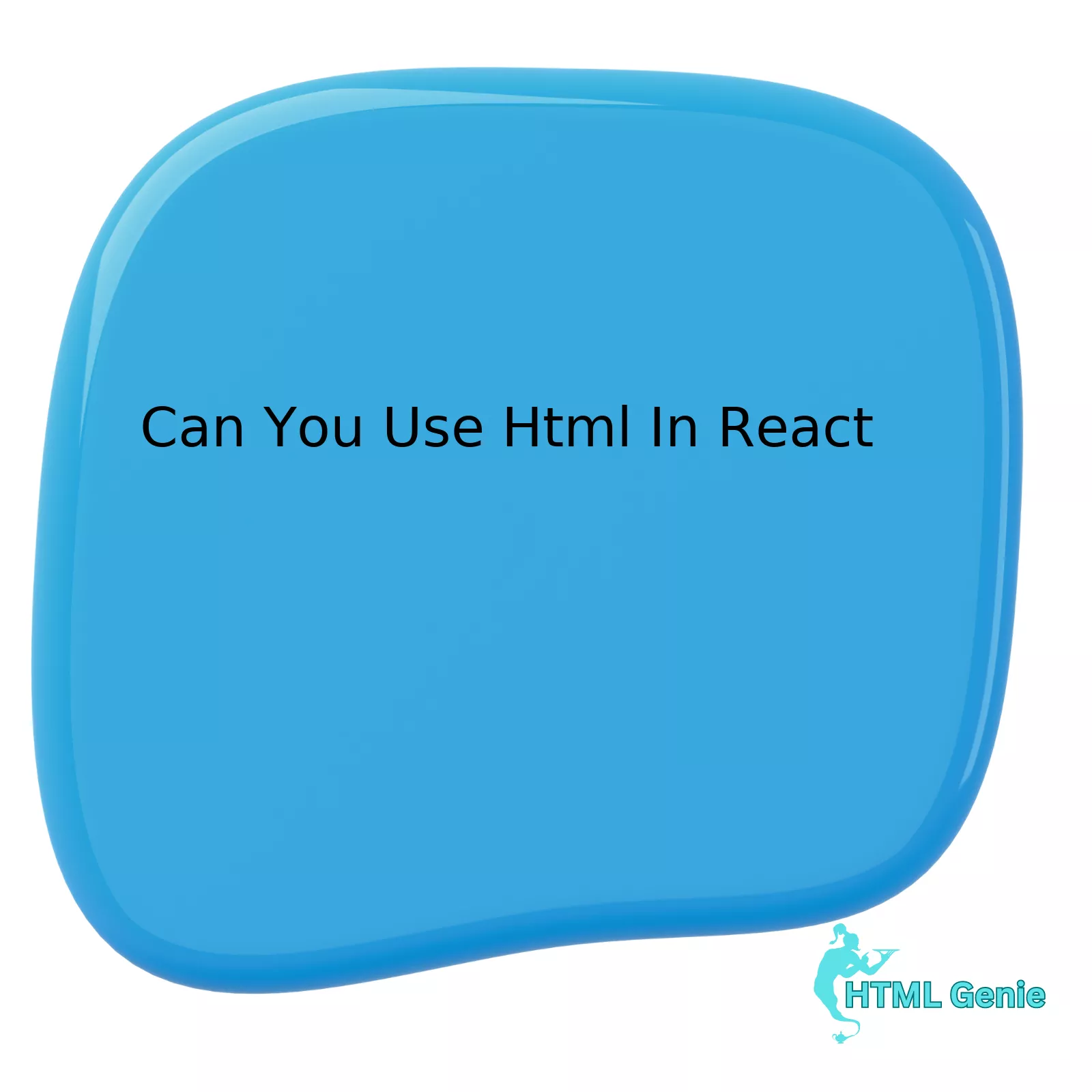
A simple comparative snapshot between using HTML in typical web development and JSX in React can be explained via the comparison table below:
Framework | Syntax | Description |
---|---|---|
Typical Web Development | HTML | Standard markup language for creating web pages and web applications. |
React | JSX | A JavaScript syntax extension allowing for writing JavaScript that resembles HTML. It is transformed into regular JavaScript at runtime. |
While the above tabular representation primarily differentiates HTML use in typical web development from JSX in React, it’s also worth delving a bit deeper into why JSX is preferred when crafting UIs with React.
In essence, React is a JavaScript library, which means it fundamentally works with JavaScript objects and data structures. JSX allows developers to write what seems like straightforward HTML while really writing JavaScript, providing an easier way to manage and create elements in React. To put it differently, rather than programmatically creating elements and then appending them to the DOM, as is done with vanilla Javascript, developers can simply write JSX that looks very much like HTML, and the React library will take care of the rest.
An important note is that there are several key differences between traditional HTML and JSX. For instance, while HTML uses the ‘class’ attribute, JSX uses ‘className.’ Additionally, because JSX is closer to JavaScript than HTML, non-string attributes must be placed in curly braces {}. Here’s an example of a piece of JSX code in a React component:
function HelloComponent() { return ( <h1 className="greeting"> Hello, world! </h1> ); }
After the transformation process, the above JSX becomes regular JavaScript.
To quote Sebastian Markbåge, a software engineer at Facebook working on the React team, “By blending HTML tags and components and using upper-case names to distinguish our own components from HTML tags, JSX gives us the benefit of word sensibility with composability awesomeness.”
For developers, it’s ideal to become acclimated to JSX when pursuing React since it promotes elegant and readable code that can easily be understood in the context of how the final UI will appear. While complexities may arise due to the blend of JS and HTML-like code, the learning curve tends to be short and the long-term advantages are significant.
Understanding the Intersection of React and HTML
HTML and React intersect intricately within modern web development practices. This combination is essential to create dynamic, user-friendly, and interactive web interfaces. Utilizing HTML within React might seem slightly different than traditional methods due to the introduction of JSX, a syntax extension that allows developers to write HTML-like code directly within their JavaScript code.
Utilization of HTML in React
React essentially uses a version of HTML known as JSX (JavaScript XML) for its template structure. However, it is crucial to note that JSX is not strictly HTML; it subtly alters certain aspects to fit more naturally into a JavaScript environment. Hence, some common HTML attributes such as
class
must be switched to
className
when used within React, due to ‘class’ being a reserved word in JavaScript.
Example:
In traditional HTML, we would use:
<div class="example">This is an example.</div>
While in React (JSX), the same statement would look like:
<div className="example">This is an example.</div>
You can see that despite a few differences, at their core, both methods utilize similar structural syntax.
Embedding Expressions in JSX
React and JSX, together allow us to embed any valid JavaScript expression within curly braces in our JSX:
function example() { const name = 'John Doe'; return <h1>Hello, {name}</h1>; }
In above example, the JavaScript variable
name
is embedded inside the JSX output.
Expressing HTML Attributes with JSX
With JSX, you can also set HTML attribute values dynamically using a JavaScript expression inside curly braces.1
Example:
const title = 'React'; const element = <h1 title={title}>Hello, world!</h1>;
To encapsulate, it’s quite straightforward to embed HTML in React through the transformative capability of JSX. This structure provides a seamless intersection between HTML’s static nature and React’s dynamism. Its implementation forms the foundation of many modern-day, interactive web applications. It brings the happening ‘live web’ experience by enabling rapid rendering and updates to the user interface.
As American computer scientist Alan Kay remarks,
"The best way to predict the future is to invent it."
The dynamic amalgamation of HTML within React beautifully exemplifies this notion2.
Exploring JSX: The Blend of HTML and JavaScript in React
React, a popular JavaScript library used in modern web development, integrates HTML into its Ecosystem by means of JSX. JSX (JavaScript XML) is an extension to the JavaScript language syntax. It is not a necessary component when creating React applications; however, its utilization certainly polishes and simplifies the development process.
Consider how you could create a simple header in pure JavaScript:
const element = React.createElement( 'h1', {className: 'greeting'}, 'Hello, world!' );
You can observe that React uses `createElement()`, a method which becomes increasingly verbose with nestings. Now, visualize the same scenario using JSX:
const element = <h1 className="greeting">Hello, world!</h1>;
HTML-like structure can now be observed from the JavaScript code, making it more readable and manageable.
Ensure that your project installs the Babel compiler to transform JSX into standard JavaScript that browsers understand(source).
Despite being an extension of JavaScript, JSX is closer to HTML in its syntax pattern. However, there are several notable differences with HTML:
- Attribute names follow camelCasing
- Instead of ‘for’, ‘htmlFor’ must be used
- Instead of ‘class’, ‘className’ must be used
Embedding any valid JavaScript expression inside curly braces `{ }` within JSX is also achievable. This ability offers flexibility to the developers to fuse static HTML code with dynamic JavaScript variables/constants/functions.
let position = "Manager" const element = <h2> As a {position}, managing projects gave me a great sense of responsibility </h2>
Recognizing that JSX builds up on JavaScript rather than rewrite its rules, is a significant realization towards understanding how HTML is employed in React.
“To understand how HTML fits into React through JSX is to acknowledge the pivot point of merging design markup with logic.” – Chris Coyier, CSS-Tricks co-founder.
How to Embed HTML Elements within React Components
HTML Elements within React Components
React, an open-source, front-end library for building user interfaces or UI components, permits the use of HTML within its syntax. This combination is often called JSX (JavaScript XML). It’s a syntax extension to JavaScript and comes with the full power of JavaScript.1
Topic | Description |
JSX | A syntax extension that allows HTML-like code on your JavaScript, which is transpiled by Babel into standard JavaScript2. |
HTML in React | HTML can be used when defining what elements a component renders by way of tagging. |
Below is an example of this concept; a functional component rendering an HTML element:
{` function HeadingComponent() { return (Hello, World!
); } `}
The line inside the function shows an HTML heading tag being used directly within a React component. When this component gets rendered, “Hello, World!” will appear as a heading on the page.
Lastly, Facebook’s software engineer Tom Occhino once said about React:
“Just the fact that Facebook engineers build things that are this complex using the same tools, I think encourages other people to aim high.”
With features like HTML embeddings, this powerful library enables developers to build sophisticated applications with relative ease.3
Dealing with Potential Conflicts: Inline Styling in React vs. Traditional HTML
React, a popular JavaScript library for building user interfaces, often bridges the divide between HTML and JS through a syntax extension: JSX. While traditional HTML styling is typically done via inline CSS or external CSS files, React opts for a more integrated approach with styled components when reaching the same visual objectives.
Comparing Inline Styling in React and Traditional HTML
—————————————————
<div style={{color: "blue", fontSize: 16}}>Hello world!</div>
The previous piece of code exemplifies an inline style in React. The syntax differs from traditional HTML in that it uses JavaScript object notation inside a pair of curly braces rather than a string. This approach aligns with React’s overarching goal to blend HTML and JavaScript seamlessly within one ecosystem.
Contrastingly, conventional HTML styles elements using a string within the style attribute, as shown below:
<div style="color: blue; font-size: 16px">Hello world!</div>
Both methods achieve the same visual outcome, altering the color and font size of “Hello World!” However, they differ significantly in terms of scalability and maintainability when considering larger scale projects.
Advantages of Using Inline Styling in React
——————————————–
React’s preference for inline styling comes with several benefits:
– **Scoped Styles**: In React, styles are isolated per component, reducing risks of unintended side-effects on other components.
– **JavaScript Power**: Leveraging JavaScript for styling provides easy manipulation of styles dynamically based on conditions or states.
– **Ease of Use**: With all style information kept alongside the related JSX code, you have a single source truth, making debugging easier.
Can HTML be utilized within React?
———————————-
Yes, besides the stylistic differences, you can use HTML within React. The primary condition is incorporating under the umbrella of JSX. JSX bears an uncanny resemblance to HTML thus, for the most part, developers transitioning from HTML will experience a small learning curve – only mindfully adapting around differences like ‘className’ substitutions for ‘class’ in traditional HTML.
However, inline styles in React require adherence to the camelCase naming convention in lieu of the kebab-case in standard CSS (i.e., backgroundColor vs. background-color). Despite these nuances, once mastered, developers will find themselves well-equipped to handle any project – be it structuring layouts using familiar HTML tags or crafting dynamic, interactive UIs leveraging powerful JavaScript functionality.
Shedding light on this topic, Erik Aybar, a coding instructor and developer noted that “[HTML in React] is more of a mental shift than anything…Once you’ve trained your brain to make the switch, you can go between HTML and JSX without thinking about it.” Providing valuable reassurance to developers familiar with traditional HTML structures and looking to transition towards modern day front-end practices.
When dealing with potential conflicts with Inline Styling in React and Traditional HTML, it fundamentally boils down to the requirement of the project, existing expertise, and personal preferences. However, understanding the nuances between inline styling in React and standard HTML is essential in determining the best approach for your application.
Please consult the official React documentation for more on managing styles in React.From a holistic perspective, HTML and React are distinct entities but do share ample common ground. React, a powerful JavaScript library, is designed primarily for building user interfaces (UIs). It functions around reusable UI components, helping developers create highly interactive web applications. HTML, the markup language that structures all web content, is woven into React in a unique form known as JSX, providing the necessary framework to emulate HTML syntax within React components.
React | HTML |
---|---|
A JavaScript library | A Markup Language |
Used for creating UI components | Used for structuring web content |
Essentially, while HTML cannot be implemented directly in React, it does form its backbone in the shape of JSX. This realization forms a synergy between them, offering exciting possibilities. When delving deeper into the intricacies of combining HTML with React, we understand the necessity of this blend. It introduces a streamlined process, marrying the interactive nature of JavaScript with the structural foundation provided by HTML. Thus, JSX comes into play, integrating seamlessly with both practices and morphing traditional HTML into an acceptable format for use within React. Developers, thus, can utilize familiar HTML syntax while leveraging the capabilities of JavaScript.
<div className="App"> <header className="App-header"> Hello, React! </header> </div>
Geoff Graham mentioned, “HTML is cornerstone technology of WWW.” Incorporating a structured approach through JSX certainly fulfills Geoff’s statement, underscoring the need to interpolate HTML within React. The JSX syntax not only resembles HTML but also allows the application of logic within the same scope using JavaScript, which is a significant advance in web development techniques. Therefore, to put all these perspectives into context, it is evident that HTML, albeit in a slightly tweaked way through JSX, plays an integral part in React [development(https://realfavicongenerator.net/)].
One must understand that recognizing the correlation between HTML and React constitutes half the battle. The other half lies in harnessing the power of this relationship through intelligent and efficient coding strategies. Web developers must familiarize themselves with JSX and its nuances to gain an edge in React development. By doing so, they will equip themselves with the tools needed to construct robust, scalable, and dynamic web applications.