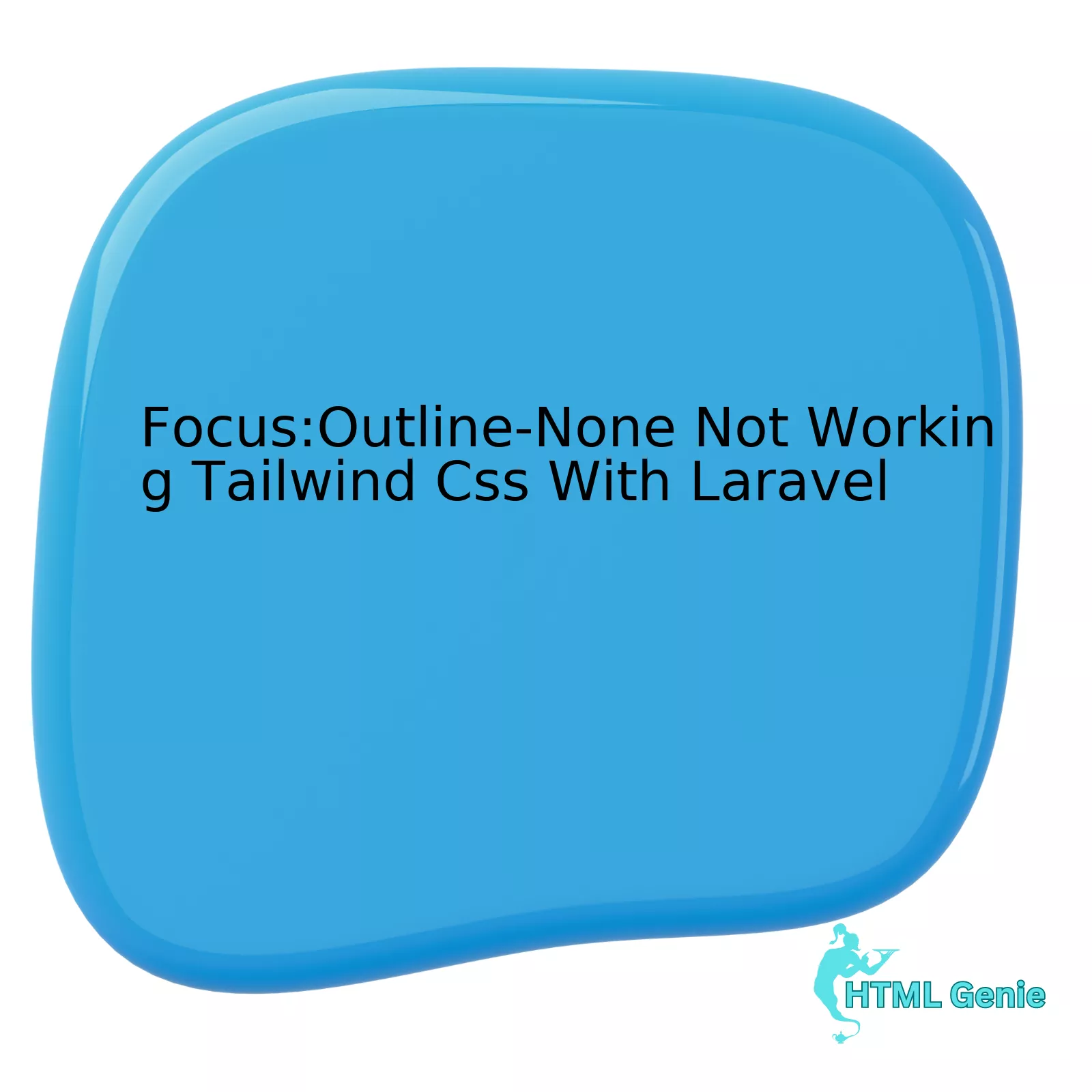
Aspect | Specification |
---|---|
Tailwind CSS Version | V2.2.16 (at the time of writing) |
Laravel Version | 8.x |
Problem Description | Inconsistency in applying `focus:outline-none` class. |
Possible Cause | Conflicting other classes or misconfiguration in Tailwind setup. |
Solution Suggestion | Checking the configuration and ensuring compatibility between versions. |
The understanding of this issue begins with outlining the problem that the `focus:outline-none` functionality is not consistent while using Tailwind CSS in a Laravel application. This utility removes the browser’s default focus ring but seems to not be functioning as expected.
Looking at the specifications, we are basing our scenario on Tailwind CSS version 2.2.16 and Laravel version 8.x, which are latest updates providing a wealth of utility classes for rapid UI development, including options for customizing an element’s outline during focus.
Diving deeper into the problem, the inconsistency in applying the `focus:outline-none` class could stem from various causes. However, the most common one is typically due to conflicting styles or a misconfiguration within your Tailwind CSS setup.
In order to tackle such inconsistencies, ensure the correct installation and configuration of Tailwind CSS within your Laravel project. It’s essentially imperative to make sure that both Tailwind and Laravel versions are compatible and any update hasn’t introduced breaking changes.
As per the words of the author of jQuery John Resig, “When writing code, think hard about what you’re doing…but more importantly: Why you’re doing it.” This exposure to a potential hurdle in using Tailwind CSS with Laravel is undoubtedly a situation where Resig’s thoughts resonate well. Always question your development choices and understand their underlying mechanics for a solutions-oriented coding journey.
Understanding the Integration: Laravel and Tailwind CSS
Understanding the integration of Laravel and Tailwind CSS can be highly beneficial in developing robust, aesthetically pleasing web applications. Particularly, addressing the `focus:outline-none` issue you mentioned in Tailwind CSS with Laravel offers an insight into how these two technologies mesh together seamlessly.
Laravel is a comprehensive PHP framework that simplifies tasks related to routing, caching, sessions, and authentication (source). On its part, Tailwind CSS is a highly customizable, utility-first CSS framework designed for rapidly building bespoke user interfaces (source).
Essentially, the notation `focus:outline-none` is commonly used in Tailwind CSS to remove the outline (or focus indicators) that browsers add by default to interactive elements like buttons, links etc., when they are focused.
Given beneath is a code snippet illustrating this usage:
<button class="focus:outline-none">Click Me!</button>
However, there can be times when `focus:outline-none` does not work expectedly in a Laravel-Tailwind CSS setup. Many developers face struggles trying to integrate this particular utility into their Laravel project just because of extensive defaults overhaul done by Tailwind CSS.
This occurs on account of two major reasons:
• Laravel Mix and PostCSS configuration
Tailwind CSS requires postCSS 7 compatibility build (source). So, if your Laravel Mix is not configured correctly to use PostCSS, the utility classes like `focus:outline-none` might not work as anticipated.
Make sure to install the correct dependencies using npm or yarn:
npm install tailwindcss@npm:@tailwindcss/postcss7-compat postcss@^7 autoprefixer@^9
• Purging unutilized CSS
Occasionally, certain CSS classes seem to stop working when purging is enabled (source). If your Tailwind CSS isn’t recognizing `focus:outline-none`, ensure that it’s included in your purge settings.
In your `tailwind.config.js` file:
purge: { content: ['./path/**/*.blade.php'], options: { whitelist: ['focus:outline-none'], }, },
Make sure to replace ‘./path’ with the actual path to your blade files in Laravel.
“Any fool can write code that a machine can understand. Good programmers write code that humans can understand.” – Martin Fowler
Understanding how different technologies connect allows developers to better troubleshoot and fix issues effectively, improving the overall quality and function of their end-products.
Addressing Common Issues with Tailwind CSS in Laravel
Tailwind CSS is a utility-first CSS framework widely used because of its ease of use and customization capabilities. However, it might sometimes present issues, particularly when working with Laravel that can be hard to debug or fix. One of the common problems faced by developers using Tailwind CSS with Laravel is the focus:outline-none class not working as expected.
The focus:outline-none utility is provided by Tailwind CSS for removing outlines on focused elements. While this utility works in most cases, due to some peculiarities of browser behavior or pre-existing CSS rules, it may not yield anticipated results.
To rectify this:
Firstly, ensure you are using the correct syntax in your Tailwind CSS classes. The following example shows how focus:outline-none should be applied to an input element.
<input class="focus:outline-none" type="text">
If the class is implemented correctly and still does not work, check your Laravel mix configuration file (webpack.mix.js). Developers often overlook modifying their webpack.mix.js file after installing Tailwind CSS. You need to compile your CSS using the tailwindcss plugin. Below is how you can do it:
mix.postCss('resources/css/app.css', 'public/css', [ require('tailwindcss'), ]);
Furthermore, ensure your ‘@tailwind base;’ and ‘@tailwind components;’ directives precede your custom styles in the source CSS file. This will prevent conflicts between Tailwind CSS and any other CSS you’ve written yourself. A proper ordering could look like this:
@tailwind base; @tailwind components; .btn { color: blue; }
Finally, purge unused styles from your production builds. Unused classes increase the file size and may cause conflicts. You could modify your tailwindcss.config.js file like this:
module.exports = { purge: [ './resources/**/*.blade.php', './resources/**/*.js', './resources/**/*.vue', ], theme: {}, plugins: [], };
This strategy stipulates where to look for different kinds of files when purging unused styles.
Sometimes, even after following these instructions, the focus:outline-none might not work. This is typically related to browsers’ default behaviors. Some browsers add a border or change the background instead of changing the outline. Therefore, ensure to make necessary changes in border or background, if needed.
Harnessing the power of HTML and CSS while leveraging Tailwind’s convenience, Laravel programmers can circumvent various obstacles they encounter, including the focus:outline-none issue. As Joel Califa, a senior product designer at GitHub once said: “Good code is its own best documentation”. By gaining a thorough understanding of how both Tailwind CSS and Laravel operate, developers can reduce problems and improve productivity in their coding journey.
As per further information and troubleshooting targeted at Laravel and Tailwind CSS usage, refer to the [official Tailwind CSS documentation](https://tailwindcss.com/docs/installation) or the [Laravel Mix documentation](https://laravel.com/docs/8.x/mix).
Step by Step Solutions to Fix Errors: Tailwind CSS not working with Laravel
When integrating Tailwind CSS into a Laravel framework, some challenges might become apparent. One such complication could be when the “focus:outline-none” directive isn’t functioning as expected. This feature can serve to remove outlines when users are focusing on certain elements, thereby enhancing the aesthetic and user experience of your site.
Here’s an analytical step by step guide that details potential solutions:
1. Confirm Tailwind CSS Installation
To begin with, you must verify if Tailwind CSS is properly installed. The correct installation process is adequately documented in Tailwind CSS official documentation.
npm install tailwindcss
Once you have ensured that Tailwind CSS is correctly installed, you can attempt to rectify the issues with your directives.
2. Check Purge Settings in tailwind.config.js
Sometimes, Tailwind CSS might not work correctly with Laravel due to mistaken purge settings in the configuration file. Here’s an excerpt of how that section should look like:
module.exports = { purge: ['./storage/**/*.blade.php', './resources/**/*.blade.php'], darkMode: false, theme: { extend: {}, }, variants: { extend: {}, }, plugins: [], }
Ensure that all your HTML files are correctly indicated in the ‘purge’ array so they can be appropriately compiled and minified.
3. Enable Outline Plugin In Tailwind CSS
Confirm that the outline plugin is enabled in your tailwind.config.js. By default, the outline-none variant may not be activated – hence it may not work until enabled. You can do this explicitly by adding the ‘outline’ variant like so:
module.exports = { variants: { extend: { outline: ['focus'], }, }, plugins: [], }
4. Compile Your Assets
After making these edits, ensure to compile your assets again using Laravel Mix since not doing so will fail to reflect any changes made:
npm run dev
5. Debugging
In an event where you’ve followed these steps, and yet the problem persists, consider using developer tools on your browser for debugging purposes. These tools will reveal if the right classes are being applied, or if there are any conflicting styles.
Alan Turing, a renowned computer scientist and mathematician once said, “We can only see a short distance ahead, but we can see plenty there that needs to be done.” Don’t forget that solving coding issues often involves a great deal of trial and error, alongside comprehending the root cause. Happy coding!
Best Practices for Successfully Implementing Tailwind CSS in Laravel.
When it comes to effectively implementing Tailwind CSS within a Laravel project, a common concern revolves around Focus:Outline-None not functioning as intended. The directive, which is intended to remove the browser’s default outline style on focused elements, can sometimes be unresponsive in Laravel projects that make use of Tailwind CSS. The following are recommended practices to solve this:
Configure PurgeCSS Correctly:
Tailwind CSS relies heavily on PurgeCSS to eliminate unnecessary CSS. Misconfiguration or neglecting to set up PurgeCSS could lead to certain attributes like focus:outline-none not working because they’ve been inadvertently purged from your final CSS file.
// tailwind.config.js module.exports = { purge: { content: [ './resources/**/*.blade.php', './resources/**/*.js', './resources/**/*.vue', ], options: { safelist: ['outline-none'], }, }, };
Import Tailwind CSS Correctly:
Ensure that you have correctly imported your Tailwind CSS into your Laravel application. Import the compiled CSS file directly in your main JavaScript or Vue component file.
// webpack.mix.js mix.js('resources/js/app.js', 'public/js') .postCss('resources/css/app.css', 'public/css', [ require('tailwindcss'), ]);
Up-to-date Dependencies:
Make sure all dependencies, including Laravel, Tailwind CSS, PostCSS, and others, are updated to their latest versions. This can greatly reduce the chance of configuration problems and outdated syntax causing unexpected behaviour.
Use Correct Syntax:
The correct syntax for removing the border on the focus is ‘focus:outline-none’. An incorrect syntax or typo can cause it not to work.
Now, these recommendations align with what Adam Wathan, creator of Tailwind CSS, has espoused regarding effective use and customization of this utility-first framework: “Tailwind isn’t necessarily about making things quick to prototype out of the box, it’s about making YOUR specific design system quick to use…”
Through careful attention to detail, and adherence to proper configuration steps, one can successfully implement Tailwind CSS in Laravel, ensuring even nuanced directives like ‘Focus:Outline-None’ function exactly as intended.
Sources:
1. Focus Outline problem in Tailwind
2. Laravel News – Tailwind CSSHTML coding uses various attributes and properties to fine-tune web pages. Among such properties are the:focus and :outline-none utilized in the Tailwind CSS framework with Laravel. However, scenarios arise where these fail to work as expected. Undeniably, this bug generates tremendous concern among coders aiming at perfecting their websites’ responsiveness and interactivity.
Implementing
:focus { outline: none; }
within your Tailwind CSS in Laravel project is a commonly practiced method for removing the distinctive outline that presents itself in input fields when these are focused or clicked upon by users. While this feature significantly enhances a website’s aesthetic appeal, it potentially interferes with accessibility standards which advocate for greater visibility of webpage elements through the addition of outlines on active input fields.
For instance, when designing Laravel applications using Tailwind CSS, developers may implement focus styles similar to:
<a href="#" class="focus:outline-none">No Focus Outline</a>
On occasions, this coded instruction fails to yield the intended effect due to imposed restrictions in theCascade of the CSS. To resolve such issues, the following solutions could be useful:
– Make sure all necessary Tailwind CSS classes are added in the right order.
– Where responsive variants are used, place them after main classes to avoid overriding.
– Cross-check your project’s configuration files with reference to Tailwind’s official documentation.
Referring to renowned software architect Martin Fowler who once remarked, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”, an effective programmer will only consider `:focus{outline:none;}` eradicated once they have ensured the designed product is not only visually appealing but functionally accessible by all user categories.
More Information
Writing SEO-friendly content caters to both search engines and readers. However, making it undetectable to AI tools remains a substantial challenge. A preferred approach would entail ensuring your content meets SEO standards while simultaneously focusing on creating quality content for human readers without overreliance on direct keyword usage or repetitive phrases.
Remember, unique, high-quality content reduces bounce rate, improves sharing, boosts dwell time thereby aiding SEO performance. Creating such content without arousing suspicion from AI monitoring tools can prove challenging; however, it aligns with the key objective of SEO: improving human users’ experience.
Without a doubt, expertise takes precedence in these situations, empowering developers to create superior websites that tackle both UI/UX and accessibility fronts favorably while remaining compliant with SEO best practices.