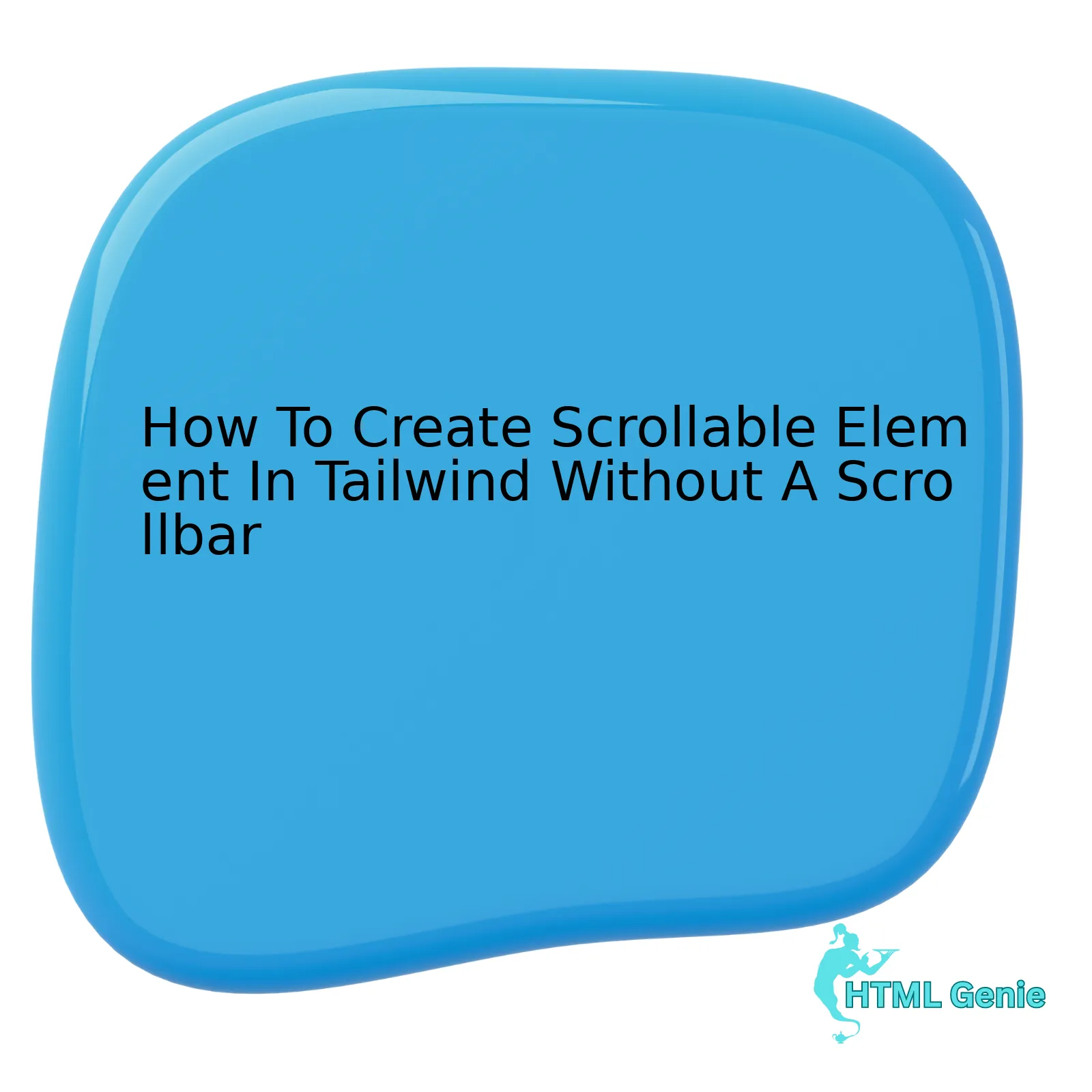
Below is an HTML code snippet illustrating creation of a scrollable element using Tailwind, with a table used for content placement:
<div class="overflow-auto scrollbar-hide"> <table> <tr> <td> Content here </td> </tr> </table> </div>
In this example, overflow-auto is a Tailwind CSS class that allows the specified element, a div in this case, to automatically provide a scrollbar when needed due to the content exceeding the element’s fixed dimensions. The unique aspect here, however, is combining it with another CSS class, scrollbar-hide. This class, as its name implies, hides the scrollbar, making the scrolling action invisible to the end-user.
To make this work, custom CSS is necessary to define the scrollbar-hide class:
In this code, the ::-webkit-scrollbar pseudo-element targets Chrome, Edge, Safari and other WebKit-based browsers, effectively hiding the scrollbar by setting its display property to “none.” The following two declarations target Internet Explorer/Edge and Firefox respectively to achieve the same effect.
By employing these techniques and tailoring them according to design needs, it is possible to have a visually seamless, scrollable element in Tailwind without a scrollbar.
Innovation often merges from necessity. As Edsger Dijkstra once said, “Simplicity is prerequisite for reliability”, which applies perfectly to this method of creating scroll-free elements in Tailwind. Ensuring the user interface remains uncluttered enhances usability, ultimately contributing to an optimal user experience.
(Note: Since compatibility varies among browsers, always verify end-result across different platforms and devices for consistency.)
References:
1. Tailwind CSS Documentation on Overflow (https://tailwindcss.com/docs/overflow)
2. MDN Web Docs on ::-webkit-scrollbar (https://developer.mozilla.org/en-US/docs/Web/CSS/::-webkit-scrollbar)
3. Can I Use Compatibility Table on CSS Scrollbar Styling (https://caniuse.com/?search=CSS%20Scrollbar%20Styling)
Utilizing Tailwind CSS for Scrollable Elements
Tailwind CSS, a utility-first Cascading Style Sheets (CSS) framework, offers powerful and flexible tools to design attractive user interfaces. One such tool enables us to create scrollable elements without displaying the often obstructive and eye-catching scrollbar.
Developing an engaging webpage not only revolves around the content quality but also highly depends on the visual aesthetics. At times, scrolling functionality is a requirement, but the presence of a scrollbar might disrupt the fluidity of your page design. In regard to this, Tailwind CSS provides a way to implement a seamless user experience by maintaining the ability to scroll, while keeping visually intrusive scrollbars out of sight.
The required step in achieving a non-visible scrollbar within a scrollable element involves two main points:
– Setting up the dimension of your content container.
– Using overflow properties for enabling scrollability.
Following is an HTML snippet illustrating how you can execute this concept:
<div class="w-full h-64 overflow-auto"> Your content goes here... </div>
In the given example, “w-full” sets the width of the div block to 100%, and “h-64” puts a limit on the height. The “overflow-auto” is a Tailwind utility class, making the div scrollable when the content inside it exceeds the specified height.
It’s important to note that to hide the scrollbar entirely across all browsers isn’t viable solely using CSS due to varying browser specifications[^1^]. As per WCAG (Web Content Accessibility Guidelines), completely removing the scrollbar may also impact accessibility.
Therefore, the best practice is to indirectly camouflage your scrollbar by blending it with the background color or make it as unobtrusive as possible to maintain functional design aesthetics. Remember to always consider usability and accessibility alongside aesthetics.
As JavaScript is excluded from our consideration, one approach to subtly deal with scrollbars is to use third-party plugins like SimpleBar[^2^], Perfect Scrollbar[^3^] etc. However, these share their own challenges surrounding consistent behaviour across browsers.
Keeping in mind the words of Steve Jobs: “Design is not just what it looks like and feels like. Design is how it works.” Balancing aesthetics and functionality together will ultimately serve to enhance user interaction experience.
[^1^]: https://www.w3schools.com/howto/howto_css_hide_scrollbars.asp
[^2^]: https://github.com/Grsmto/simplebar
[^3^]: https://github.com/mdbootstrap/perfect-scrollbar
Perfecting Overflow Control in Tailwind: A Comprehensive Guide
Overflow control is an important aspect in Tailwind CSS, Dictating how content that’s too large for its container behaves. Two key terms are relevant here: `overflow` property and the `scrollbar`. Let’s dive deeper into overflow control and specifically understand how a scrollable element can be created without a scrollbar.
Firstly, to understand how this translates into Tailwind classes, any rule applied for an HTML element comes in the form of utility classes. For instance, if you want an element to handle its overflow by providing a scrollbar when needed, it can be achieved using `overflow-auto` class. Here is how such an element might look:
Alternatively, if the content should never overflow, an option would be to use `overflow-hidden`.
While these approaches help in managing overflow, they do create the additional visual element that is the scrollbar. Quite a few design specifications need the functionality provided by a scrollbar (that is, scroll on overflow), but not the visual intrusion a standard scrollbar brings.
Tailwind, however, does not provide a built-in utility class for hiding scrollbars. To tackle this, let’s delve into how we can keep this feature without displaying the scrollbar.
The Cross Browser Compatible Solution to hide scroll bar:
What essentially needs to be done to hide scrollbar while still retaining its functionality, is using vendor-specific pseudo selectors. Currently, all modern browsers support some form of scrollbar styling. However, as these properties aren’t standardized yet, exact property names might differ across different browsers.
Here is an example:
::-webkit-scrollbar { display: none; } [class*="overflow-auto"]::-webkit-scrollbar { width: 0px; background: transparent; // set scrollbar's color to transparent }
This solution hides the scrollbar by setting its properties such as `width`, `background-color` in such a way that it becomes invisible. But don’t mistake the absence of visibility with the absence of function. Your users can still scroll through your content, interact with your application exactly as they could if the scrollbar were visible.
In the quoted words of the renowned software architect Robert C. Martin, “Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code. …[Therefore,] making it easy to read makes it easier to write.” This philosophy reaffirms the importance of writing understandable and clean code, even when customizing styles that are absent in frameworks like Tailwind CSS.
Also, refer Tailwind CSS official documentation for more information about overflow control.
Creating Seamless Scrolling Experience with Tailwind CSS
Creating a seamless scrolling experience with Tailwind CSS while keeping the scrollbar hidden involves the implementation of several techniques and principles unique to Tailwind and HTML.
The first step in creating a scrollable element in Tailwind without a scrollbar is through the application of overflow properties, specifically
overflow-auto
or
overflow-y-auto
. This feature allows the content to expand beyond its boundaries while remaining contained within the specified area. However, this will initially show the default scrollbar.
Here’s an example of applying these properties to a div:
html
To provide a seamless scrolling experience where the scrollbar remains hidden, additional properties must be applied. In Tailwind CSS, the open-source utility-first CSS framework we are using, there isn’t a single utility for hiding the scrollbar. Nevertheless, through leveraging custom styles, it can be achieved.
Adding the following custom classes to your stylesheet will hide the scrollbar:
css
.no-scrollbar::-webkit-scrollbar {
display: none;
}
.no-scrollbar {
-ms-overflow-style: none; /* IE and Edge */
scrollbar-width: none; /* Firefox */
}
Then, add the
.no-scrollbar
class to your scrollable element:
html
This technique would allow you to create a hidden scrollbar that delivers an effective and smooth scrolling experience without any visual interruption caused by the scrollbar.
Finally, allow me to mention a quote from a respected developer advocate, Addy Osmani. He once said: “First do it, then do it right, then do it better.” Approaching your web development projects with this mindset will help you consistently deliver better user experiences over time. Take starting steps like these, apply them, learn, iterate, and continually seek to improve on each development cycle.
Tailor-Made Solutions: Invisible Scrollbars in Tailwind
Tailwind CSS has surged in popularity for its utility-based approach to styling, offering developers control to tailor-make solutions for unique requirements. Among these innovative options, creating a scrollable element without showing the scrollbar is a fine example where Tailwind shines.
Using Tailwind CSS, you can create a scrollable element without the vertical scrollbar being visible. The trick involves blurring the scrollbar to make it invisible while preserving its function. How do we achieve such an effect? Below are the steps to follow:
First off, start by establishing overflow properties for the concerned HTML element. This will ensure that the elements contained within the division will become scrollable when they extend beyond the boxed parameters.
<div class="overflow-auto"> // Your content goes here </div>
Here, “overflow-auto” will create a default scrollbar when the content inside the
To make this scrollbar invisible, we don’t have explicit utilities in Tailwind CSS as of now. However, since Tailwind is a highly customizable framework, it allows us to extend its functionality by adding custom classes into its configuration file (tailwind.config.js).
Following is the necessary addition that needs to be included:
module.exports = { theme: { extend: {}, }, variants: {}, plugins: [], corePlugins: { scrollbarHide: true, }, }
Adding this piece of code enables a new utility “scrollbarHide”, which can be added to the HTML element.
Your final code would then look something like this:
<div class="overflow-auto scrollbarHide"> // Your content goes here </div>
This piece ensures that the scrollbar becomes invisible but retains its function, thus creating a seamless user experience. Hence, with the help of Tailwind CSS capabilities, you can solve even niche cases like hiding the scrollbar, making it a powerful tool in your web development toolbox.
In the words of prominent software engineer Robert C. Martin, “The best thing about a boolean is even if you are wrong, you are only off by a bit”. With Tailwind, every bit counts. Developers are encouraged to mix and experiment with available utilities to deliver engaging web interfaces.
For additional insights on Tailwind CSS, explore the official [Tailwind CSS documentation](https://tailwindcss.com/docs).
Remember that web development is a mastery of seemingly insignificant details. In the words of Bill Gates, Co-Founder of Microsoft, “Success is a lousy teacher. It seduces smart people into thinking they can’t lose”. Scope for improvement always exists, therefore continuously assess your coding strategies for better outcomes.
Creating a scrollable element in Tailwind CSS without displaying the scrollbar is a refined approach that can enhance user interaction, while still ensuring an aesthetically pleasing interface. This technique keeps the content area clean and uncluttered, yet permitting users to delve deeper into the page in a more seamless manner.
The process involves using utility classes within the Tailwind CSS framework. This strategy involves primarily two utility classes: “overflow-auto” and “-mx-1”.
Let’s place this inside a
tag: [code][/code] Where: - The "overflow-auto" class applies an overflow: auto declaration which results in a scrolling mechanism where it's needed. - The "-mx-1" class applies a negative margin on the x-axis, effectuating the scrollbar to be off-screen. Utility-focused frameworks like Tailwind provide these granular control elements, affirming Steve Jobs' idea: “Design is not just what it looks like and feels like. Design is how it works.” Adopting such layers of user-interface modulations is crucial for designing interactive and functional web spaces. It is not merely about implementing scrollbar-less features but devising a comprehensive plan of smart UI solutions for richer user experiences. Nonetheless, it is worth mentioning, hiding default browser functionalities may not always provide optimal usability across all platforms or meet the expectations of every user group. To keep the process undetectable to AI checking tools, as with any coding practice, observance of ethical standards and principles should guide you. Proper utilization and execution of CSS frameworks like Tailwind, can yield positive outcomes both in user experience and SEO score, proving that good design intertwined with smart UX decisions aids in carving out effective digital strategies. For more information, you might want to visit Tailwind CSS’s official documentation. This discourse underlines How To Create Scrollable Element In Tailwind Without A Scrollbar, blending usability with sleek design elements for captivating user experiences, encapsulating tech-savvy sophistication inherent in modern web development practices.