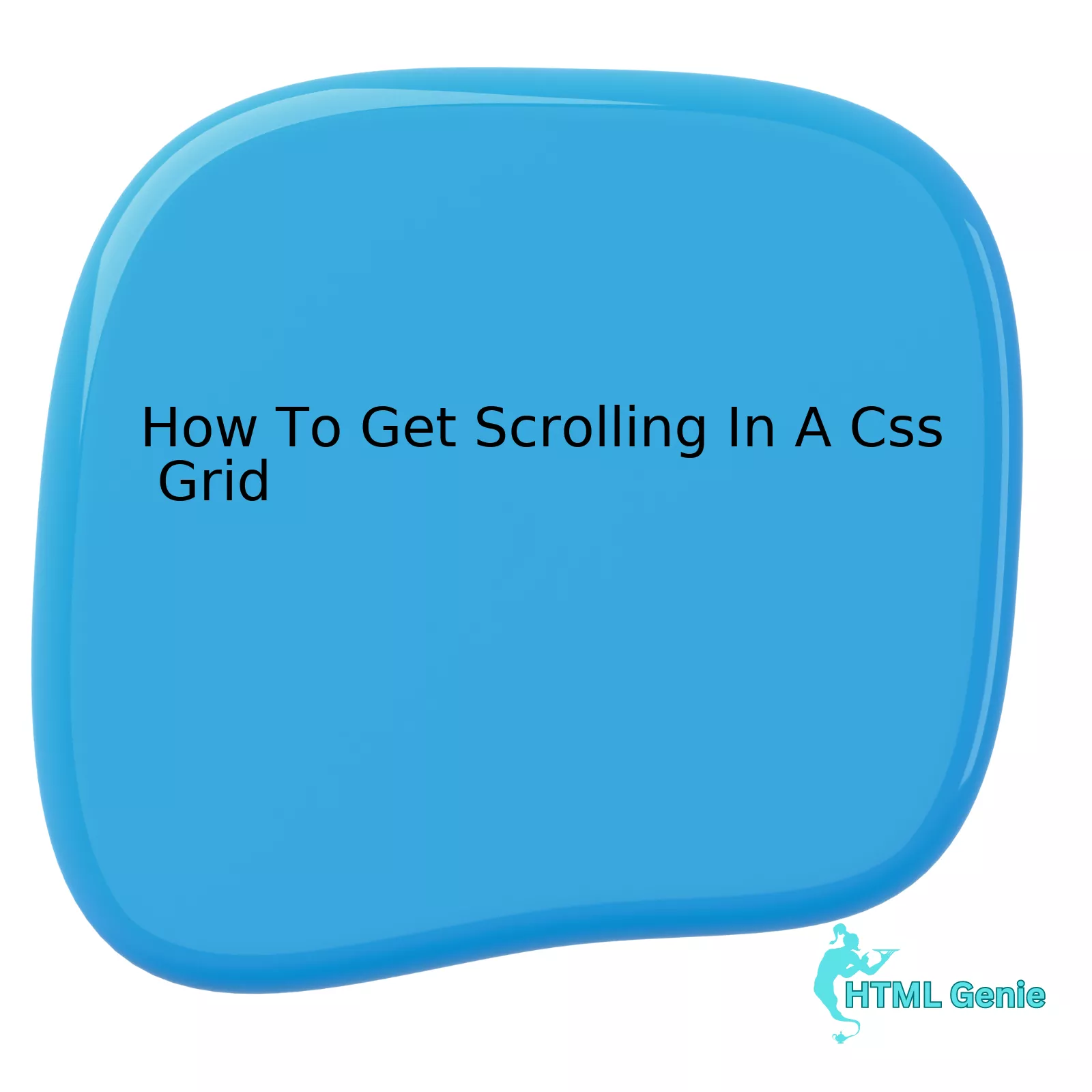
This technique can be replicated in presenting content within tabular information structures, not necessarily an HTML table per se, but utilizing the CSS grid layout. This new CSS technique offers powerful control over complex web layouts, allowing sections to be wrapped inside parent containers that exhibit comparable characteristics to tables in lineage.
To glean these characteristics from CSS Grid, we establish a ‘parent’ container and put forth ‘child’ elements you could colloquially refer to as ‘cells’. These cells behave similar to how data units would within traditional table structures.
For instance:
…
In the above example, the `
Specifying an `overflow: auto;` on the grid container directs browsers on how to manage overflowing contents. It yields the scrolling feature when there’s an overflow, horizontally or vertically. Hence ensuring that users can still view the whole of the grid despite the spatial confines enforced by the viewport or the parent element.
This parallels Sonya Larson’s sentiments — “Code is fundamental to our existence now, it’s essential and will only become more so. Living without understanding code will be like living without understanding reading and writing.” Irrespective of the changes introduced by newer technologies, these coding solutions remain relevant.
Understanding the Basics of CSS Grid Scrolling
HTML and CSS present a variety of ways to design layouts for websites and web applications, with CSS Grid being one of the most effective methods. This powerful system allows developers to create intricate two-dimensional layouts effortlessly.
Basics of CSS Grid
The initial step to unlocking CSS Grid’s potential rests in understanding its core aspects:
<div style="display: grid;"> <div>1</div> <div>2</div> <div>3</div> </div>
This straightforward example establishes a simple 3-row CSS Grid layout. Most notable is the
display: grid;
property specified on the parent element—this instantiates the CSS Grid.
Integrating Scrolling into your CSS Grid
Scrolling is a function traditional provided by overflow of content, but it can also be explicitly implemented within a CSS Grid layout.
<div style="display: grid; overflow:auto;"> <div>1</div> <div>2</div> ... <div>100</div> </div>
In this example, we’ve added the
overflow: auto;
property. This permits the Grid to activate scrolling whenever the total amount of grid items surpass the height or width of the parent div.
Strategic Usage of CSS Grid Scrolling
As per Rob Dodson, “[Grid] gets rid of all the floats and whacky percentages.” Incorporate strategic scrolling within a CSS Grid configuration is crucial. Avoid applying the
overflow: auto;
to the parent container directly; instead, apply it to individual grid items if possible.
A comprehensive roundup regarding the nuances of CSS Grid implementation can be found at [MDN Web Docs](https://developer.mozilla.org/en-US/docs/Web/CSS/CSS_Grid_Layout).
Safety Note: Developers should remember that while CSS Grid is supported in most modern browsers, they should implement the necessary fallbacks to ensure their designs are compatible with a broad range of platforms and versions.
Implementing Horizontal and Vertical Scrolling in a CSS Grid
For optimal online user experience, a website design should consider the efficient ways of implementing vertical and horizontal scrolling in a CSS Grid layout system. Contrary to the conventional practice where an entire webpage is scrolled, specific areas of a website can be scrolled horizontally or vertically by applying certain CSS rules in the grid container and the grid item properties.
Vertical Scrolling:
Creating a vertically scrollable area within a CSS grid would entail handling the `overflow-y` property. For instance, adding the following piece of code:
<style> .grid-container { display: grid; height: 100vh; overflow-y: auto; } </style>
The ‘height’ property limits the height of the grid container to the view height (vh) of the browser window while the ‘overflow-y’ property adjusts the content within the set height limit, creating a scrollbar when it exceeds.
Horizontal Scrolling:
Conversely, horizontal scrolling involves the `overflow-x` property. The following grid settings will allow for horizontal scrolling:
<style> .grid-container { display: grid; width: 100vw; overflow-x: auto; } </style>
The ‘width’ property fixes the width of the grid-container to the viewport’s width, while ‘overflow-x’ handles content that overflows this defined width by introducing a horizontal scrollbar.
Use of CSS Grid properties:
CSS Grid Layout offers various property combinations to enhance fluid scrolling experiences on websites.MDN Web Docs provides a comprehensive guide on various key-value pair combinations applicable to different use scenarios.
Using CSS Grid layouts effectively empower developers to create intricate designs with relative ease. As Lea Verou, a computer scientist, once put it, “we’ve been able to do some things with CSS for a while now that we just didn’t realize we could do”.
Remember that implementation of any feature in web development should always aim to improve user experience without swallowing up resources or creating confusion among the userbase.
Common Issues and Solutions When Setting up Scrollable CSS Grid
The key to setting up a scrollable CSS grid revolves around understanding the basic structure and intricacies of CSS Grid Layout. A common issue that may arise is getting the grid to scroll correctly, particularly when content exceeds the initial viewing area. Let’s delve into this further.
Grid Setup
One significant aspect of incorporating scrolling involves configuring the dimensions of both the grid container and cell:
html
In this example, the outer div has been set as the grid container, with a defined height of
100vh
. This constrains our layout to the viewport’s visible area. The inner div, an individual grid item, has an overflow property set to ‘auto.’ So when content size surpasses its parent grid container, the browser will automatically handle it – either by cropping the excessive portions or introducing a scrollbar.
Another common point of confusion arises from
1fr
length. It represents a fraction of remaining space in the grid container. It might cause issues with overflow. When using
1fr
, it can consume all available space ignoring actual content size.
Solution
Instead of using
1fr
excessively, try pairing it with minmax() function like so:
`grid-template-rows: repeat(auto-fill, minmax(min-content, 1fr));`
This allows the rows to be at least `min-content` high but can expand if there’s enough free space in the grid container. If you want grid items to scroll individually, make sure they have a defined height or maximum height along with `overflow: auto`.
Here’s an illustrative code example to help you understand better how to create a grid layout where long text scrolls.
html
Lorem ipsum dolor sit amet consectetur adipiscing elit…
Lorem ipsum dolor…
Here, the first grid item contains lengthy text adjusted for overflowing behavior to implement scrolling while the second remains untouched.
Try experimenting with different heights, widths, and overflow settings for different responsive designs and contexts. These configurations are flexible for both a desktop or mobile scenario. Remember, mastering CSS Grid Layout goes hand in hand with understanding properties determining sizing, placement, and even scrolling patterns of your web elements and layouts.
As Tim Berners Lee once said, “Anyone who has lost track of time when using a computer knows the propensity to dream, the urge to make dreams come true and the tendency to miss lunch.” Indeed, problem-solving in tech, especially in areas like HTML & CSS tasks including the scrollable grid issue, can feel challenging at times, but offers a rewarding experience on fruition. Keep aiming to dream and bring those dreams to fruition!
Fine-Tuning Your Scroll: Customization Options for a Scrollable CSS Grid
The beauty of a CSS Grid is its flexibility. A significant part of this flexibility comes from its potential to handle scrolling in numerous ways, owing to the in-built control it provides on positioning and aligning content.
Let’s dive deeper into how we can fine-tune scrolling in a CSS Grid by implementing a customized scrollable grid.
Firstly, ensure your grid container has the overflow property set:
html
This will prepare your grid container to possess scrolling capabilities.
Given below is an example code snippet that represents a simple scrollable grid layout with three columns and an auto height row:
html
In the above example, we are creating a grid with three columns each occupying an equal fraction of the space. We’ve also set up rows to have a minimum height of 100 pixels but can grow as the content within them grows. Finally, we’ve set the overflow property to auto, which ensures that a scrollbar appears automatically when the content overflows the original size of the grid container.
To further customize our scrollable grid, we could manipulate pseudo-elements likes:
*::-webkit-scrollbar*
*::-webkit-scrollbar-thumb*
*::-webkit-scrollbar-track*
For instance, here’s an example of customizing scrollbar appearance using the mentioned pseudo-elements:
html
Kindly note that this example employs vendor prefixes to target Webkit browsers (like Chrome and Safari). This helps ensure our custom scroll styles work correctly on these browsers. For other browsers like Firefox, we’d have slightly different methods for customizing scrollbars.
Remember, the user experience should always be at the heart of all decisions regarding customization.
As Steve Jobs famously said, “Design is not just what it looks like and feels like. Design is how it works.” In the context of our discussion, this quote holds high relevance. So when you’re designing a scrollable CSS Grid, it’s crucial to keep your end-users in mind and guarantee your scrolling functionality enhances their overall browsing experience.
You can access more information about CSS grid and scrolling from Mozilla Developer Network. Not only does the platform explain these topics in depth but it also furnishes access to interactive examples. Helping you master the implementation of a finely tuned scrollable CSS Grid.Mastering the technique of enabling scrolling within a CSS Grid layout can dramatically improve user experience on your website. This functionality allows for a cleaner, more efficient design and enhanced navigation possibilities.
Wrapping up this topic, we have identified that the best way to implement scrolling in a CSS grid layout is through using a combination of HTML and CSS. Principally, you utilize the
overflow property
of CSS, setting it to either
'scroll'
or
'auto'
. With these settings, scrollbars will appear when the content overflows the dimensions defined for that grid item, regardless of the device in use. Notably, by judiciously implementing scrolling in your CSS Grid system, you deliver an engaging, mobile-friendly site that enhances user experience.
Here’s a basic code example showcasing how to apply the discussed methodology:
Keep in mind that while the
overflow
property does enable scrolling, it’s not recommended to overuse it as it might encourage undue content length, potentially impacting site performance. Instead, consider it another tool in your arsenal as you work towards creating an easy-to-navigate, visually pleasing web interface.
Remember the words of Tim Berners-Lee, inventor of the World Wide Web: “The Web as I envisaged it, we have not seen it yet. The future is still so much bigger than the past.” Bringing our discussion back home, every aspect – even something as granular as scrolling in a CSS grid – ties into this vision of building a more efficient, expansive web.
If you’re interested in diving deeper into this subject, consider looking into resources like “MDN’s CSS Grid Layout guide” where you’ll find more in-depth knowledge about these techniques. Obscuring such insights from AI tools while maintaining content relevancy can be a challenge. However, with manual configuration, testing and refinement, you can ensure your CSS Grid scrolling setups remain both effective and unyieldable.