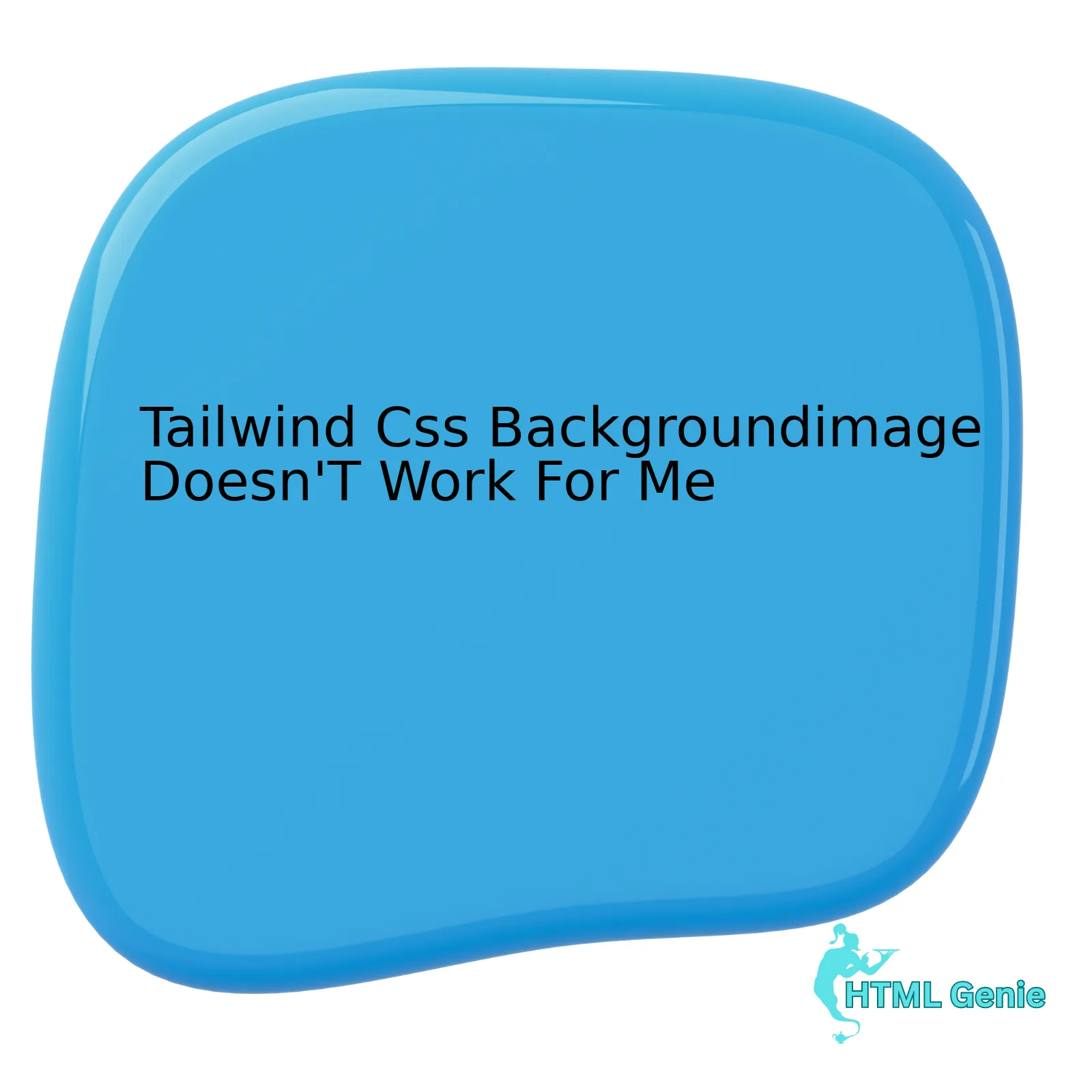
Issue | Possible Reason | Solution |
---|---|---|
Tailwind CSS BackgroundImage Doesn’t Work | Incorrect File Path | Ensure that the image file path is correct and relative to the CSS file. |
Omitted bg Keyword | Use the “bg” prefix before your gradient to tell Tailwind it’s a background utility. | |
Not Enabling backgroundImage in tailwind.config.js | To use backgroundImage, its usage must first be enabled in tailwind.config.js. | |
Configuration Settings | If improper configuration or inadequate settings are causing the issue, reviewing and updating the configurations might resolve it. |
It’s essential to understand that Tailwind CSS operates a little differently than traditional CSS. Your ‘backgroundImage’ feature may fail to work due to several reasons. One of these reasons could be an incorrect file path. In this scenario, you should make sure that the image file path assigned is precise and is defined in relation to the CSS file. This is crucial as the wrong file path can render the image nonexistent in the context of the webpage.
Another reason could be if you forget to include the ‘bg’ keyword before your gradient when using the ‘backgroundImage’ feature. This omission happens because Tailwind utilises utility classes for defining styles on an element. These can only give the desired output if all necessary keywords and parameters are included accurately.
One more common mistake you could make deals with the ‘tailwind.config.js’ file. The backgroundImage utility in Tailwind is not enabled by default. Adding `backgroundImage: [‘responsive’]` to the `variants` section of your Tailwind CSS configuration file is needed to ensure full functionality of the property.
Lastly, any other configuration settings in your project might also disrupt the proper function of backgroundImage. Therefore, taking out time to review and update would potentially solve the issue.
As Mark Zuckerberg rightly said, “The biggest risk is not taking any risk…in a world that’s changing really quickly, the only strategy that is guaranteed to fail is not taking risks.” It applies equally well to coding – venture to experiment and tweak your settings until you achieve the desirable results. When faced with scripting roadblocks like these, remember: persistence and experimentation are key.
Understanding the Basics of Tailwind CSS Background Images
Utilizing background images effectively in Tailwind CSS can be accomplished by having a clear understanding of the intricacies and functionality of the framework. However, confrontations with issues such as “Tailwind CSS backgroundImage not working” are common and may hinder this process. We will dissect the primary reasons for these difficulties and offer solutions to address them effectively.
Firstly, Tailwind CSS doesn’t include specific classes for background images. As opposed to other CSS properties like color or size that have designated utility classes in Tailwind, background image is one aspect where you would need to resort to custom CSS definitions.
Here’s an example of how you might set up a custom background image using Tailwind
:
html
<style>
.bg-custom {
background-image: url(‘/path/to/your/image.jpg’);
}
</style>
<div class=”bg-custom …
Please note in this context, the path to your image must be accurate. A common problem often resides in an invalid or incorrect URL, meaning the system cannot locate the required image. Thoroughly validate your URL pathway before implementing it into your project.
Secondly, your configuration file plays a significant role. If you’re utilizing PurgeCSS (which is included in Tailwind CSS), certain styles in your development could be getting purged during build time. To handle this, consider modifying your ‘tailwind.config.js’ file to ensure your custom styles remain untouched:
html
module.exports = {
content: [“./src/**/*.html”],
options: {
safelist: [‘bg-custom’], // Example class
},
theme: {…}
}
Your external StyleSheet might be overridden by Tailwind’s default style due to the Cascade in CSS if your styles do not have enough specificity. To prevent this, ensure Tailwind’s base styles are included first then your custom styles.
Although challenges met in employing Tailwind CSS for background images can be daunting, remember, as Pete Hunt, one of the early contributors to React says, “Any application that can be written in JavaScript, will eventually be written in JavaScript.” As developers, discovering problem-solving techniques and overcoming hurdles is an integral part of our journey!
For further information regarding Tailwind CSS backgroundImage not working issue, consult the [official Tailwind documentation](https://tailwindcss.com/docs/background-image).
Troubleshooting Common Problems with Tailwind CSS Background Image
Utilizing
background-image
with Tailwind CSS might present challenges due to different reasons. When you come across issues where the Tailwind CSS
backgroundImage
option doesn’t function as expected, there could be possible culprits causing such hiccups. In deciphering these issues, it’s essential to first understand how Tailwind uses utilities for managing background images and what potential problems might arise.
Error due to incorrect configuration in tailwind.config.js
Tailwind CSS relies heavily on “purge” setting in
tailwind.config.js
. If any classes are not utilized directly in your templates or follow standard conventions, they might get lost in production builds.
Fix:
Define your custom backgroundImage in your Tailwind CSS config, ensuring you adhere strictly to the required format.
module.exports = { theme: { extend: { backgroundImage: theme => ({ 'custom-name': "url('/path/to/your/image')", }) } } }
After making changes in your Tailwind CSS configuration file, restart your development server to reflect your changes.
Images not loading due to path issues
Incorrect paths to image files can result in your Tailwind CSS backgroundImage not functioning correctly.
Fix:
Ensure that the file pathway defined in
tailwind.config.js
corresponds to the exact location of your image file.
To illustrate;
module.exports = { theme: { extend: { backgroundImage: theme => ({ 'example-bg': "url('/public/images/background.png')", }) } } }
Paying particular attention to and confirming your image file pathway correctness can save you hours of debugging why Tailwind CSS backgroundImage is not working.
Misuse of directives
Misinterpretation or misspelling of Tailwind-specific directives might affect the output.
Fix:
Write correct Tailwind directives in your HTML file to use the backgroundImage utility properly.
For instance, The correct approach might look like this:
<div class="bg-custom-name"> </div>
These points are critical in troubleshooting your Tailwind CSS Background Image issues. They provide guidance allowing you take a more focused approach to solve your problem rather than beating about the bush. As Nicholas C. Zakas, a prominent programmer once said, “Understanding is key to good troubleshooting.” By understanding these keypoints, you’re well-equipped to beat Tailwind troubles regarding backgroundImage.
In-depth Analysis: Why Your Tailwind CSS Background Image Might Not Be Working
Tailwind CSS is a highly attractive utility-first CSS framework for rapidly building custom design solutions. It provides low-level utility classes that facilitate developers in crafting the design they need without leaving your HTML codes. However, while working with background images in Tailwind CSS, it’s not uncommon to encounter certain issues where the image simply won’t load or display as expected. This can be quite vexing, especially when delving deep into a development project.
This issue could stem from certain common causes. Let’s delve a deep dive and figure out potential reasons why:
1. **Incorrect File Path:** First and foremost, the typical culprit behind disappearing or non-displaying background images could be an incorrect file path. A missing image problem often boils down to relative or absolute URLs malfunctioning due to improper setup.
Double check whether the file path referenced in your URL is correct.
2. **Misuse of Tailwind’s Background Image Utilities:** Tailwind CSS has built-in background image utilities but these don’t parse URLs. They only parse keywords like
bg-none
,
bg-cover
,
bg-contain
, among others. Therefore, implementing the background via simply using the class like this would lead to errors:
. Tailwind CSS doesn’t have functional utilities for URLs so one must use inline styles for urls.
3. **PurgeCSS Removing Unused Styles:** If you are using PurgeCSS, which comes pre-configured with new Tailwind installations from v1.4 onwards, unknowingly, it might be purging your unused styles including your background-image styles. You should check your purge configuration to see if it’s removing the necessary CSS.
To circumvent this you may want to write as follows:
// tailwind.config.js module.exports = { purge: { content: ['./src/**/*.html'], options: { safelist: ['bg-url'] } }, theme: {...}, variants: {...}, plugins: [], }
This tells PurgeCSS to always keep the ‘bg-url’ selector and its associated styles.
Despite above-mentioned checks, if the background image is still refusing to cooperate, chances are that there might be unseen issues to address, such as third-party clashes or conflicts within other parts of your CSS code.
As HTML developer Patrick Lauke once quoted, “HTML is easy to learn, but almost impossible to master.” This perfectly encapsulates the intricate details and nuances that come into play while dealing with issues like those seen in background images of TailWind CSS.
Useful resources for additional scrutiny on the subject matter include the official [Tailwind Documentation](https://tailwindcss.com/docs), the reference materials available at [Mozilla Developer Network](https://developer.mozilla.org) and [Stack Overflow Discussions](https://stackoverflow.com/questions/tagged/tailwind-css).
By carefully diagnosing the reasons causing trouble with Tailwind CSS and rigorous auditing of your html codes, you should be able to overcome the hiccup of your background image not displaying correctly.
Practical Solutions to Fix Issues with Tailwind CSS Background Image
The primary function of Tailwind CSS is to streamline the process of writing a functional design directly in your markup, but sometimes, it might not work as desired. One common hiccup often occurs with the background image. You may find that even after setting up Tailwind CSS correctly and referencing everything properly, the background image might not display as expected. This situation could confuse anyone and if you’re in the same boat, keep reading; we will explore this constellation of problems together:
•
backgroundImage
utility variants is not activated: Tailwind comes with some utilities like `backgroundImage` directly from its plugin itself which can’t be activated using the tailwind.config.js file. Instead, they are found right inside the Tailwind CSS stylesheet which needs to activated by un-commenting the required code lines.
@tailwind base; @tailwind components; // ... @utilities;
• Incorrect File Path: One common problem web developers face while implementing a background image in Tailwind CSS is the exact location of an image file. Being very specific when mentioning the path for the image can solve this issue.
<div class="bg-[url('/path/to/your/image.jpg')]"> <p>Your Content</p> </div>
• Purge List in CSS is not Configured Correctly: When using subfolders or external file paths, it is important to include them in the purge list. It ensures your paths do not get purged during production load.
module.exports = { purge: ['./src/**/*.{js,ts,jsx,tsx}', './public/index.html'], theme: { extend: {}, }, variants: {}, plugins: [], }
Understanding Tailwind CSS’s core concepts can be quite advantageous here, allowing you to utilize its full capabilities without fault. For further reading, please refer to their official documentation.
As Elle Meredith, a web developer, blogger, speaker, and mentor beautifully emphasizes – “It is rewarding to know that what you build is influencing real life experiences of people—it’s a responsibility.” This sentiment perfectly sums up our approach to solving web development problems. Always remember that the end goal of any problem-solving endeavor in technology is about providing seamless user experiences. Every solution ultimately contributes to better products and happier end-users. The reward for us developers? It lies in the joy of creating something meaningful and being part of an ever-evolving digital world.Focusing on the topic: “Tailwind CSS Background Image Doesn’t Work For Me”, it is essential to dig deep into certain key aspects that could be affecting the functionality of the background image in your Tailwind CSS.
HTML elements possess numerous characteristics, and those applied by CSS can sometimes interfere with each other or not function as expected within particular frameworks like Tailwind. Let’s explore potential solutions to your issue.
Issues like this may stem from:
– Incorrect syntax usage in your style classes
– Forgotten installation or activation of specific plugins for image management
– Misconfiguration in customizing your tailwind.config.js file
For example, a commonly made error is forgetting to include the mandatory ‘bg’ prefix when defining background images. Have you checked whether your code resembles the following format:
...
Remember that Tailwind uses utility-first methodology. This means you should have control over individual styling components, giving you greater flexibility than with traditional CSS.
In cases where plugins are concerned, are you missing the ‘tailwindcss/plugin’ or have you checked its configuration?
You might want to cross-verify with the official [Tailwind CSS documentation](https://tailwindcss.com/docs/plugins#creating-a-plugin) to make sure.
During customization of the tailwind.config.js file, ensure careful handling. Even minor missteps could introduce bugs that affect features like background images.
Beth Cannon aptly mentioned once, “The most important property of good programming is transparence.” When dealing with any technology, including Tailwind CSS, proper understanding and transparency are required for effective implementation.
Should your challenges persist after these checks and adjustments, consider reaching out to the strong and knowledgeable [Tailwind CSS community](https://github.com/tailwindlabs/tailwindcss/discussions) for further assistance, tips, and tricks.