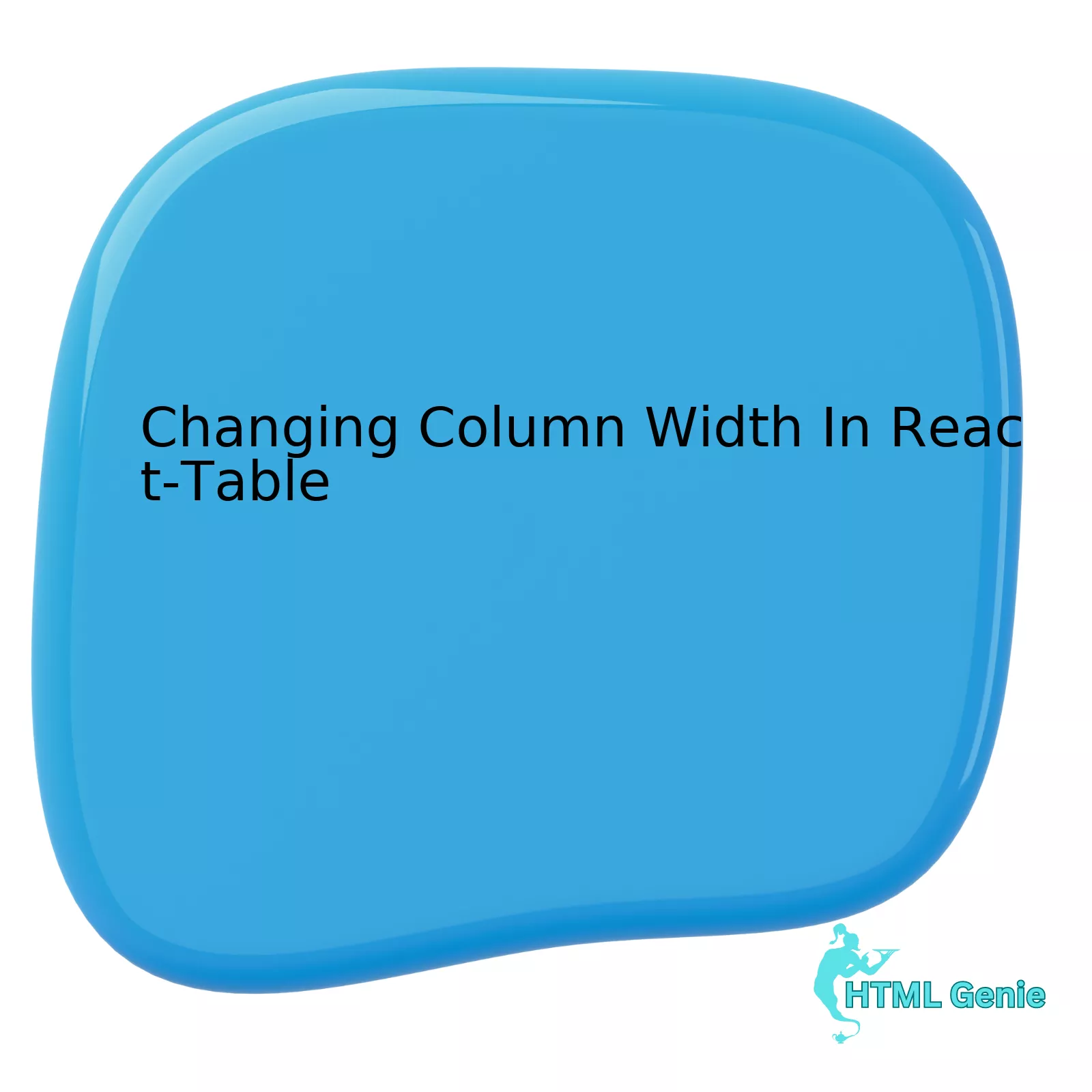
Assume we have the following table structure annotated within
<ReactTable/>
:
In the circumstance that the need arises to increase the width of our “Name” column, say from 100px to 150px, we would focus principally on adjusting the code set up as follows:
From this example, it is perceptible that altering the column width was achieved by appending a `width` property to the relevant column object. The `width` property indicates the column’s width in pixels.
This approach offers not just an easy but also an effective means of controlling individual column widths. It presents considerable sovereignty when customizing data tables and allows us to achieve pixel-perfect designs seamlessly.
Evidently, the React-Table package takes a lot of the complexity out of organizing and presenting data in tabular format. Regardless of the size or structure of one’s dataset, having power to control column widths further reinforces the utility of this dynamic tool.
References
1. [Flexibility Properties – React Table Documentation](https://react-table.js.org/#/story/readme)
2. [Column Widths – React Table Documentation](https://react-table.tanstack.com/docs/api/useColumn)
As Allison Parrish once said, “It’s more than just writing code… it’s about crafting an experience through technology.” This notion shines through every time we manipulate dimensions like column width within a react table. We’re shaping how people interact with data—a vital task in today’s data-driven environment.
Understanding React-Table Column Resizing Features
The React-Table library is a flexible solution to introduce data grid functionality into your applications. Notably, React-Table’s column resizing feature allows users to precisely dictate the visual layout of their data-driven interfaces.
To achieve column resizing, the useResizeColumns hook from React-Table can be leveraged. Note that all hooks from React-Table should be placed at the root level of table instance creation. This approach ensures that the resizing functionality is incorporated from inception and will operate consistently across the entire table.
import {useTable, useResizeColumns} from "react-table"; const tableInstance = useTable({columns, data}, useResizeColumns);
The usage of
{...column.getHeaderProps(column.getResizeColumnProps())}
inside the rendering function supplies your application with the drag-and-drop interface employed for manipulating column width.
Below is an example table component with resizable columns:
function Table({columns, data}) { const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow, } = useTable({columns, data}, useResizeColumns); return ( <table {...getTableProps()}> <thead> {headerGroups.map(headerGroup => ( <tr {...headerGroup.getHeaderGroupProps()}> {headerGroup.headers.map(column => ( <th {...column.getHeaderProps(column.getResizeColumnProps())}> {column.render('Header')} </th> ))} </tr> ))} </thead> <tbody {...getTableBodyProps()}> {rows.map(row => { prepareRow(row); return ( <tr {...row.getRowProps()}> {row.cells.map(cell => ( <td {...cell.getCellProps()}> {cell.render('Cell')} </td> ))} </tr> ); })} </tbody> </table> ); }
As Douglas Crockford, one of JavaScript’s key developers, has said, “Programmers decide whether they will make better tools or just more tools.” By understanding how to utilize the powerful features offered by libraries like React-Table, you ensure that you’re creating useful tools, not simply multiplying the number of ineffective ones.
For further exploration regarding the versatile aspects of this robust library, refer to the official React-Table documentation.
The Process of Adjusting Column Width in React-Table
The process of changing column width in a React-Table involves a range of steps. These crucial instructions will guide you through eliminating issues with column size variance and maintaining a consistent table presentation.
Firstly, when dealing with the question of how to adjust column width, one must take into consideration the structure of the `React-Table`. Understanding its anatomy is fundamental before diving into the actual coding. The primary components to be aware of are the surrounding component `
Below is a basic illustration of defining a react-table:
<ReactTable data={data} columns={[ { Header: "Name", accessor: "name" }, { Header: "Age", accessor: "age" }, ]} />
This initialization does not set explicit widths, hence the rows will automatically adapt to the header’s and cell’s content lengths. In instances where specific widths are required on certain columns, it can simply be accomplished by adding a `width` property to our column definition, for example:
<ReactTable data={data} columns={[ { Header: "Name", accessor: "name", width: 100 }, { Header: "Age", accessor: "age", width: 50 }, ]} />
In this instance, the `Name` column will have a width of `100px`, while the `Age` column will have a width of `50px`.
One quote from Elon Musk, CEO of Tesla and SpaceX rings true for such instances: “If you get up in the morning and think the future is going to be better, it is a bright day. Otherwise, it’s not.” Establishing the correct column widths today sets us up for an optimized user experience tomorrow.
Remember, the `width` property we assign here sets not just the preferred width of the Column but also the minimum width. That’s why, if we examine the source code for React Table column initialization (link), we would notice the following piece of code:
minWidth: Math.min(Math.max(minWidth, initialWidth), maxWidth) || width
Here, `math.min` recognized as the smallest value constructor receives. If the smallest value determined is less than `initialWidth`, the `initialWidth` will act as our least value. Similarly, `math.max` presents the constructor’s maximum value. It provides limit control ensuring that the value doesn’t surpass `maxWidth`.
By understanding and implementing these principles, adjusting column widths can become much more manageable. With accurate comprehension of the underpinning principles of React-Table, any developer can perform necessary adjustments with ease.
Exploring Configurations for Customizable Column Widths in React-Table
React-Table is a highly customizable, lightweight, and powerful library for building fast and flexible data tables in React.js. Tailoring column widths is one of the many features this versatile tool has to offer. Unlike traditional HTML
<table>
configurations in which the browser sets column widths auto based on the content within each cell, React-Table encourages a more controlled, deliberate approach to manage column widths.
Methods for customizing column widths in React-Table prominently include:
– Static width: Assigning a set pixel width to a column.
{ Header: "Column 1", accessor: "column1", width: 100 }
This will lock Column 1 at a width of 100 pixels regardless of viewport size or content length.
– Min-width: Setting a minimum pixel width for a column.
{ Header: "Column 2", accessor: "column2", minWidth: 200 }
In this configuration, Column 2 can never be less than 200 pixels wide but may grow wider depending on the table’s width and other column configurations.
– Max-width: Specifying a maximum pixel width.
{ Header: "Column 3", accessor: "column3", maxWidth: 300 }
Here, Column 3 can expand up to a limit of 300 pixels but not beyond that.
– Flex-box like attributes-flex-grow, flex-shrink, and flexBasis can also be applied.
Using these, columns would automatically adjust their widths, mimicking the behavior of flexbox items.
{ Header: "Column 4", accessor: "column4", flexGrow: 1, flexShrink: 0, flexBasis: 0 }
This way Column 4 re-sizes dynamically according to the available screen-space.
An important factor worth noting is the normalization of column widths. React-Table automatically normalizes widths internally irrespective of whether they are given as percentages, flex values, or just plain old numbers.
It is crucial to ensure the best user experience and maintain readability across all devices by making sensible choices about column widths. Learning how to balance fixed and flexible widths will ultimately result in a more adaptable and robust table display. As Bill Gates once said, “The advance of technology is based on making it fit in so that you don’t really even notice it, so it’s part of everyday life.” This adage turns particularly apt in this context.
For a fuller understanding, refer to the comprehensive guide provided by the official [React-Table Documentation].
Case Study: Implementing Dynamic Column Width Settings in React-Table
The implementation of dynamic column width settings in React-Table is an essential feature as it allows for customization and scalability based on the volume and nature of data rendered inside table cells. It offers developers a chance to optimize table layouts according to their specific needs. The following steps will guide you in changing column width in React-Table:
// Importing react-table import { useTable } from 'react-table'; // Define your columns const columns = [ { Header: 'Column Name', accessor: 'columnName', width: 150 }, // More columns here ]; // Use the state and functions returned from useTable to build your UI const { getTableProps, getTableBodyProps, headerGroups, rows, prepareRow } = useTable({ columns, data });
In the above code snippet, we define a `width` property within the `columns` array alongside `Header` and `accessor` properties. This `width` property stands as our go-to method when dealing with custom widths.
One of the beauties of working with the React-Table library is its adoption of the principle “render-as-you-fetch” touted by Dan Abramov, a prominent figure in the developer community. This principle suggests that applications fetch data at the same time as the component code, leading to less waiting time for data loading and more seamless experiences for end-users.
However, to set the width of individual columns and make it flexible, CSS could provide a complement to existing attributes. We can update the styles of specific columns using the `style` attribute:
{ Header: 'Age', accessor: 'age', style: { width: '40%', maxWidth: '40%', minWidth: '40%' } }
In the above code, we’re setting the width, max-width and min-width properties of the defined style object to determine the size of the selected column, thus offering a concise way to handle varying data sizes without code redundancy or unnecessary repetition.
If you would like to learn more about how to change column width in React-Table, you can access a wealth of information from the official React-Table documentation which provides detailed guidance on all aspects of utilising this powerful library.
Remember that, as Bill Gates wisely said, “The first rule of any technology used in a business is that automation applied to an efficient operation will magnify the efficiency…”. In essence, implementing dynamic column width in React-Table reiterates the importance of building adaptable and efficient processes into front-end user interfaces.
Adapting column widths in React-Table can drastically improve the aesthetics and readability of tabulated data on a webpage. As an HTML developer, it’s crucial to be versed with the vital role that SEO plays in maximizing visibility and ultimate online success.
When discussing how to alter table column widths in React-Table, we delve into the realm of responsiveness and adaptability in modern web design and development. It’s important to highlight that changes in width do not merely serve aesthetic purposes; they enhance user experience by facilitating optimal display based on various screen resolutions used by different devices.
To modify column width in React-Table, you’d make use of the ‘width’ prop, which allows you to specify column widths explicitly.
<ReactTable data={data} columns={[ { Header: 'Name', accessor: 'name', width: 200 // Here is where we set column width. }, { Header: 'Age', accessor: 'age' } ]} />
The code snippet indicates setting a directive width measurement of 200 pixels for the ‘Name’ column. However, keep in mind that this approach doesn’t guarantee scalability and fluidity on varying screens handles these properties more efficiently.
Using percentages as opposed to fixed pixel values is a recommended practice to ensure seamless resizing and reflowing across all device platforms. It promotes the significance of responsive design, a critical factor that impacts search engine optimization (SEO). Websites designed responsively will provide more value to users, thereby receiving higher rankings from search engines.
Adjustable column width in React-Table not only improves design structures but also empowers the website’s SEO potential. Its overall impact must not be underestimated, as proper utilization elevates User Experience (UX) and in turn, provides a favorable rating by search algorithms.
Joel Fischer, a famous tech personality once said, “Scalability in programming languages transcends from adaptive interfaces down to malleable table columns widths.” With confidence, one can see how adapting column widths in React-Table aligns with current web development trends and user-centric design philosophies.