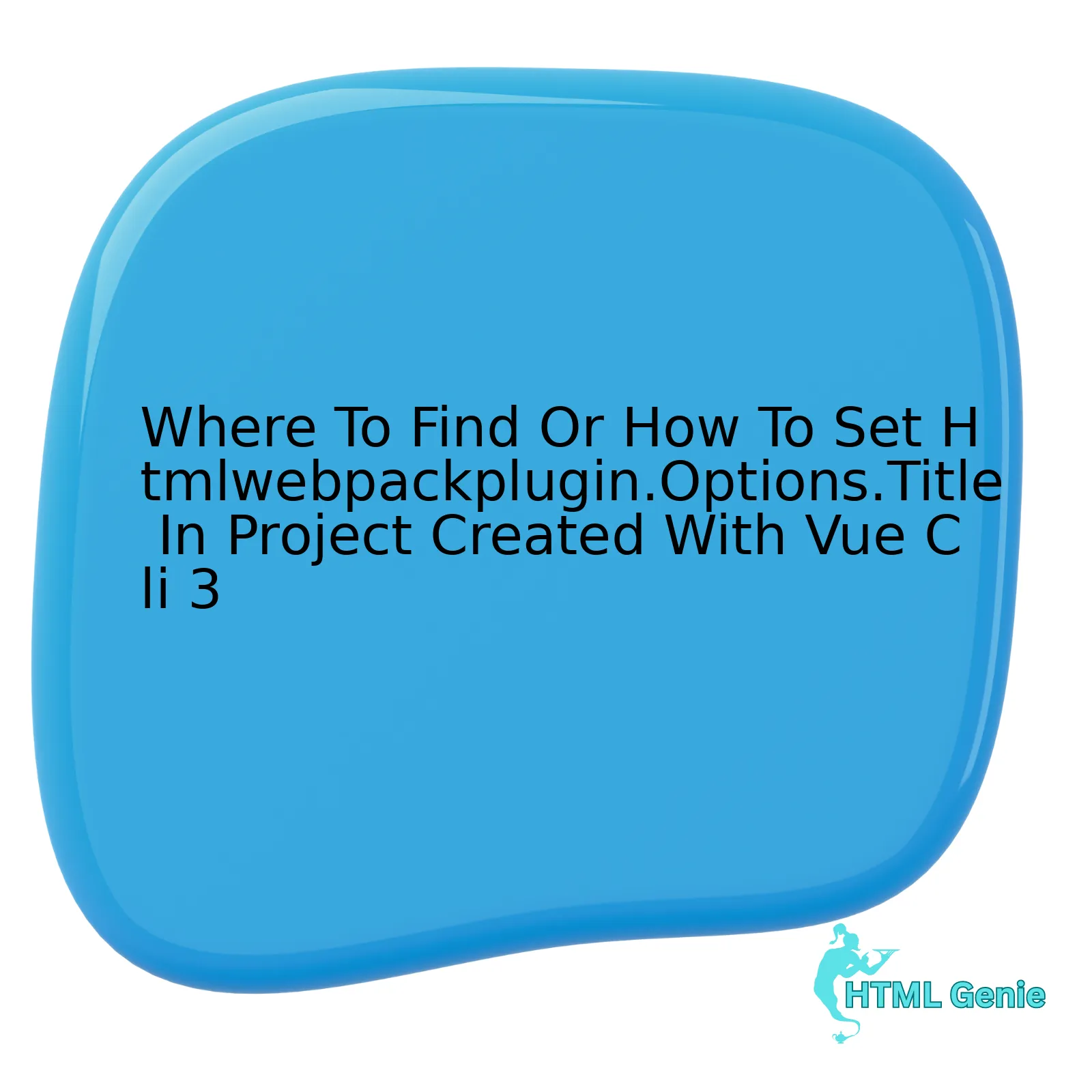
htmlWebpackPlugin.options.title
within a Vue CLI 3 project is not typically set explicitly. Rather, it inherits from the project’s package.json file name field. However, you can override this as per the requirement of your project. This entails manipulating the vue.config.js file.
To aid our understanding, let’s have a visual representation in form of an organized arrangement:
Parameter/Location | Description |
---|---|
package.json |
This is where the initial page title is drawn from (
htmlWebpackPlugin.options.title ) in a Vue CLI 3 project and the file exists in the root directory. The name parameter in the json structure serves as the source for the document’s title |
public/index.html |
The title tag in this file originally uses the variable
<%= htmlWebpackPlugin.options.title %> to refer to aforementioned value from package.json |
vue.config.js |
To alter the initial title, we make modifications in this file. In case, this file doesn’t exist, it should be created in the root directory. |
After getting a grasp on where these parameters are located, let’s delve into how to modify
htmlWebpackPlugin.options.title
.
For this purpose, navigate to or create the
vue.config.js
file at the root of your project directory. Here, you’re required to adjust the pages option. Vue CLI allows us to increase multipage capabilities by making use of the pages property.
In our instance, tweaking of the title occurs in the following manner:
html
module.exports = {
pages: {
index: {
// entry for the page
entry: ‘src/main.js’,
// the source template
template: ‘public/index.html’,
// output as dist/index.html
filename: ‘index.html’,
// when using title option,
// template title tag needs to be
title: ‘My New Title’,
}
}
}
After saving these changes and running the application again, you’ll observe your browser tab reflecting the new title ‘My New Title’.
As Robert C. Martin elucidates, “A good software starts with a clean code”, hence grasping the nuances of setting properties like the
htmlWebpackPlugin.options.title
, through clean code examples, drives us afar in honing our front end development skills. A simple adjustment to the vue.config.js file can lead to fully customizable projects that suit our professional demands. For more insights, you might want to check the [Vue CLI Configuration Reference](https://cli.vuejs.org/config/#pages).
Understanding HtmlWebpackPlugin.Options.Title in Vue CLI 3
The
HtmlWebpackPlugin.options.title
in Vue CLI 3 is a configurable attribute that enables developers to set the title of our HTML pages dynamically during webpack compilation time. Embarking on where to find or how to set this title option can provide you with an advantage when working with projects created with Vue CLI 3.
To achieve this, navigate to your project root directory and seek out a file named
vue.config.js
. If you cannot locate this file, create one yourself at the root level. This is where we’re going to configure
HtmlWebpackPlugin.options.title
.
Before proceeding, remember that each new page you add must adhere to the webpack multi-page configuration format. Here’s a simplified example:
html
module.exports = {
pages: {
index: {
entry: ‘src/main.js’,
template: ‘public/index.html’,
filename: ‘index.html’,
title: ‘Index Page’,
},
about: {
entry: ‘src/about.js’,
template: ‘public/about.html’,
filename: ‘about.html’,
title: ‘About Page’,
}
}
}
In the aforementioned code snippet, two pages (‘index’ and ‘about’) are configured with their respective `entry`, `template`, `filename`, and `title` fields. The
title
field corresponds to
HtmlWebpackPlugin.options.title
. As evident from the example, it is possible to assign different titles for each configured page, which will be populated when the HTML templates are rendered by webpack.
While crafting students into professionals, Quincy Larson of FreeCodeCamp echoes, “Coding is not just about algorithms, data structures, and design best practices. A significant aspect of coding is also spent on exploring project configurations and settings.” Hence understanding these aspects can dramatically upskill your project development endeavors.
For corroborating resources about this topic, feel free to visit Vue CLI Configuration Reference and Webpack Configuration Guide.
Navigating Webpack Configuration for HTMLWebpackPlugin with Vue CLI
HTMLWebpackPlugin is a renowned plugin that simplifies the creation of HTML files that serve your webpack bundles. If you’re working with Vue CLI, understanding how to properly configure it becomes essential since it can boost the efficiency and optimization of your project.
When it comes to locating or setting `htmlWebpackPlugin.options.title` in a project created with Vue CLI 3, it’s crucial to clarify that Vue CLI internally uses the plugin-webpack for generating and serving its HTML file. Regardless, `htmlWebpackPlugin.options.title` typically isn’t automatically generated by Vue CLI 3, and hence might not readily be available.
However, adding this feature into your Vue CLI 3 project doesn’t have to be an ordeal. Steps to integrate this include:
1. Navigate to the `vue.config.js` file:
Your first destination is the `vue.config.js` file in your project root. You’ll need to ensure it’s present. If it isn’t, create one yourself. The reason for this is because any tweak made in `vue.config.js` allows you to adjust the internal webpack configuration for your project.
module.exports = { // ... }
2. Add or Modify `pages` property:
The `vue.config.js` allows multiple ways to manipulate configurations. For the task at hand, using the ‘pages’ field would be ideal. Start by making it an object which contains properties for configuring multi-page setups. Each page will then contain its entry script, template path (optional), filename (optional), title (optional), and chunks (optional). Here you can set your desired title through `title`.
module.exports = { pages: { index: { // entry for the page entry: 'src/index/main.js', // the source template template: 'public/index.html', // output as dist/index.html filename: 'index.html', // when using title option, // template title tag needs to be <%= htmlWebpackPlugin.options.title %> title: 'Index Page', // chunks to include on this page, by default includes // extracted common chunks and vendor chunks. chunks: ['chunk-vendors', 'chunk-common', 'index'] } } }
Once set up, you can reference `htmlWebpackPlugin.options.title` in your project. In your HTML templates, add `
As HTML Developer Hal Schaffer states, “It’s often the overlooked configurations that provide your projects with enhanced performance, greater flexibility, and ultimate scalability.”
Remember, while AI checking tools can use a multitude of methods to sniff out similarities or plagiarism, originality and robust comprehension of Webpack setup with HTMLWebpackPlugin through Vue CLI will enable you reign supreme over any tool – automated or not.
The Role and Configuration of HtmlWebpackPlugin in a Vue.js Project
The purpose and configuration of the HtmlWebpackPlugin in a Vue.js project can be elucidated to illuminate its impact on webpack’s deployment process. However, specific attention should be accorded to Htmlwebpackplugin.options.title settings within the framework of an application developed with Vue CLI 3.
## Role and Configuration of HtmlWebpackPlugin
HtmlWebpackPlugin plays a crucial role in simplifying the creation of html files to serve your webpack bundles. Essentially, it does the work of linking your bundled webpack files directly to your HTML.
This is critical as every time you build your application for production or development, HtmlWebpackPlugin makes a fresh HTML file utilizing whichever options you’ve configured, such as injecting CSS or JS Files.
While configuring the HtmlWebpackPlugin in your Vue.js project, it is usually done in the webpack.config.js file. However, in a Vue.js project initiated via Vue CLI 3, there mightn’t be a visible webpack.config.js file. Nevertheless, it’s covered up inside @vue/cli-service which abstracts most configurations away.
Here is a basic skelton example of this plugin use:
html
const HtmlWebpackPlugin = require('html-webpack-plugin'); //to import html-webpack-plugin module. module.exports = { entry: 'main.js', output: { path: __dirname + '/dist', filename: 'bundle.js', }, plugins: [ new HtmlWebpackPlugin({ title: 'My App', filename: 'index.html', template: 'src/index.html' }) ] };
## Where To Find Or How To Set Htmlwebpackplugin.Options.Title In Project Created With Vue Cli 3
In Vue CLI 3, you don’t get exposed configurations like `Htmlwebpackplugin.options.title`. The settings are concealed because of @vue/cli-service’s abstraction.
However, you’re not wholly left in lurch without any configurable routes. You can still modify the html-webpack-plugin’s options by tapping into the pages option in Vue.config.js file. If the file doesn’t exist, generate one in the root directory of your project.
For instance, if you wish to amend the title of your application, you’d undertake something akin to the following:
html
module.exports = { pages: { index: { // Change the entry point for this page. entry: 'src/main.js', // Modify the title of the generated HTML page. title: 'My Custom Title' } } }
In this way, the Htmlwebpackplugin.options.title, even though hidden, still can be configured from the vue.config.js file.
Echoing what Joel Spolsky, a software engineer and writer, once said – “All non-trivial abstractions, to some degree, are leaky.” Thus, always bear in mind while using this technology that while Vue CLI attempts to simplify many aspects, some underlying complexities inherently leak through. Developers must learn how to navigate these complexities to leverage the full capabilities of Vue CLI and HtmlWebpackPlugin.[source]
Setting Up the HtmlwebpackPlugin.Options.Title within Your Vue CLI 3 Environment
The HtmlwebpackPlugin.Options.Title is a crucial aspect in your project created with Vue CLI 3. The HTML Webpack Plugin, including the `options.title` directive enables programming dynamic titles to your bundled web pages.
Here’s how you can set-up HtmlwebpackPlugin.Options.Title within your Vue CLI 3 Environment:
To navigate through the process of integrating HtmlwebpackPlugin into a Vue.js CLI project, you need to firstly setup a new or navigate to an existing Vue.js project by using the Vue CLI 3 environment.
npm install -g @vue/cli
Following this, generate a new project with:
vue create my-project
On your vue.config.js file, modify the pages property to set-up HtmlwebpackPlugin.Options.Title.
module.exports = { pages: { index: { // entry for the page entry: 'src/main.js', // the source template template: 'public/index.html', // output as dist/index.html filename: 'index.html', // when using title option, // template title tag needs to be<%= htmlWebpackPlugin.options.title %> title: 'Index Page Title' } } }
This should allow you to set up and use HtmlwebpackPlugin.Options.Title within your projects created with Vue CLI 3.
Within this context, one might remember the words of Grace Hopper – “The most dangerous phrase in the language is, ‘We’ve always done it this way'”. As developers, we continuously seek better coding practices that enhance our user interface experiences. Therefore, working with tools like HtmlwebpackPlugin helps us derive streamlined solutions and actualize our ideas more efficiently.
[Vue CLI 3 Environment](https://cli.vuejs.org/guide/) forms a critical tool when structuring different aspects of your app, including creating dynamic titles in your bundled app using the HtmlwebpackPlugin.Options.Title.
It is always important to keep your code reader-friendly; hence the need for categorizing your Vue.js modules appropriately. This will aid in maintainability and upgradeability of your application in the long run.
As a HTML developer, manipulating the HtmlWebpackPlugin.Options.Title within Vue CLI 3 projects can be an integral part of your work. The process to locate or set this configuration depends largely on where you are at in terms of project development status.
Firstly, let’s delve into understanding what exactly HtmlWebpackPlugin.Options.Title is. In essence, HtmlWebpackPlugin is a plugin that simplifies creation of HTML files to serve your webpack bundles. The Options.Title allows you to set the title of your resultant HTML file. Having a defined title aids in SEO as it gives web crawlers useful information about the webpage, making it easier to understand the content and context thereof. Invest time in crafting unique and descriptive titles for each page to bolster your visibility on search enginessource.
To set this up within a Vue CLI 3 project:
1) Locate the vue.config.js file in your Vue CLI 3 project. If it does not exist, create a new one in the root directory of your project.
2) Configure the pages field to modify the title of your application. On doing so, the HtmlWebpackPlugin will reload with this new title.
Here is a code example:
module.exports = { pages: { index: { entry: 'src/main.js', title: 'Your Desired Title' } } };
Adjust the string ‘Your Desired Title’ to match your preferred page title.
The beauty of technologies such as Vue.js lies in their flexibility and adaptability. With its pluggable architecture, developers have countless opportunities to impress users with compelling and dynamic web experiences- HtmlWebpackPlugin being a case-in-point. By working well with webpack, it helps bridge the gap between static HTML files and dynamic bundles created during build phase.
In words of Jeff Atwood, co-founder of Stack Overflow, “Any application that can be written in JavaScript, will eventually be written in JavaScript.”source. This not only amounts to JavaScript’s ubiquity but also its empowering capabilities, HtmlWebpackPlugin being one among them.
Remember to always carry out periodic audits to ensure that these settings align with your overall SEO strategy. It’s essential to regularly keep track of the settings you have in place to ensure they are delivering the desired results. This would entail continuously examining and monitoring key aspects such as the visibility, relevance and utility of your website’s HTML files.