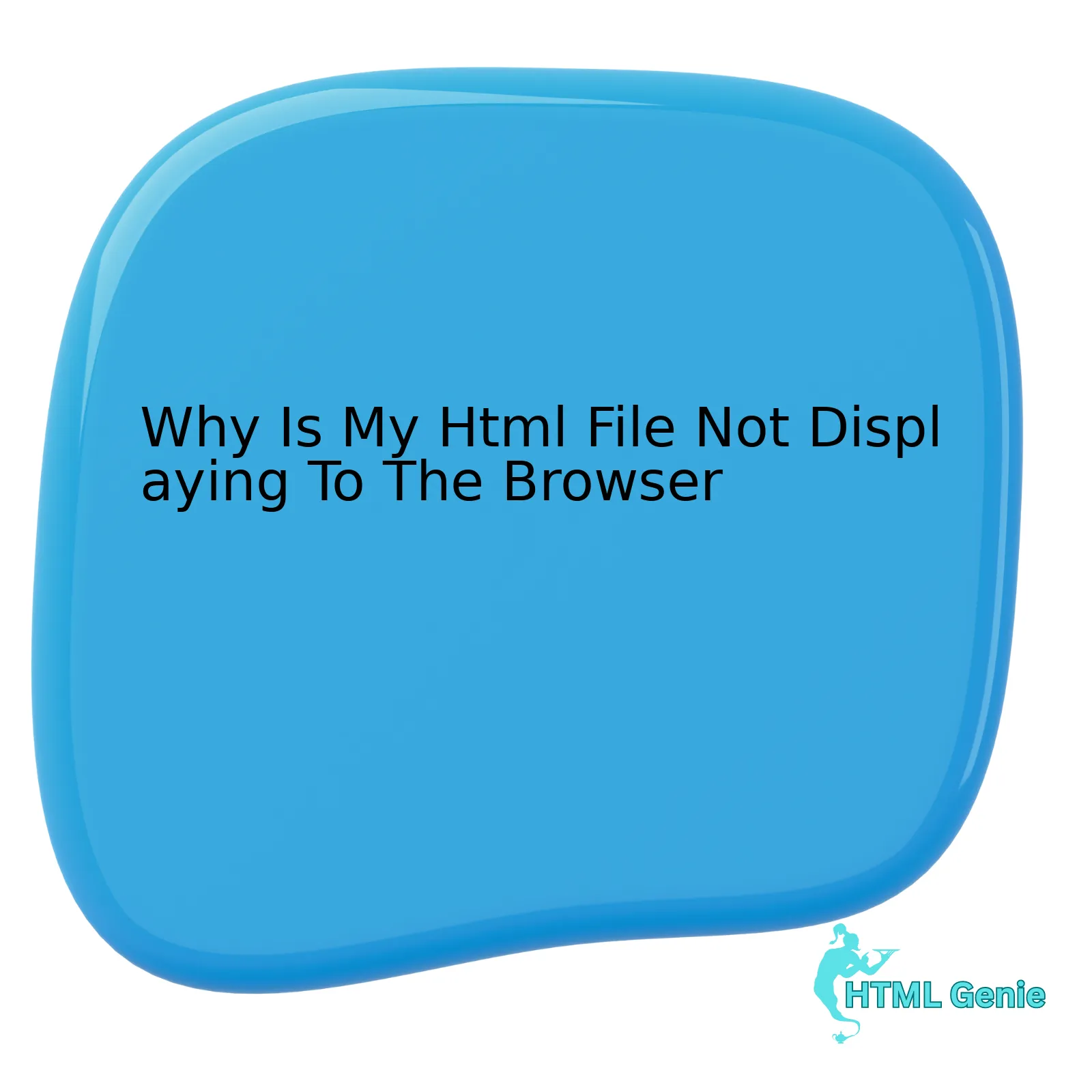
Possible Cause | Solution |
---|---|
Incorrect File Extension | Ensure the file saved as ‘.html’ or ‘.htm’ |
Path Issue | Check the absolute/relative path address for correctness |
Code Error | Validate your HTML code for missing tags or syntax errors |
Browser Cache | Clear the browser cache and try reloading the page |
Server Misconfiguration | Contact your hosting provider or check server settings |
Firstly, the most common mistake involves saving files without the correct extension. An HTML document should be saved with ‘.html’ or ‘.htm’. If your file is saved with a different extension, the browser won’t recognize it as an HTML file.
Secondly, the issue may arise from incorrect paths to your HTML file. If you are trying to access the HTML file locally, assure that you have specified the correct relative or absolute path.
Thirdly, erroneous HTML coding can prevent successful file rendering. Missed closing tags, extra quotes, or other syntax mistakes can create issues. Use an online validator (The W3C Markup Validation Service) to identify these errors in your HTML code.
Another factor causing the problem could be your web browser’s cache. As Tim Berners-Lee, inventor of the World Wide Web, states “The Web does not just enable more efficient discovery of information, but it also enables errors to propagate at the speed of light.” Old or corrupted cache data can obstruct the current form of your HTML document. Clearing your browser’s cache may resolve this issue.
Lastly, when hosting your site, a misconfigured server could be causing your HTML not to display. This includes incorrect file permissions, faults in the .htaccess file, or issues with the configuration file of your server.
By breaking down each possible cause and corresponding solution, we can systematically diagnose and fix the issue stopping your HTML file from displaying on your browser.
Understanding the Basics of HTML Browser Display Issues
HTML is the foundational markup language that powers the visible web. If you’re experiencing issues with your HTML file not displaying correctly in a browser, there could be several key reasons at work:
Embedding a Table for diagnosing common issues:
Potential Issues | Description | Common Solutions |
---|---|---|
File Save Format | If the file isn’t saved as an .html or .htm format, it may not be readable by browsers | Always make sure to save files with a proper extension such as filename.html |
Incorrect Document Structure | A missing or incorrectly used tag can cause display issues | Ensure the basic structure of
<html>, <head>, <title>, and <body> tags are properly nested |
Misuse of Tags | Writing incorrect syntax or using inappropriate attribute values can lead to non-rendering elements | Familiarize yourself with standard HTML tags and their usage. Tools like [W3Schools](https://www.w3schools.com/) can be very helpful. |
Additionally, here’s a snippet from Linus Torvalds, the creator of Linux on the importance of attention to detail in coding, “Bad programmers worry about the code. Good programmers worry about data structures and their relationships.”
Understandably, navigating through browser display problems can seem daunting, especially for beginners in HTML development. However, a carefully staged approach to troubleshooting often yields results. Adopting best practices when writing HTML, learning from mistakes, and constantly enhancing one’s understanding of how to construct robust, efficient websites will prevent many future browser display issues.
When diving deeper into this topic, reference well-documented resources like Mozilla Developer Network and StackOverflow threads. It’s crucial to the discipline’s improvement to stay informed about digital technology trends and acknowledge the reality of continual learning in the profession. This approach prepares you to address more complex browser compatibility issues in the future.
Resolving Common Syntax Errors in HTML
Often times, HTML files fail to display correctly on a browser due to various common syntax errors in the code. Let’s consider some of these along with tips and techniques to resolve them.
1. Incorrect or Missing DOCTYPE declaration: The DOCTYPE declaration is crucial within an HTML document as it helps the browser identify the version of HTML being used, thus leading to appropriate rendering. If your website is not displaying correctly, ensure that the initial declaration follows the format
<!DOCTYPE html>
, signifying HTML5.
2. Ill-structured tags: Each tag within HTML must adhere to a certain structure for valid syntax. For instance, every opening tag should be corresponded with a closing tag unless it is inherently self-closing (e.g.,
<img>
or
<br>
) . Always validate your tags begin with
<tagname>
and end with
</tagname>
.
3. Unescaped Characters: Certain characters require special treatment in HTML, particularly when they are part of the text content. If you’re noticing oddities in your displayed content, look at whether all special characters have been appropriately escaped. For example, if you require an ampersand “&”, utilize the escape sequence
&
instead.
4. Nesting Errors: Proper nesting involves encapsulation of child elements within parent elements. Any incorrect order can result in a misleading document tree which could throw off the browser’s rendering process. The formula goes as follows
<parent><child></child></parent>
.
To illustrate, consider a table structure:
<table> <tr> <td>Content 1a</td> <td>Content 1b</td> </tr> <tr> <td>Content 2a</td> </td>Content 2b</td> </tr> </table>
Each
<td>
(or cell) is nested within a
<tr>
(or row), and rows are nested within the
<table>
element, forming a hierarchical and orderly structure.
For debugging HTML and locating these syntax errors, tools like [W3C Markup Validation Service](https://validator.w3.org/) can be invaluable. Simply inputting your URL or uploading your file will present you with a list of warnings and errors observed in your code.
As Bjarne Stroustrup, the creator of C++, once said, “Proof by analogy is fraud”. This applies to web development as well – just because one piece of code worked in one circumstance doesn’t mean it’ll work in another. Therefore, always take the time to understand your syntax, debug your issues logically, and reassess from different perspectives as necessary.
Navigating File Path Problems with HTML Files
When developing a website using HTML, encountering file path issues that prevent your HTML file from displaying correctly in the browser is a common problem. This issue typically arises due to incorrect directory paths whilst linking to your CSS files, images or scripts in your HTML document. When these paths are not precisely defined, the browser will fail to locate the referenced files, causing your page not to display as expected.
Understanding File Paths
There are two types of file path references that you could use:
• Absolute: An absolute path refers to the complete details needed to locate a file or directory, starting from the root element and ending with the other subdirectories. For example, /home/example/document.html denotes an absolute path.
• Relative: Conversely, relative paths are based on the current directory where the file resides. For instance, if our HTML file is within the same directory as our CSS file, then the path would look like: <link rel=”stylesheet” type=”text/css” href=”style.css”>.
To ensure proper HTML rendering across all browsers:
1. Mitigate Syntax-related Issues: Check for any typographical errors while writing the file paths. A single typo can result in the entire file path being invalid. As such, the corresponding resources like images or styles won’t load.
2. Normalize Your Directory Structure: Adopt a clear directory structure that makes it easy to reference your files. Organized folder structures with descriptive names ease maintainability and accessibility.
3. Relative versus Absolute Paths: If your file structure isn’t overly complicated, using relative paths may be more beneficial. They are generally easier to understand at a glance and reduce chances of displaying incorrectly due to server differences.
4. Resolve Compatibility Problems: Also, remember different browsers interpret file paths differently. Therefore, ensure the paths used are compatible across different browsers. Cross-browser compatibility increases website traffic and reduces bounce rate.
5. Avoid Special Characters: Avoid naming your file paths using special characters. While some systems can handle this without problems, others may encounter issues which can lead to non-display of an HTML file in a browser.
As Bill Gates famously stated, “Measuring programming progress by lines of code is like measuring aircraft building progress by weight.” In essence, focusing on the accuracy of your code, including file paths, will have a greater impact on the quality of your HTML document than simply writing more lines of code.
Below is a simple HTML file structure example emphasizing directory structure for better understanding:
root_directory ├── index.html └── css ├── main.css └── js ├── script.js └── img ├── logo.png
For the above structure, an accurate file path in your index.html file would be:
<link rel="stylesheet" typeof="text/css" href="css/main.css"> <script src="js/script.js"></script> <img src="img/logo.png" alt="Company Logo">
The relative file paths are used here because the referenced files (CSS, JS, and the image) exist within the same directory as our HTML file.
To sum up, correct interpretation of file paths is crucial to ensure the proper displaying of your HTML content. Military-grade precision when creating your links and references can make or break your website’s functionality. By following these guidance points, utilizing the pertinent links and cross-referenced information provided, you should experience fewer to nil file path problems leading to maximized productivity and an optimal user experience.
Role of Web Browsers in Rendering HTML Files
When it comes to the topic of why your HTML file might not be displaying correctly in a web browser, it’s important to understand the role that web browsers play in rendering these files.
Web browsers are technically interpreters. They read and interpret HTML (and other languages like CSS and JavaScript) and present them visually to users on their device [source]. This process, known as rendering, is essential for viewing web pages as intended by their developers.
However, certain issues can occur during the HTML to visual content translation process which could result in your HTML file not displaying correctly. Here’s what needs to be kept in mind:
Browser Compatibility:
Different web browsers interpret HTML code in different ways. Internet Explorer, Chrome, or Firefox may display your HTML file differently due to subtle differences in how each interprets these instructions [source]. Make sure your HTML, CSS, and JavaScript code are compatible with multiple browsers.
Syntax Errors:
HTML uses specific syntax rules that must be followed for your code to work correctly. Misspelled tags, missing closing tags, or incorrectly nested tags can all cause a web browser to fail when attempting to render your file properly [source] .
Poorly Formed URL(s):
A common reason for an HTML file not displaying in a browser is a miswritten URL. Ensure you have included the correct protocol (http or https), host, and complete path to your file [source].
File Encoding:
Your file’s encoding might be incompatible with the browser. Windows generally use “ISO-8859-1”, while Linux and Mac OS use “UTF-8”. As such, if your HTML file was created in Linux or Mac OS and opened in a Windows-based browser, it may not render correctly unless its meta charset corresponds to the operating system’s encoding.
As Jeff Atwood, co-founder of Stack Overflow, once said, “Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” So, ensure to write clean, clear HTML code. This way, not only will other coders understand your code – but more importantly, so will web browsers.Diving into the crux of the matter, HTML is inherently a tool leveraged to structure and present content on the web. But what happens when your HTML file refuses to display in the browser? This underlying problem can be attributed to multiple factors.
The absences of a proper DOCTYPE declaration:
One reason might be missing or incorrect Document Type Declaration (doctype). Doctype indicates the HTML version to the browser and looks somethings like this:
<!DOCTYPE html>
Absence of correct doctype can lead to the browser rendering the page in ‘quirks mode’ which can cause various display problems.
Syntax errors in your HTML code:
HTML is unforgiving when it comes to syntax errors. Even the smallest mistake such as an unclosed tag or missing quotation mark around attribute values can result in the HTML file failing to load in the browser.
<h1>Hello World</h1>
In the above example, ensure all tags are properly opened and closed.
Incorrect path to the HTML file:
Sometimes, it’s not about the HTML itself but its location. If the browser doesn’t know where to find your HTML file, it won’t be able to display it. Ensure that you’re using the right path, whether it’s absolute or relative.
Your file is not saved as .html:
This may seem elementary, but double-check that your file has the correct .html extension. Without it, the browser won’t recognize this as HTML.
While these points encapsulate the primary causes for an HTML file not displaying in a browser, there can inevitably be additional particular reasons based on a case-by-case basis. As Bill Gates once said, “I choose a lazy person to do a hard job because a lazy person will find an easy way to do it”. Similarly, consistently checking and eliminating the possibilities can root out the problem faster: ensure proper syntax, correct paths and appropriate encoding while saving your efforts from repetitive amends.
Do explore the Mozilla HTML Basics Guide for further understanding of HTML behaviours on browsers. So, keep experimenting, learning by doing and soon the clouds of confusion will clear away!